In this topic
- About dynamic drawing APIs to create dynamic content
- Drawing dynamic symbols
- Dynamic glyphs
- Dynamic glyphs groups
- Compound and array markers
- DrawCompoundMarkerN method
- DrawArrayMarker method
Dynamic content can be drawn on a dynamic map using the following dynamic draw callbacks:
- IDynamicLayer.drawDynamicLayer() method
- IDynamicMapEvents.afterDynamicDraw method (event handler) with the dynamic map draw phase, esriDynamicMapDrawPhase.esriDMDPDynamicLayers
- IDynamicMapEvents.beforeDynamicDraw method (event handlers) with the dynamic map draw phase, esriDynamicMapDrawPhase.esriDMDPDynamicLayers
When invoking dynamic draw callbacks, the dynamic display (DD) framework creates an active OpenGL rendering context internally and passes a reference to an active DynamicDisplay object; therefore, in the dynamic draw callbacks, the DynamicDisplay drawing APIs and OpenGL APIs can be utilized to draw any dynamic content on the underlying map.
Any usage of the DynamicDisplay drawing API (or OpenGL API) in any other method results in an unknown behavior.
The DynamicDisplay object has the following four active dynamic symbols to draw dynamic objects:
- Dynamic marker symbol (esriDSymbolMarker)
- Dynamic line symbol (esriDSymbolLine)
- Dynamic text symbol (esriDSymbolText)
- Dynamic fill symbol (esriDSymbolFill)
When the drawing APIs are invoked, the dynamic drawing is determined by the properties of these four dynamic symbols. The combination of dynamic symbols are used by the DD framework to render marker, multiple markers, line, multiple lines, polyline, polygon, rectangle, text, and compound items (that include a marker and text strings around it). The following table shows the correlation of the specified geometries to each dynamic symbol:
Draw method
|
Dynamic
marker
symbol |
Dynamic text
symbol |
Dynamic line
symbol |
Dynamic fill
symbol |
Marker
|
+
|
|
|
|
MultipleMarkers
|
+
|
|
|
|
Line
|
|
|
+
|
|
MultipleLines
|
|
|
+
|
|
Polyline
|
|
|
+
|
|
Polygon
|
|
|
+
(polygon's outline) |
+
polygon's fill |
Rectangle
|
|
|
+
(rectangle's outline) |
+
(rectangle's fill) |
Text
|
|
+
|
+
(text box outline) |
+
(text box fill) |
CompoundMarker*
|
+
|
+
|
+
(text box outline) |
+
(text box fill) |
ArrayMarker
|
+
|
+
|
+
(text box outline) |
+
(text box fill) |
Dynamic symbol properties, such as color, size, and so on, can be altered using methods and properties on IDynamicSymbolProperties2. The following table shows the dynamic symbol properties that can be modified using IDynamicSymbolProperties2:
Property
|
Description
|
G/S*
|
Text
symbol |
Marker
symbol |
Line
symbol |
Fill
symbol |
DynamicGlyph
|
Dynamic glyph for the specified dynamic symbol
|
G
|
+
|
+
|
+
|
+
|
Color
|
Color for the specified dynamic symbol
|
G
|
+
|
+
|
+
|
+
|
Scale(x,y)
|
Scales the dynamic symbol.
|
G
|
+
|
+
|
+
|
+
|
Smooth
|
Indicates if the specified dynamic symbol is smooth;
only valid for text, line, and marker |
G
|
+
|
+
|
+
|
+
|
Offset(x,y)
|
Offsets the dynamic symbol
|
G
|
+
|
+
|
+
|
+
|
UseReferenceScale
|
Indicates if the specified dynamic symbol conforms to the map reference scale
|
G
|
+
|
+
|
+
|
+
|
Heading
|
Heading for the specified dynamic symbol; only valid if dynamic symbol rotation alignment is a heading, and for
text and marker |
G
|
+
|
+
|
|
+
|
RotationAlignment
|
Rotation alignment
for the specified dynamic symbol; only valid for text and marker |
G
|
+
|
+
|
|
+
|
TextVerticalAlignment
|
Indicates vertical alignment for the
text dynamic symbol |
S
|
+
|
|
|
|
TextHorizontalAlignment
|
Indicates the horizontal alignment for the text
dynamic symbol |
S
|
+
|
|
|
|
TextLeading
|
Indicates the text leading for the dynamic text
symbol |
S
|
+
|
|
|
|
TextCharacterSpacing
|
Indicates an
additional space added to each character beyond what is defined by its character box in the TextGlyph |
S
|
+
|
|
|
|
TextWordSpacing
|
Indicates an
additional space added between words in the text string |
S
|
+
|
|
|
|
TextRightToLeft
|
Indicates if the
text is drawn from right-to-left for the dynamic text symbol |
S
|
+
|
|
|
|
TextBoxHorizontalAlignment
|
Text box horizontal alignment for the dynamic text symbol
|
S
|
+
|
|
|
|
TextBoxUseDynamicFillSymbol
|
Indicates whether
to use the dynamic fill symbol when drawing the text |
S
|
+
|
|
|
|
TextBoxUseDynamicLineSymbol
|
Indicates whether
to use the dynamic line symbol when drawing the text |
S
|
+
|
|
|
|
TextBoxMargins
|
Text box margins
for the dynamic text symbol |
S
|
+
|
|
|
|
LineContinuePattern
|
Indicates if the line pattern is continued
or restarted for multipart line drawings |
S
|
|
|
+
|
|
*IDynamicSymbolProperties2 has two types of methods and properties—Generic (G) and Specific (S).
Generic takes an extra parameter, type of dynamic symbol, and changes the state of the passed in dynamic symbol type. Specific are distinct to a dynamic symbol type; therefore, their names start with the type of dynamic symbol; for example, text leading is specific to a dynamic text symbol.
|
When drawing dynamic symbols, declare IDynamicGlyphFactory, and IDynamicSymbolProperties2 as class variables considering the repetition of their drawing sequences.
//This method creates and draws a marker glyph on a dynamic layer.
private void drawDynamicSymbols(IDynamicDisplay dynamicDisplay, IPoint point)throws
AutomationException, IOException{
// Step 1: Create glyphs from IDynamicGlyphFactory.
if (!dynamicGlyphsCreated){
dynamicSymbolProps = (IDynamicSymbolProperties2)dynamicDisplay;
dynamicGlyphFactory = dynamicDisplay.getDynamicGlyphFactory();
//Implement a method to create a glyph as specified in the next section.
this.createMarkerGlyph();
dynamicGlyphsCreated = true;
}
//Step 2: Modify dynamic symbol properties using IDynamicSymbolProperties2.
dynamicSymbolProps.setRotationAlignment(esriDynamicSymbolType.esriDSymbolMarker,
esriDynamicSymbolRotationAlignment.esriDSRAScreen);
dynamicSymbolProps.setScale(esriDynamicSymbolType.esriDSymbolMarker, 0.8f, 0.8F);
dynamicSymbolProps.setColor(esriDynamicSymbolType.esriDSymbolMarker, 0.0f, 1.0f,
0.0f, 1.0f);
//Step 3: Assign the glyph to the dynamic symbol.
dynamicSymbolProps.setDynamicGlyphByRef(esriDynamicSymbolType.esriDSymbolMarker,
markerGlyph);
//Step 4: Draw the dynamic symbol using the DynamicDisplay object.
dynamicDisplay.drawMarker(point);
}
Dynamic glyphs
A Dynamic glyph is a handle to a graphic resource that is rendered as a dynamic symbol. Dynamic glyphs can be created using the IDynamicGlyphFactory from file, a symbol, a glyphs group, or a handle to a bitmap. The following are the four types of dynamic glyphs:
- Dynamic marker glyph (esriDGlyphMarker)
- Dynamic line glyph (esriDGlyphLine)
- Dynamic text glyph (esriDGlyphText)
- Dynamic fill glyph (esriDGlyphFill)
It is important to correctly manage dynamic glyphs to minimize the application memory usage. Incorrect management of dynamic glyphs lead to over consumption of resources and can result in poor performance and application crashes. Minimize the dynamic glyphs creation and reuse created dynamic glyphs as much as possible. For example, create a dynamic glyph from a white symbol, set the dynamic symbol’s glyph property to use the created dynamic glyph, and use the dynamic symbol’s color and scale properties to render the dynamic symbol (that uses one created dynamic glyph) in different colors and sizes.
When a dynamic glyph is no longer in use, use the IDynamicGlyphFactory.deleteDynamicGlyph() method to release the dynamic glyph’s resources.
The dynamic glyphs factory is a singleton object; therefore, you can always cocreate and use it to create and delete dynamic glyphs. Performance wise, create dynamic glyphs in the context of IDynamicLayer.drawDynamicLayer in the esriDDPImmediate draw phase, in the context of the IDynamicMapEvents.beforeDynamicDraw, or in the IDynamicMapEvents.afterDynamicDraw methods, while casting the passed in IDynamicDisplay interface to IDynamicGlyphFactory.
See the following code example:
[Java]
//This method creates a simple marker glyph from a CharacterMarkerSymbol.
private void createDynamicGlyphs(){
try{
// Step 1: Create a character marker symbol.
CharacterMarkerSymbol characterMarkerSymbol = new CharacterMarkerSymbol();
RgbColor color = new RgbColor();
color.setRGB(0xFFFFFF);
characterMarkerSymbol.setColor(color);
characterMarkerSymbol.setSize(40);
characterMarkerSymbol.setCharacterIndex(72);
// Step 2: Create a glyph from a character marker symbol using IDynamicGlyphFactory.
markerGlyph = dynamicGlyphFactory.createDynamicGlyph((ISymbol)
characterMarkerSymbol);
}
catch (Exception e){
e.printStackTrace();
}
}
A dynamic glyphs group is a container that holds multiple glyphs. It is composed of a bitmap and database that keeps information about the group and elements (glyphs and glyphs items) in the group. The image is a mosaic of subimages of icons (marker glyphs), lines (line glyphs), and groups of characters (each group composes a text glyph).
The bitmap keeps color information (red, green, and blue [RGB], and alpha values) for each pixel. The bitmap can be loaded from the following two image files:
- One that holds the RGB values
- One that holds the alpha values or from a single image file that holds RGB and alpha (RGBA) values
The glyphs group database is loaded from an Extensible Markup Language (XML) file. This XML file holds general information about the group, specific location, and size for each item and element of the group.
A new glyphs group can be loaded using IDynamicGlyphFactory.loadDynamicGlyphsGroup. The method creates a glyphs group and returns an allocated glyphs group ID. The returned ID is a positive integer. See the following:
- Group ID 0—Refers to rendering without using a graphical resource, which means simple lines, simple points, and so on.
- Group ID 1—Reserved as the default glyphs group (there is a default one that exists with the dynamic display) and supplies a default set of dynamic glyphs.
To replace the preloaded default group, delete group ID 1, and immediately create a group. The dynamic map assigns the new group ID 1 and uses it as the new default glyphs group.
Performance wise, it is preferable to create a glyph group with multiple glyphs and use the glyphs from the group, rather than creating glyphs from individual image files.
Compound and array markers
It is frequently required to draw a marker with text strings around it. For ease of use, use the IDynamicCompoundMarker2 interface, which provides helper methods that draw a marker with text strings around it. You can also control the text distance from the marker, spacing between the text strings, and so on, using the methods and properties of IDynamicCompoundMarker2. The following are the two most significant methods of IDynamicCompoundMarker2:
- IDynamicCompoundMarker2.drawCompoundMarkerN()
- IDynamicCompoundMarker2.drawArrayMarker()
DrawCompoundMarkerN methods draw a marker where the n value is 1, 2, 4, 8, or 10. The n value specifies the number of text strings around the marker. These methods use map coordinates to draw text and its corresponding marker.
The following illustration shows the layout of the drawCompoundMarker10() method:
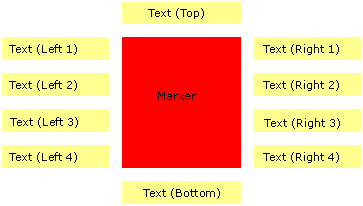
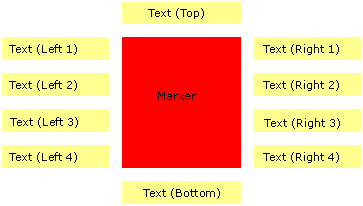
See the following code example:
[Java]
// This method creates and draws a marker glyph, two text labels, and
// the x,y location on the top and bottom of the marker.
private void drawDynamicSymbols(IDynamicDisplay dynamicDisplay, IPoint point)throws
AutomationException, IOException{
// Step 1: Create glyphs from IDynamicGlyphFactory.
//Create glyphs only once since they are expensive.
if (!dynamicGlyphsCreated){
dynamicSymbolProps = (IDynamicSymbolProperties2)dynamicDisplay;
dynamicGlyphFactory = dynamicDisplay.getDynamicGlyphFactory();
dynamicCompoundMarker = (IDynamicCompoundMarker2)dynamicDisplay;
// Write a method to create glyphs as specified in the preceding section.
this.createMarkerGlyph();
dynamicGlyphsCreated = true;
}
// Step 2: Modify symbol properties using IDynamicSymbolProperties2.
dynamicSymbolProps.setRotationAlignment(esriDynamicSymbolType.esriDSymbolMarker,
esriDynamicSymbolRotationAlignment.esriDSRAScreen);
dynamicSymbolProps.setScale(esriDynamicSymbolType.esriDSymbolMarker, 0.8f, 0.8F);
dynamicSymbolProps.setColor(esriDynamicSymbolType.esriDSymbolMarker, 0.0f, 1.0f,
0.0f, 1.0f);
// Step 3: Assign the glyph to the dynamic symbol.
dynamicSymbolProps.setDynamicGlyphByRef(esriDynamicSymbolType.esriDSymbolMarker,
markerGlyph);
// Step 4: Draw the item as a compund marker, which means you do not have to draw
// the items and its accompanying labels separately; thus allowing you to write less code
// and get better performance.
dynamicCompoundMarker.drawCompoundMarker2(point, String.valueOf(point.getX()),
String.valueOf(point.getY()));
}
DrawArrayMarker methods draw markers with arrays of text strings in each of the five regions around the marker. The regions are center, left, right, top, and bottom. This method can draw a marker on a location in map coordinates, whereas to draw a marker on a location in screen (pixel) coordinates uses the drawScreenArrayMarker() method.
The following illustration shows the layout of the drawArrayMarker method with the input of arrays of sizes—Center[4], Left[5], Right[5], Top[2], and Bottom[3]:
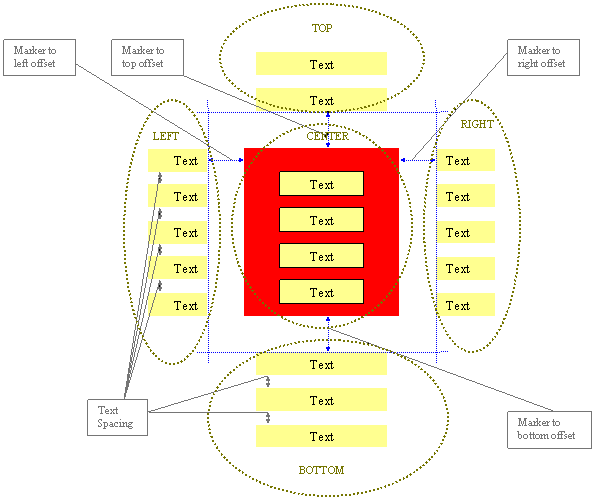
See Also:
About dynamic displayHow to draw a bitmap element using OpenGL
How to create a dynamic glyph from a marker symbol
Sample:Dynamic Layer WalkThrough
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |