In this topic
About working with events
If necessary, see the following topics first to get a general understanding of how to work with events:
- How to wire ArcObjects .NET events
- Delegates and the AddressOf Operator
- AddressOf Operator
- Events and Event Handlers
- Using ArcObjects (COM-based) in .NET
Wiring custom events using IActiveViewEvents
Do the following steps to wire and use events:
- Declare a delegate object variable that handles the specific event - A member variable ( m_ActiveViewEventsAfterDraw ) is declared that is global in scope for the Class Form1. This member variable is the delegate object that will be used later to hold a type-safe function pointer for the AddHandler and RemoveHandler statements. See the following code example:
Private m_ActiveViewEventsAfterDraw As ESRI.ArcGIS.Carto.IActiveViewEvents_AfterDrawEventHandler
In VB .NET, the various IActiveViewEvents handlers are not displayed in IntelliSense in Visual Studio. They are considered hidden types and do not display as a drop-down option when typing the dot (.) while coding. However, you can view the names of the hidden types in the Visual Studio Object Browser and in the ArcObjects Help system. See the following screen shot of the Object Browser:
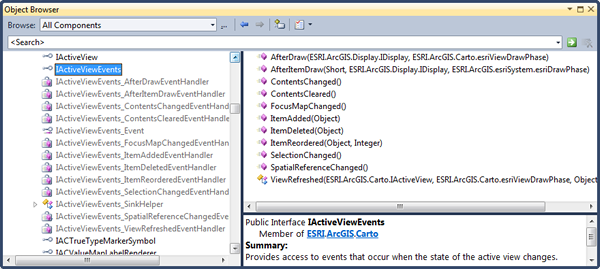
- Create an instance of the delegate using the AddressOf operator - The delegate object member variable (m_ActiveViewEventsAfterDraw) is set to an instance of the IActiveViewEvents_AfterDrawEventHandler interface. This is a type-safe pointer to the procedure (that is, a sub or function) to accomplish the work of your event. The sub, OnActiveViewEventsAfterDraw, is the procedure that does the work whenever this event is fired. See the following code example:
m_ActiveViewEventsAfterDraw=New ESRI.ArcGIS.Carto.IActiveViewEvents_AfterDrawEventHandler(AddressOf OnActiveViewEventsAfterDraw)
- Dynamically associate an event handler to the delegate object - Using the AddHandler statement, specify which ArcObjects event uses your delegate. The delegate object member variable (m_ActiveViewEventsAfterDraw) is used for the .AfterDraw event of the IActiveViewEvents_Event interface.
In ArcObjects .NET, the event interfaces all have an _Event suffix. These event interfaces (also known as outbound interfaces) are automatically suffixed with _Event by the type library importer. When programming with ArcObjects .NET, use the event interfaces with the appended _Event suffix as shown in the Visual Studio Object Browser. Again, in VB .NET, the various _Event handlers are not displayed in IntelliSense in Visual Studio. They are considered hidden types and do not display as a drop-down option when typing the dot (.) while coding. See the following screen shots:
- Dynamically remove an event handler - Using the RemoveHandler statement, specify which ArcObjects event you want to remove from a particular delegate. The delegate object member variable (m_ActiveViewEventsAfterDraw) is removed as the delegate event of the .AfterDraw event of the IActiveViewEvents_Event interface. See the following code example:
RemoveHandler CType(map, ESRI.ArcGIS.Carto.IActiveViewEvents_Event).AfterDraw, m_ActiveViewEventsAfterDraw
Dynamically adding and removing event handlers in ArcObjects .NET allows for powerful and flexible applications. Depending on your application, you can code one type of behavior (for example, inserting balloon text boxes with a mouse click) in one part of your application and a different behavior in another part of your application (for example, performing hyperlinks to other documents with a mouse click) by dynamically wiring events to custom procedures. Delegates are the mechanism for which a type-safe pointer can be utilized to use the AddHandler and RemoveHandler statements.
The following code example shows what a final product looks like using event handlers and follows the four steps previously discussed:
[VB.NET] The following code example shows what a final product looks like using event handlers and follows the four steps previously discussed:
Public Class Form1
'Step 1: Declare a delegate object variable that will handle the specific event.
Private m_ActiveViewEventsAfterDraw As ESRI.ArcGIS.Carto.IActiveViewEvents_AfterDrawEventHandler
Private Sub AddHandlerEx()
Dim application2 As ESRI.ArcGIS.Framework.IApplication=CType(EventHandlerExample.My.ThisApplication, ESRI.ArcGIS.Framework.IApplication)
Dim mxDocument As ESRI.ArcGIS.ArcMapUI.IMxDocument=application2.Document
Dim activeView As ESRI.ArcGIS.Carto.IActiveView=mxDocument.ActiveView
Dim map As ESRI.ArcGIS.Carto.IMap=activeView.FocusMap
'Step 2: Create an instance of the delegate using the AddressOf operator and add it to
'the AfterDraw event.
m_ActiveViewEventsAfterDraw=New ESRI.ArcGIS.Carto.IActiveViewEvents_AfterDrawEventHandler(AddressOf OnActiveViewEventsAfterDraw)
'Step 3: Dynamically associate an event handler to the delegate object.
AddHandler CType(map, ESRI.ArcGIS.Carto.IActiveViewEvents_Event).AfterDraw, m_ActiveViewEventsAfterDraw
End Sub
Private Sub RemoveHandlerEx()
Dim application2 As ESRI.ArcGIS.Framework.IApplication=CType(EventHandlerExample.My.ThisApplication.m_application, ESRI.ArcGIS.Framework.IApplication)
Dim mxDocument As ESRI.ArcGIS.ArcMapUI.IMxDocument=application2.Document
Dim activeView As ESRI.ArcGIS.Carto.IActiveView=mxDocument.ActiveView
Dim map As ESRI.ArcGIS.Carto.IMap=activeView.FocusMap
'Step 4: Dynamically remove an event handler.
RemoveHandler CType(map, ESRI.ArcGIS.Carto.IActiveViewEvents_Event).AfterDraw, m_ActiveViewEventsAfterDraw
End Sub
Private Sub OnActiveViewEventsAfterDraw(ByVal Display As ESRI.ArcGIS.Display.IDisplay, ByVal phase As ESRI.ArcGIS.Carto.esriViewDrawPhase)
'Add your code here.
System.Windows.Forms.MessageBox.Show("AfterDraw")
End Sub
End Class
Development licensing | Deployment licensing |
---|---|
ArcGIS Desktop Basic | ArcGIS Desktop Basic |
ArcGIS Desktop Standard | ArcGIS Desktop Standard |
ArcGIS Desktop Advanced | ArcGIS Desktop Advanced |
Engine Developer Kit | Engine |