In this topic
About tool parameter domains
Domains can be defined to specify fixed input values for a custom geoprocessing function tool's parameters or to filter parameter values. For example, you can assign a domain to an input parameter of the integer data type and restrict its value to a range of 1 to 100. Or you can define a tool input parameter to accept only shapefiles.
The following screen shot shows the Clip tool dialog box. The input parameter for the XY Tolerance can have an assigned domain, and the available domain values are listed in a drop-down menu.
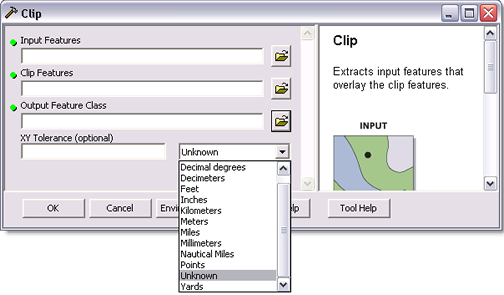
Use the GPParameter.setDomainByRef() method to assign a domain to a tool parameter. Define a parameter domain value using one of the following domain classes that implement the IGPDomain interface:
The most common scenarios for assigning a domain to an input parameter are as follows:
- Creating a fixed list of string keywords
- Defining a Boolean choice (true or false)
- Defining input feature types (point, line, or polygon)
String keywords
Domains can be assigned to an input parameter to allow the user to choose a parameter value from a fixed set of string values. For example, the Add Field tool has set Field Type values of text, long, double, short, float, date, blob, and raster as shown in the following screen shot:
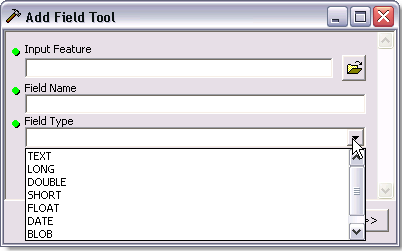
class AddFieldTool extends BaseGeoprocessingTool{
public IArray getParameterInfo()throws IOException, AutomationException{
IArray parameters = new Array();
GPParameter parameter;
//.....
//Define parameter 3: Field type
parameter = new GPParameter();
parameter.setName("in_fieldType");
parameter.setDisplayName("Field Type");
parameter.setDirection
(esriGPParameterDirection.esriGPParameterDirectionInput);
parameter.setParameterType(esriGPParameterType.esriGPParameterTypeRequired);
parameter.setDataTypeByRef(new GPStringType());
parameter.setValueByRef(new GPString());
//Define domain values (keywords) for the Field Type parameter.
IGPCodedValueDomain domain = new GPCodedValueDomain();
domain.addStringCode("TEXT", "TEXT");
domain.addStringCode("LONG", "LONG");
domain.addStringCode("DOUBLE", "DOUBLE");
domain.addStringCode("SHORT", "SHORT");
domain.addStringCode("FLOAT", "FLOAT");
domain.addStringCode("DATE", "DATE");
domain.addStringCode("BLOB", "BLOB");
domain.addStringCode("RASTER", "RASTER");
//Assign the domain to the parameter.
parameter.setDomainByRef((IGPDomain)domain);
parameters.add(parameter);
}
//Implement other methods.
}
The keywords for domain values are hard coded in the geoprocessing function tool and are not changed once the tool is built. Do not put keywords in a resource file since they can be inadvertently localized, which could break your code. Additional good practices for creating keywords are as follows:
- Type keywords in all capital letters.
- List keywords with their default keyword first. In documentation, the default keyword is followed by other keywords, separated by a vertical bar (|). (For example, TEXT | DOUBLE | FLOAT).
- Do not localize keywords.
Boolean parameters
In a tool dialog box, Boolean parameters are listed as check box options; in the command line and scripting, they are listed as two strings. Always create a coded value domain for a Boolean parameter.
The following code snippet shows how to create a coded value domain with Boolean values and corresponding string codes and how to assign the domain to a parameter:
Always create a coded value domain for a Boolean parameter.
class CustomJavaTool extends BaseGeoprocessingTool{
public IArray getParameterInfo()throws IOException, AutomationException{
//....
GPParameter parameter = new GPParameter();
//Set other properties.
// Always create a coded value domain for Boolean parameters.
IGPCodedValueDomain bool_domain = new GPCodedValueDomain();
IGPValue bool_value = new GPBoolean();
bool_value.setAsText("true");
bool_domain.addCode(bool_value, "CONTINUE_COMPARE");
bool_value = new GPBoolean();
bool_value.setAsText("false");
bool_domain.addCode(bool_value, "NO_CONTINUE_COMPARE");
parameter.setDomainByRef((IGPDomain)bool_domain);
//.....
}
//Override other required methods of BaseGeoprocessingTool.
}
Feature types
If the input values of a parameter are features—that is, points, lines, or polygons—you can use a domain to build the list of layers or features in the catalog minibrowser. Filtering occurs when the data type validates the value. When using getParameterInfo(), the input feature class parameter is built with a domain to filter the input feature classes so only polygon features are used as shown in the following code:
[Java]
class CustomJavaTool extends BaseGeoprocessingTool{
public IArray getParameterInfo()throws IOException, AutomationException{
IArray parameters = new Array();
GPParameter inputParameter = new GPParameter();
//Define other properties for the parameter.
//......
// Define the domain to filter the input features to polygon features only.
IGPFeatureClassDomain fcDomain = new GPFeatureClassDomain();
fcDomain.addFeatureType(esriFeatureType.esriFTSimple);
fcDomain.addType(esriGeometryType.esriGeometryPolygon);
inputParameter.setDomainByRef((IGPDomain)fcDomain);
parameters.add(inputParameter);
//....Define other parameters of the tool.
}
//Override other required methods of BaseGeoprocessingTool.
}
Dynamic domains
Domain values can be assigned or the values can be modified dynamically. You can use dynamic domains when you want keywords in one parameter to be based on the value of another parameter. For example, you want one set of keywords if point features are input, and another set if line features are input. In this case, you can modify the domain values dynamically using the BaseGeoprocessingTool.updateParameters() method. Another example is a coded value domain of SDE configuration keywords. The domain list changes based on the workspace parameter used.
The following code snippet shows how to modify domains using the updateParameters() method. When the value for parameter 1 is input (GPBoolean datatype), parameter 3 (GPString datatype) is enabled, and its domain is assigned dynamically by the updateParameters() method.
[Java]
class CustomJavaTool extends BaseGeoprocessingTool{
public void updateParameters(IArray paramvalues, IGPEnvironmentManager envMgr){
// Get the Boolean parameter value for this tool.
IGPBoolean val1 = (IGPBoolean)gpUtilities.unpackGPValue
(paramvalues.getElement(1));
// Get the coded value parameter.
IGPParameterEdit3 cvParam = (IGPParameterEdit3)paramvalues.getElement(3);
// If the Boolean parameter value is true, update the domain with new values.
if (val1.isValue()){
//Enable paramater 3.
cvParam.setEnabled(true);
//Create the domain.
IGPCodedValueDomain cvDomain = new GPCodedValueDomain();
cvDomain.addStringCode("Seconds", "Seconds");
cvDomain.addStringCode("Feet", "Feet");
cvParam.setDomainByRef((IGPDomain)cvDomain);
}
}
See Also:
Geoprocessing tool parameter data typesCustomizing a tool dialog
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |
Server | Server |
ArcGIS for Desktop Standard | ArcGIS for Desktop Basic |
ArcGIS for Desktop Basic | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |