Available with Spatial Analyst license.
Summary
Defines the neighbor that is the next cell on the least accumulative cost path to the nearest source.
Illustration
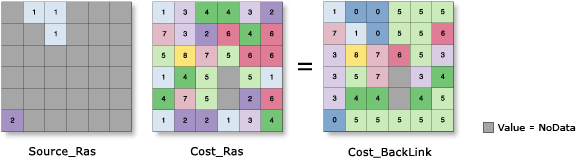
Usage
The input source data can be a feature class or raster.
When the input source data is a raster, the set of source cells consists of all cells in the source raster that have valid values. Cells that have NoData values are not included in the source set. The value 0 is considered a legitimate source. A source raster can be easily created using the extraction tools.
-
When the input source data is a feature class, the source locations are converted internally to a raster before performing the analysis. The resolution of the raster can be controlled with the Cell Size environment. By default, the resolution will be set to the resolution of the input cost raster.
When using polygon feature data for the input source data, care must be taken with how the output cell size is handled when it is coarse, relative to the detail present in the input. The internal rasterization process employs the same default Cell assignment type method as the Polygon to Raster tool, which is CELL_CENTER. This means that data not located at the center of the cell will not be included in the intermediate rasterized source output, and so will not be represented in the distance calculations. For example, if your sources are a series of small polygons, such as building footprints, that are small relative to the output cell size, it is possible that only a few of them will fall under the centers of the output raster cells, seemingly causing most of the others to be lost in the analysis.
To avoid this situation, as an intermediate step, you could rasterize the input features directly with the Polygon to Raster tool and set a Priority field, and use the resulting output as input to the Distance tool. Alternatively, you could select a small enough cell size to capture the appropriate amount of detail from the input features.
Cell locations with NoData in the Input cost raster act as barriers in the cost surface tools. Any cell location that is assigned NoData on the input cost surface will receive NoData on all output rasters (cost distance, allocation, and back link).
If the input source data and the cost raster are different extents, the default output extent is the intersection of the two. To get a cost distance surface for the entire extent, choose the Union of Inputs option on the output Extent environment settings.
If a Mask has been set in the environment, all masked cells will be treated as NoData values.
When a mask has been defined in the Raster Analysis window and the cells to be masked will mask a source, the calculations will occur on the remaining source cells. The source cells that are masked will not be considered in the computations. These cell locations will be assigned NoData on all outputs (distance, allocation, and back link) rasters.
The Maximum distance is specified in the same cost units as those on the cost raster.
-
For the output distance raster, the least-cost distance (or minimum accumulative cost distance) of a cell to a set of source locations is the lower bound of the least-cost distances from the cell to all source locations.
The characteristics of the source, or the movers from a source, can be controlled by the Source cost multiplier (the mode of travel or magnitude at the source), Source start cost (starting cost before the movement begins), Source resistance rate (a dynamic adjustment accounting for the impact of accumulated cost, for example, simulating how much a hiker is getting fatigued), and Source capacity (how much cost can a source assimilate before reaching its limit).
If any of the source characteristics parameters are specified using a value, that value is applied to all of the sources. If the parameters are specified through fields associated with the source, the values in the table will be uniquely applied to the corresponding sources.
See Analysis environments and Spatial Analyst for additional details on the geoprocessing environments that apply to this tool.
Syntax
CostBackLink (in_source_data, in_cost_raster, {maximum_distance}, {out_distance_raster}, {source_cost_multiplier}, {source_start_cost}, {source_resistance_rate}, {source_capacity})
Parameter | Explanation | Data Type |
in_source_data |
The input source locations. This is a raster or feature dataset that identifies the cells or locations to which the least accumulated cost distance for every output cell location is calculated. For rasters, the input type can be integer or floating point. | Raster Layer | Feature Layer |
in_cost_raster | A raster defining the impedance or cost to move planimetrically through each cell. The value at each cell location represents the cost-per-unit distance for moving through the cell. Each cell location value is multiplied by the cell resolution while also compensating for diagonal movement to obtain the total cost of passing through the cell. The values of the cost raster can be integer or floating point, but they cannot be negative or zero (you cannot have a negative or zero cost). | Raster Layer |
maximum_distance (Optional) | Defines the threshold that the accumulative cost values cannot exceed. If an accumulative cost distance value exceeds this value, the output value for the cell location will be NoData. The maximum distance defines the extent for which the accumulative cost distances are calculated. The default distance is to the edge of the output raster. | Double |
out_distance_raster (Optional) | The output cost distance raster. The cost distance raster identifies, for each cell, the least accumulative cost distance over a cost surface to the identified source locations. A source can be a cell, a set of cells, or one or more feature locations. The output raster is of floating-point type. | Raster Dataset |
source_cost_multiplier (Optional) | Multiplier to apply to the cost values. Allows for control of the mode of travel or the magnitude at a source. The greater the multiplier, the greater the cost to move through each cell. The values must be greater than zero. The default is 1. | Double | Field |
source_start_cost (Optional) | The starting cost from which to begin the cost calculations. Allows for the specification of the fixed cost associated with a source. Instead of starting at a cost of zero, the cost algorithm will begin with the value set by source_start_cost. The values must be zero or greater. The default is 0. | Double | Field |
source_resistance_rate (Optional) | The rate to multiply the accumulative cost to determine the resistance adjustment. This parameter simulates the increase in the effort to overcome costs as the accumulative cost increases. This is used to model fatigue of the traveler. The growing accumulative cost to reach a cell is multiplied by the resistance rate and added to the cost to move into the subsequent cell. The greater the resistance rate, the more additional cost is added to reach the next cell, which is compounded for each subsequent movement. Since the resistance rate is similar to a compound rate and generally the accumulative cost values are very large, small resistance rates are suggested, such as 0.02, 0.005, or even smaller, depending on the accumulative cost values. The values must be zero or greater. The default is 0. | Double | Field |
source_capacity (Optional) | Defines the cost capacity for the traveler from a source. Each source grows to the specified capacity. The values must be greater than zero. The default capacity is to the edge of the output raster. | Double | Field |
Return Value
Name | Explanation | Data Type |
out_backlink_raster | The output cost back-link raster. The back-link raster contains values of 0 through 8, which define the direction or identify the next neighboring cell (the succeeding cell) along the least accumulative cost path from a cell to reach its least cost source. If the path is to pass into the right neighbor, the cell will be assigned the value 1, 2 for the lower right diagonal cell, and continuing clockwise. The value 0 is reserved for source cells. ![]() | Raster |
Code sample
CostBackLink example 1 (Python window)
The following Python Window script demonstrates how to use the CostBackLink tool.
import arcpy
from arcpy import env
from arcpy.sa import *
env.workspace = "C:/sapyexamples/data"
outBacklink = CostBackLink("observers","costraster", "",
"c:/sapyexamples/output/distRast", "Multiplier", "StartCost", "Resistance", 500000)
outBacklink.save("c:/sapyexamples/output/backlink")
CostBackLink example 2 (stand-alone script)
Create a back link raster defining the direction of the next cell in least accumulated cost path analysis.
# Name: CostBackLink_Ex_02.py
# Description: Defines the neighbor that is the next cell on
# the least accumulative cost path to the nearest
# source.
# Requirements: Spatial Analyst Extension
# Import system modules
import arcpy
from arcpy import env
from arcpy.sa import *
# Set environment settings
env.workspace = "C:/sapyexamples/data"
# Set local variables
inSource = "observers.shp"
inCostRaster = "costraster"
inMaxDist = ""
outDistRast = "c:/sapyexamples/output/distRast"
multiplier = "Multiplier"
startCost = "StartCost"
resistance = "Resistance"
capacity = 500000
# Check out the ArcGIS Spatial Analyst extension license
arcpy.CheckOutExtension("Spatial")
# Execute CostBackLink
outBacklink = CostBackLink(inSource,inCostRaster, inMaxDist,
outDistRast, multiplier, startCost,
resistance, capacity)
# Save the output
outBacklink.save("c:/sapyexamples/output/backlink.tif")
Environments
Licensing information
- ArcGIS Desktop Basic: Requires Spatial Analyst
- ArcGIS Desktop Standard: Requires Spatial Analyst
- ArcGIS Desktop Advanced: Requires Spatial Analyst