Summary
Extracts LAS files that overlay the clip features or extent.
Illustration
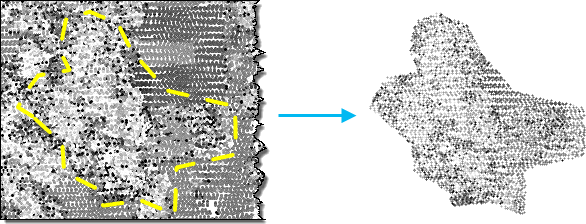
Usage
-
When a LAS dataset is specified as input, all the data points in the LAS files it references will be processed. A subset of lidar data can also be selected by its classification codes, classification flags, and return values by applying the desired LAS point filters through a LAS dataset layer. Filters can be defined through the layer properties dialog or the Make LAS Dataset Layer tool.
Consider using this tool to extract a subset of lidar data captured in the source lidar files. For example, if you only need to work in an area defined by a polygon boundary, you can extract the LAS file using the polygon as the boundary feature.
To re-project LAS files, specify an Output Coordinate System in the Environment Settings. If the LAS files being processed do not have a spatial reference defined, the extracted LAS files will inherit the specified projection.
If the extraction extent is defined along with an extraction boundary, the intersection of both will be used to define the coverage of the extracted LAS files.
The Rearrange Points parameter, if checked (rearrange_points = 'REARRANGE_POINTS' in Python), orders the point records into spatial clusters that are optimized for reading the files. This optimization dramatically enhances display throughout the ArcGIS platform. Rearranging points will add some processing time to complete. Since rearranging the order of points for improved data access is a fundamental improvement to the data, this parameter is checked by default.
Syntax
ExtractLas_3d (in_las_dataset, target_folder, {extent}, {boundary}, {process_entire_files}, {name_suffix}, {remove_VLR}, {rearrange_points}, {compute_stats}, {out_las_dataset})
Parameter | Explanation | Data Type |
in_las_dataset | The LAS dataset to process. | LAS Dataset Layer |
target_folder | The folder that LAS files will be written out to. Each output file will have the same LAS file version and point record format as the input file. | Folder |
extent (Optional) | Specify the extent of the data that will be evaluated by this tool. | Extent |
boundary (Optional) | A polygon boundary that defines the locations where LAS files will be extracted. | Feature Layer |
process_entire_files (Optional) | Specify how the processing extent is applied.
| Boolean |
name_suffix (Optional) | The text that will be appended to the name of each output LAS file. Each file will inherit its base name from its source file, followed by the suffix specified in this parameter. | String |
remove_VLR (Optional) | Determines whether to remove the additional variable length records or to keep them in the LAS files.
| Boolean |
rearrange_points (Optional) | Determines whether to rearrange points in the LAS files.
| Boolean |
compute_stats (Optional) | Specifies whether statistics should be computed for the LAS files referenced by the LAS dataset. The presence of statistics allows the LAS dataset layer's filtering and symbology options to only show LAS attribute values that exist in the LAS files.
| Boolean |
out_las_dataset (Optional) | The output LAS Dataset. | LAS Dataset |
Code sample
ExtractLas example 1 (Python window)
The following sample demonstrates the use of this tool in the Python window.
import arcpy
from arcpy import env
env.workspace = 'C:/data'
arcpy.ddd.ExtractLas('test.lasd', 'c:/lidar/subset', boundary='study_area.shp',
name_suffix='subset', remove_vlr=True,
rearrange_points='REARRANGE_POINTS',
out_las_dataset='extracted_lidar.lasd')
ExtractLas example 2 (stand-alone script)
The following sample demonstrates the use of this tool in a stand-alone Python script.
'''****************************************************************************
Name: Split Large LAS File
Description: Divides a large LAS file whose point distribution covers the full
XY extent of the data into smaller files to optimize performance
when reading lidar data.
****************************************************************************'''
# Import system modules
import arcpy
import tempfile
import math
in_las_file = arcpy.GetParameterAsText(0)
tile_width = arcpy.GetParameter(1) # double in LAS file's XY linear unit
tile_height = arcpy.GetParameter(2) # double in LAS file's XY linear unit
out_folder = arcpy.GetParameterAsText(3) # folder for LAS files
out_name_suffix = arcpy.GetParameterAsText(4) # basename for output files
out_lasd = arcpy.GetParameterAsText(5) # output LAS dataset
try:
temp_lasd = arcpy.CreateUniqueName('temp.lasd', tempfile.gettempdir())
arcpy.management.CreateLasDataset(in_las_file, temp_lasd,
compute_stats='COMPUTE_STATS')
desc = arcpy.Describe(temp_lasd)
total_columns = int(math.ceil(desc.extent.width/tile_width))
total_rows = int(math.ceil(desc.extent.height/tile_height))
digits = int(math.log10(max(cols, rows))) + 1
for row in range(1, total_rows+1):
yMin = desc.extent.YMin + tile_height*(row-1)
yMax = desc.extent.YMin + tile_height*(row)
for col in range (1, total_columns+1):
xMin = desc.extent.XMin + tile_width*(col-1)
xMax = desc.extent.XMax + tile_width*(col)
name_suffix = '_{0}_{1}x{2}'.format(out_name_suffix,
str(row).zfill(digits),
str(col).zfill(digits))
arcpy.ddd.ExtractLas(temp_lasd, out_folder,
arcpy.Extent(xMin, yMin, xMax, yMax),
name_suffix=name_suffix,
rearrange_points='REARRANGE_POINTS',
compute_stats='COMPUTE_STATS')
arcpy.env.workspace = out_folder
arcpy.management.CreateLasDataset(arcpy.ListFiles('*{0}*.las'.format(out_name_suffix)),
out_lasd, compute_stats='COMPUTE_STATS',
relative_paths='RELATIVE_PATHS')
except arcpy.ExecuteError:
print(arcpy.GetMessages())
Environments
Licensing information
- ArcGIS for Desktop Basic: Requires 3D Analyst
- ArcGIS for Desktop Standard: Requires 3D Analyst
- ArcGIS for Desktop Advanced: Requires 3D Analyst