Creating a multipart polyline
This example uses an existing polyline to define a new, multipart polyline. In the following illustration, the input polyline is shown in the background and the new polyline is shown in dark green with its vertices marked. Each part of the new polyline is a single segment normal to a segment from the original polyline, incident at its midpoint, and one-third of its length.
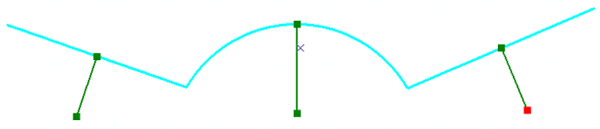
See the following code example:
[Java]
static IPolyline constructMultiPartPolyline(IPolyline inputPolyline)throws Exception{
IGeometry outGeometry = new Polyline();
//Always associate new, top-level geometries with an appropriate spatial reference.
outGeometry.setSpatialReferenceByRef(inputPolyline.getSpatialReference());
IGeometryCollection geometryCollection = (IGeometryCollection)outGeometry;
ISegmentCollection segmentCollection = (ISegmentCollection)inputPolyline;
//Iterate over existing polyline segments using a segment enumerator.
IEnumSegment segments = segmentCollection.getEnumSegments();
ISegment[] currentSegment = new ISegment[1];
int[] partIndex = new int[1];
int[] segmentIndex = new int[1];
segments.next(currentSegment, partIndex, segmentIndex);
while (currentSegment != null){
ILine normal = new Line();
//Geometry methods with _Query_ in their name expect to modify existing geometries.
//In this case, the QueryNormal method modifies an existing line
//segment (normal) to be the normal vector to
//currentSegment at the specified location along currentSegment.
currentSegment[0].queryNormal(esriSegmentExtension.esriNoExtension, 0.5,
true, currentSegment[0].getLength() / 3, normal);
//Since each normal vector is not connected to others, create a new path for each one.
ISegmentCollection newPath = new Path();
newPath.addSegment((ISegment)normal, null, null);
//The spatial reference associated with geometryCollection will be assigned to all incoming paths and segments.
geometryCollection.addGeometry((IGeometry)newPath, null, null);
segments.next(currentSegment, partIndex, segmentIndex);
}
//The geometryCollection now contains the new, multipart polyline.
return (IPolyline)geometryCollection;
}
Development licensing | Deployment licensing |
---|---|
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |
Engine Developer Kit | Engine |