In this topic
- ArcObjects API for developing SOEs
- SOE annotations
- Interfaces and classes
- ArcGIS Java ArcObjects Software Development Kit
- Eclipse IDE wizards for SOE development and export
ArcObjects API for developing SOEs
A server object extension can be defined by implementing the com.esri.arcgis.server.IServerObjectExtension interface.
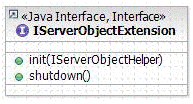
This interface is mandatory for SOEs, and includes two methods: init() and shutdown(). This interface is used by the server object to manage the lifetime of the SOE. The server object creates the SOE and calls the init() method, handing it back a reference to the server object via the ServerObjectHelper argument. The ServerObjectHelper implements a weak reference, one that is not protected from the garbage collector, on the server object. The extension can keep a strong reference on the server object helper (for example, in a member variable), but this is not recommended as a strong reference can cause unnecessary objects to remain in memory and hinder performance. An extension should get the server object from the server object helper in order to make any method calls on the server object, then release the reference after making the method calls.
SOE annotations
Annotations are used inside Java SOEs to indicate that a Java class is an ArcGIS extension and to hold metadata that ArcGIS for Server requires to deploy and manage SOEs at run time. At ArcGIS 10.1 and above, Java SOEs support two types of annotations:
@ArcGIS Extension
An @ArcGISExtension annotation indicates that the annotated interface or class is automatically exposed to the ArcGIS platform as an ArcGIS extension. The @ArcGISExtension annotation is used by ArcGIS as a way to associate your interface and classes as extensions without the need for any configuration or API calls embedded in some external source. The following is an example of an annotated interface and class for a SOE:
[Java]
// Custom Interface
@ArcGISExtension public interface ISoeInterface{
public String mySoeFoo();
}
// SOE class
@ArcGISExtension public class SOE implements IServerObjectExtension, IMySoeInterface{
// IServerObjectExtension methods
public void init(IServerObjectHelper arg0)throws IOException,
AutomationException{
// Called once when the instance of the SOE is created
}
public void shutdown()throws IOException, AutomationException{
// Called once when the SOE’s context is shut down
}
//IMySoeInterface method
public String mySoeFoo(){
return "some string";
}
}
This annotation is mandatory, and its absence will render the SOE’s Java class invisible to ArcGIS for Server at deployment time. Consequently, this SOE will not exist at run time.
@ServerObjectExtProperties
An @ServerObjectExtProperties annotation is required by an SOE to hold name-value pairs that are supplied to ArcGIS for Server when your SOE is deployed as an extension to a map service.
The following element-pairs are supported:
- displayName—This is the display name your SOE will have when users enable it as a capability in an ArcGIS for Server administrative client such as ArcGIS Server Manager and the Catalog window in ArcMap. This name can have spaces.
- description—This is used to describe your SOE in a more detailed and user-friendly manner, and will show up in an ArcGIS for Server administrative client to help administrators understand the usage of your SOE.
- properties—This is where you can define properties on your SOE. For example, if an SOE allows editing of a layer, a property value could dictate which layer would be available for editing, thus giving the administrator control over run time usage/behavior of the SOE.
- allSOAPCapabilities—When exposing your SOE as a web service, you can create functionality that can be enabled or disabled by an administrator of ArcGIS for Server. Such functionality is called a “web capability”. This parameter’s value is a comma-separated list that holds all capabilities exposed by an SOE.
- defaultSOAPCapabilites—This parameter’s value lists all web capabilities that are enabled on the SOE by default.
The following code snippet demonstrates use of the above-mentioned annotations and available attributes, along with their values:
[Java]
// SOE class
@ArcGISExtension
@ServerObjectExtProperties(displayName = "My SOE", description = "My first SOE",
properties = {
"property1Name=property1Value", "property2Name=property2Value"
}
, defaultSOAPCapabilities = {
"myWebCapability1"
}
, allSOAPCapabilities = {
"myWebCapability1", "myWebCapability2"
}
)public class SOE implements IServerObjectExtension, IMySoeInterface{
public void init(IServerObjectHelper arg0)throws IOException,
AutomationException{
// Called once when the instance of the SOE is created
}
public void shutdown()throws IOException, AutomationException{
// Called once when the SOE’s context is shut down
}
//IMySoeInterface method
public String mySoeFoo(){
return "some string";
}
}
Interfaces and classes
The following are some interfaces your SOE can implement to enable specific behavior inside SOEs.
com.esri.arcgis.system.IObjectConstruct
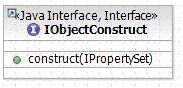
This interface is optional and needs to be implemented only if the SOE requires extra initialization such as initializing properties with values defined by a server administrator in ArcGIS Server Manager. The interface includes a single method called construct(), which is called only once when the SOE is created, after IServerObjectExtension.init() is called. For SOEs that have properties, construct() hands back the configuration properties for the SOE as a property set.
com.esri.arcgis.system.IObjectActivate
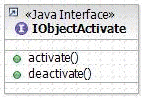
The IObjectActivate interface is optional (that is, its implementation is not required unless your SOE requires special logic to execute before and after servicing a request). It includes two methods:
- activate()—Called each time a client makes a request to the SOE, via SOAP or REST
- deactivate()—Called each time a client gets and releases the server object's context.
Any logic you implement in these methods should not be expensive.
com.esri.arcgis.server.SOAPRequestHandler
If you plan on exposing your Java SOE as a SOAP-based web service, your SOE class must then extend the SOAPRequestHandler base class. Extending this base class is not required if you are creating a REST SOE. This base class defines a handleStringRequest() method that’s exposed by the IRequestHandler interface. This method takes in SOAP requests, invokes the appropriate business method from the SOE class, generates a SOAP response, and returns it to the client application, thus relieving you of the burden of handling SOAP requests and responses and allowing you to focus on your SOE’s business logic development. This is discussed in more detail in the "Developing SOAP SOEs" topic.
com.esri.arcgis.system.IRESTRequestHandler
If you plan on exposing your Java SOE as a RESTful Web Service, you must implement the IRESTRequestHandler interface. The interface provides two methods:
- handleRESTRequest()—Called once for each HTTP request to the SOE.
- getSchema()—Called by the ArcGIS REST handler to interrogate the SOE for its resource and operations hierarchy at runtime.
If you're using the Eclipse SOE creation wizard, these methods are automatically generated, thereby allowing you to focus on your implementation of subresource and operation methods. This is discussed in more detail in the 'Developing REST SOEs' topic.
ArcGIS Java ArcObjects Software Development Kit
The ArcGIS Java ArcObjects Software Development Kit (Java AO SDK) is shipped with ArcGIS for Server but is a separate install. This SDK includes the following:
- Eclipse IDE plugins
These facilitate use of the Java ArcObjects API for developing custom applications and extensions. They include the following: - Wizards for creating custom SOAP and REST SOEs, custom geoprocessing tools, custom renderers, feature class extensions, and add-ins.
- Tools that can be used to generate deployment artifacts such as jar files for various Java extensions.
For instructions on installing ArcGIS plug-ins for Eclipse, see “Developing desktop applications” -> “Using ESRI Eclipse Plug-ins” -> “Installing ESRI Plug-ins”. - Samples
- Docs
Eclipse IDE wizards for SOE development and export
All SOEs share boilerplate code that includes initialization logic and interface implementation. This code is fairly static between SOEs and is necessary to manage the SOE life cycle. You can manually write this code or use Eclipse IDE wizards to save some time and effort. Eclipse IDE plug-ins include two wizards for SOEs:
- The SOE creation wizard—This wizard creates .java classes for REST and SOAP SOEs. It requires you to:
- Provide the SOE class name, package name, and description.
- Choose the type of SOE you're interested in creating (REST or SOAP).
- Create capabilities and properties. These are optional.
The wizard then generates .java files based on choices made in the wizard. For example, if you chose to create a SOAP SOE, then your generated files would include the SOE class file as well as an interface (more on this later). If you chose to create a REST SOE, then the interface is not mandatory, only the SOE class would be generated. If you also chose to provide your SOE with configurable properties, then a Java class file holding logic to handle such properties would also be generated.
- The SOE Export wizard—The SOE export wizard helps you export all Java classes and dependencies to a deployment container, called a .soe file. This .soe file is the only available vehicle for deploying SOEs at ArcGIS 10.1 and above, which means that SOEs written in any supported programming language need to be packaged into a .soe file for deployment. However, a single .soe file may not contain SOEs written in different languages. It must always contain SOEs written in the same supported language. If you must deploy SOEs written in different languages, use separate .soe files to package and deploy them.
For more details on using this wizard, please see the “Exporting SOAP and REST SOEs" topic in the “Java ArcObjects Developer Guide” -> “Developing Extensions” -> “Server Object Extensions” -> "Exporting SOEs" section of the ArcGIS Java Developer Help.
Development licensing | Deployment licensing |
---|---|
Server | Server |