In this topic
- About creating and modifying domains
- Creating range domains
- Creating coded value domains
- Opening and modifying domains
About creating and modifying domains
Domains are represented in the geodatabase application programming interface (API) by the abstract class, Domain. The Domain class has two subclasses - RangeDomain and CodedValueDomain - that can be used to constrain the permissible values for a particular field in an object class. Domains are associated with a field object and can be assigned at the class or subtype level.
Domains are used by ArcMap to constrain the values that can be entered into a field, as well as during the validation process within the geodatabase.
A domain can be shared by any number of fields. Domains are stored at the workspace level and are added to the workspace through IWorkspaceDomains.AddDomain.
The IDomain interface is implemented by both Domain subclasses, and provides access to the properties that are common to both, such as Name and Description. Each of the properties is read/write except for the Type property. When creating a domain, the client is required to set the Name and FieldType properties. See the following illustration:
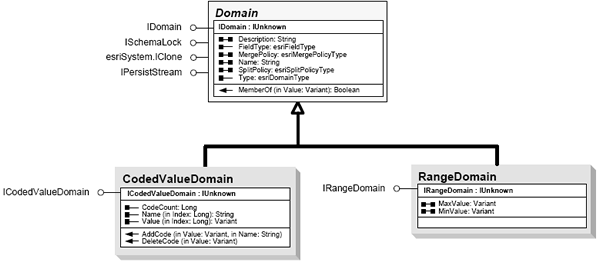
Domains are only applicable to geodatabases. File-based data sources do not support domains.
Creating range domains
Range domains can be associated with either numeric fields (that is, esriFieldTypeSmallInteger, esriFieldTypeDouble) or date (esriFieldTypeDate) fields. Range domains may not be associated with string fields, Blob fields, or other non-numeric/non-date fields.
The IRangeDomain interface allows the client to examine the minimum and maximum range values of an existing range domain, and to set the range values in a new range domain during creation.
The following code example shows the creation of a range domain for an integer field. The range domain is then persisted in the geodatabase when it is added to the workspace.
[C#] // Cast the workspace to the IWorkspaceDomains interface.
IWorkspaceDomains workspaceDomains=(IWorkspaceDomains)workspace;
// The code to create a range domain.
IRangeDomain rangeDomain=new RangeDomainClass();
rangeDomain.MinValue=0;
rangeDomain.MaxValue=255;
// The code to set the common properties for the new range domain.
IDomain domain=(IDomain)rangeDomain;
domain.Name="EightBitUnsignedInt";
domain.FieldType=esriFieldType.esriFieldTypeInteger;
domain.SplitPolicy=esriSplitPolicyType.esriSPTDuplicate;
domain.MergePolicy=esriMergePolicyType.esriMPTAreaWeighted;
// Add the new domain to the workspace.
workspaceDomains.AddDomain(domain);
[VB.NET] ' Cast the workspace to the IWorkspaceDomains interface.
Dim workspaceDomains As IWorkspaceDomains=CType(workspace, IWorkspaceDomains)
' The code to create a range domain.
Dim rangeDomain As IRangeDomain=New RangeDomainClass()
rangeDomain.MinValue=0
rangeDomain.MaxValue=255
' The code to set the common properties for the new range domain.
Dim domain As IDomain=CType(rangeDomain, IDomain)
domain.Name="EightBitUnsignedInt"
domain.FieldType=esriFieldType.esriFieldTypeInteger
domain.SplitPolicy=esriSplitPolicyType.esriSPTDuplicate
domain.MergePolicy=esriMergePolicyType.esriMPTAreaWeighted
' Add the new domain to the workspace.
workspaceDomains.AddDomain(domain)
Creating coded value domains
Coded value domains store a set of value and name pairs that represent the discrete values that a field can have. The value is what is persisted inside a field, and the name is displayed in the ArcMap and ArcCatalog attribute tables and feature inspectors. The name can be considered a human-readable string that describes what the value represents. In contrast to range domains, coded value domains can also be associated with string fields (esriFieldTypeString), with strings as values.
The ICodedValueDomain interface provides the mechanism for adding and removing the value and name pairs from a coded value domain. In addition, it provides properties that allow users to inspect the value and name pairs by index.
The following code example shows the creation of a coded value domain for a string field. The domain is then persisted in the geodatabase when it is added to the workspace.
[C#] // Cast the workspace to the IWorkspaceDomains interface.
IWorkspaceDomains workspaceDomains=(IWorkspaceDomains)workspace;
// The code to create a coded value domain.
ICodedValueDomain codedValueDomain=new CodedValueDomainClass();
// Value and name pairs.
codedValueDomain.AddCode("RES", "Residential");
codedValueDomain.AddCode("COM", "Commercial");
codedValueDomain.AddCode("IND", "Industrial");
codedValueDomain.AddCode("BLD", "Building");
// To remove a value:
codedValueDomain.DeleteCode("BLD"); // The "Building" code is removed.
// The code to set the common properties for the new coded value domain.
IDomain domain=(IDomain)codedValueDomain;
domain.Name="Building Types";
domain.FieldType=esriFieldType.esriFieldTypeString;
domain.SplitPolicy=esriSplitPolicyType.esriSPTDuplicate;
domain.MergePolicy=esriMergePolicyType.esriMPTDefaultValue;
// Add the new domain to the workspace.
workspaceDomains.AddDomain(domain);
[VB.NET] ' Cast the workspace to the IWorkspaceDomains interface.
Dim workspaceDomains As IWorkspaceDomains=CType(workspace, IWorkspaceDomains)
' The code to create a coded value domain.
Dim codedValueDomain As ICodedValueDomain=New CodedValueDomainClass()
' Value and name pairs.
codedValueDomain.AddCode("RES", "Residential")
codedValueDomain.AddCode("COM", "Commercial")
codedValueDomain.AddCode("IND", "Industrial")
codedValueDomain.AddCode("BLD", "Building")
' To remove a value:
codedValueDomain.DeleteCode("BLD") ' The "Building" code is removed.
' The code to set the common properties for the new coded value domain.
Dim domain As IDomain=CType(codedValueDomain, IDomain)
domain.Name="Building Types"
domain.FieldType=esriFieldType.esriFieldTypeString
domain.SplitPolicy=esriSplitPolicyType.esriSPTDuplicate
domain.MergePolicy=esriMergePolicyType.esriMPTDefaultValue
' Add the new domain to the workspace.
workspaceDomains.AddDomain(domain)
Opening and modifying domains
To modify a domain, first open it from its workspace. In the following code example, the IWorkspaceDomains.DomainByName is used to open a domain:
[C#] IWorkspaceDomains workspaceDomains=(IWorkspaceDomains)workspace;
IDomain requestedDomain=workspaceDomains.get_DomainByName(domainName);
[VB.NET] Dim workspaceDomains As IWorkspaceDomains=CType(workspace, IWorkspaceDomains)
Dim requestedDomain As IDomain=workspaceDomains.DomainByName(domainName)
The following method takes a domain and prints the valid values to the console:
[C#] Console.WriteLine("Valid values for domain '{0}':", domain.Name);
if (domain.Type == esriDomainType.esriDTCodedValue)
{
ICodedValueDomain codedValueDomain=(ICodedValueDomain)domain;
for (int i=0; i < codedValueDomain.CodeCount; i++)
{
// codedValueDomain.get_Value(i) returns the code that is stored in the geodatabase
// (for example, RES).
// codedValueDomain.get_Name(i) returns the name that is shown in the ArcMap
// inspector (for example, Residential).
Console.WriteLine("Code: {0} Name: {1}", codedValueDomain.get_Value(i),
codedValueDomain.get_Name(i));
}
}
else
{
IRangeDomain rangeDomain=(IRangeDomain)domain;
Console.WriteLine("Minimum Value: {0} Maximum Value: {1}", rangeDomain.MinValue,
rangeDomain.MaxValue);
}
[VB.NET] Console.WriteLine("Valid values for domain '{0}':", domain.Name)
If domain.Type=esriDomainType.esriDTCodedValue Then
Dim codedValueDomain As ICodedValueDomain=CType(domain, ICodedValueDomain)
For i As Integer=0 To codedValueDomain.CodeCount - 1
' codedValueDomain.Value(i) returns the code that is stored in the geodatabase
' (for example, RES).
' codedValueDomain.Name(i) returns the name that is shown in the ArcMap inspector
' (for example, Residential).
Console.WriteLine("Code: {0} Name: {1}", codedValueDomain.Value(i), codedValueDomain.Name(i))
Next i
Else
Dim rangeDomain As IRangeDomain=CType(domain, IRangeDomain)
Console.WriteLine("Minimum Value: {0} Maximum Value: {1}", rangeDomain.MinValue, rangeDomain.MaxValue)
End If
Once a domain has been opened, the range of an existing range domain can be modified and values can be added to and removed from a coded value domain using the same properties and methods that were used at creation time. However, calling these methods and properties does not automatically persist changes to the geodatabase. After a domain has been modified, call IWorkspaceDomains2.AlterDomain to persist the changes.
Starting at ArcGIS 10.1, domains can be renamed through the use of the IWorkspaceDomains3.AlterDomainWithName method.
See Also:
Creating subtypesAssigning domains to fields
To use the code in this topic, reference the following assemblies in your Visual Studio project. In the code files, you will need using (C#) or Imports (VB .NET) directives for the corresponding namespaces (given in parenthesis below if different from the assembly name):
- ESRI.ArcGIS.Geodatabase
- ESRI.ArcGIS.System (ESRI.ArcGIS.esriSystem)
Development licensing | Deployment licensing |
---|---|
ArcGIS Desktop Basic | ArcGIS Desktop Basic |
ArcGIS Desktop Standard | ArcGIS Desktop Standard |
ArcGIS Desktop Advanced | ArcGIS Desktop Advanced |
Engine Developer Kit | Engine |