In this topic
- About rendering dynamic map content
- Dynamic symbols
- Dynamic glyphs
- Dynamic glyphs group
- Dynamic display draw methods
- Dynamic layer
- Immediate vs. compiled drawing phases
- Compound marker and array marker
About rendering dynamic map content
The dynamic display is an active display, which means it has a "heart beat" that checks the display status and causes the display to redraw or repaint when needed.
The dynamic display renders the standard layers and graphics in the background, and has the ability to render dynamic map content with the use of dynamic layers and map drawings (standard layers and standard graphics will be referred to as standard layers).
The following are the ways to render dynamic map content:
- Dynamic layer - Have a custom layer implement IDynamicLayer and draw dynamic content from the IDynamicLayer.DrawDynamicLayer callback method. These layers show as a standard layer in the map's layer list and in the table of contents (TOC); therefore, the layers will be reordered between any other layers. This is useful for dynamic content that needs to be grouped together and treated as a layer, for example, tracks that can be reordered and uncluttered using a TOC or the layer's application programming interface (API).
- Dynamic map drawing - Listens to the IDynamicMapEvents, BeforeDynamicDraw, and AfterDynamicDraw events and draws dynamic content in those events. This dynamic content will be drawn before or after all the map's layers, respectively, and will not be visible in the map's layers list and TOC. This is useful for content that is not identified as a layer, for example, a compass or navigation data that needs to be rendered on top of all the map's layers.
IDynamicLayer.DrawDynamicLayer, IDynamicMapEvents, BeforeDynamicDraw, and AfterDynamicDraw events, will be referred to as dynamic draw callbacks.
Drawing dynamic content is done by using the dynamic display API and the Open Graphics Library (OpenGL) API. Before invoking dynamic draw callbacks, the dynamic map is setting up an active OpenGL rendering context, as well as passing in a reference to an active dynamic display with the method and event invocation. Therefore, in the dynamic draw callbacks (method and event handlers) context, the dynamic display API and the OpenGL API can be utilized to draw any dynamic content.
Dynamic symbols
The dynamic display object has the following active dynamic symbols:
- Dynamic marker symbol
- Dynamic line symbol
- Dynamic text symbol
- Dynamic fill symbol
The current state of these dynamic symbols is used to determine how the drawing renders when the dynamic display draw methods are called. Use IDynamicSymbolProperties to change the current state of the dynamic symbols.
The following are the types of methods and properties on IDynamicSymbolProperties:
- Generic - Takes an extra parameter, type of dynamic symbol, and changes the state of the passed in dynamic symbol type.
- Specific - Pertains to a dynamic symbol type; therefore, the names start with the type of dynamic symbol (for example, text leading is specific to dynamic text symbol).
The following table shows the properties of each dynamic symbol:
Property |
Generic or specific |
Dynamic text symbol |
Dynamic marker symbol |
Dynamic line symbol |
Dynamic fill symbol |
Dynamic glyph |
G |
+ |
+ |
+ |
+ |
Color |
G |
+ |
+ |
+ |
+ |
Scale (x,y) |
G |
+ |
+ |
+ |
+ |
Smooth |
G |
+ |
+ |
+ |
+ |
Offset (x,y) |
G |
+ |
+ |
+ |
+ |
Use reference scale |
G |
+ |
+ |
+ |
+ |
Heading |
G |
+ |
+ |
+ | |
Rotation alignment |
G |
+ |
+ |
+ | |
Text vertical alignment |
S |
+ |
|||
Text horizontal alignment |
S |
+ |
|||
Text leading |
S |
+ |
|||
Text character spacing |
S |
+ |
|||
Text word spacing |
S |
+ |
|||
Text right to left |
S |
+ |
|||
Text box horizontal alignment |
S |
+ |
|||
Text box use dynamic fill symbol |
S |
+ |
|||
Text box use dynamic line symbol |
S |
+ |
|||
Text box margins |
S |
+ |
|||
Line continue pattern |
S |
+ |
Dynamic glyphs
A dynamic glyph is a handle to a graphic resource. Use the DynamicGlyphFactory to create and delete dynamic glyphs. The following are the types of dynamic glyphs:
- Dynamic marker glyph
- Dynamic line glyph
- Dynamic text glyph
- Dynamic fill glyph
It is important to correctly manage dynamic glyphs to minimize the application memory usage. Incorrect management of dynamic glyphs leads to over consumption of resources, and can result in poor performance and an application crash. Minimize the creation of dynamic glyphs and reuse dynamic glyphs as much as possible. For example, create a dynamic glyph from a white symbol, set the dynamic symbol's glyph property to use the dynamic glyph, and use the dynamic symbol's color and scale properties to render the dynamic symbol (that uses one dynamic glyph) in different colors and sizes.
When a dynamic glyph is not in use, use IDynamicGlyphFactory.DeleteDynamicGlyph to release the dynamic glyph's resources.
Dynamic glyphs can be created from a file, symbol, glyphs group, or handle to a bitmap.
Dynamic glyphs group
A dynamic glyphs group is a container that holds multiple glyphs. It is composed of a bitmap and database that keeps information about the group and elements (glyphs and glyphs items) in the group. The image is a mosaic of sub-images of icons (marker glyphs), lines (line glyphs), and groups of characters (each group composes a text glyph).
The bitmap keeps color information (red, green, and blue [RGB], and alpha values) for each pixel. The bitmap can be loaded from the following two file images:
- One that holds the RGB values
- One that holds the alpha values or from a single file image that holds red, green, blue, and alpha (RGBA) values
The glyphs group database is loaded from an Extensible Markup Language (XML) file. This XML file holds general information about the group, specific location, and size for each of the items and elements of the group.
A new glyphs group can be loaded using IDynamicGlyphFactory.LoadDynamicGlyphsGroup. The method creates a glyphs group and returns an allocated glyphs group ID. The returned ID is a positive integer.
- GroupID 0 refers to rendering without using a graphical resource, which means simple lines, simple points, and so on.
- Glyphs group ID 1 is reserved as the default glyphs group (there is a default one that exists with the dynamic display) and supplies a default set of dynamic glyphs.
To replace the preloaded default group, delete group ID 1, then immediately create a group. The dynamic map assigns the new group ID 1 and uses it as the new default glyphs group.
Performance wise, it is preferable to create a glyph group with multiple glyphs and use the glyphs from the group, rather than creating glyphs from individual image files.
The dynamic glyphs factory is a singleton object; therefore, you can always cocreate and use it to create and delete dynamic glyphs.
Performance wise, it is preferable to create dynamic glyphs in the context of IDynamicLayer.DrawDynamicLayer in the esriDDPImmediate draw phase, or in the context of the IDynamicMapEvents.BeforeDynamicDraw or IDynamicMapEvents.AfterDynamicDraw methods, while casting the passed in IDynamicDisplay interface to IDynamicGlyphFactory.
When cocreating the DynamicGlyphFactory singleton in a .NET application, use the Activator class instead of using the new keyword. See the following code example:
[C#] Type t=Type.GetTypeFromProgID("esriCarto.DynamicGlyphFactory");
System.Object dynamicGlyphFactoryObject=Activator.CreateInstance(t);
IDynamicGlyphFactory2 dynamicGlyphFactory=dynamicGlyphFactoryObject as
IDynamicGlyphFactory2;
[VB.NET] Dim t As Type=Type.GetTypeFromProgID("esriCarto.DynamicGlyphFactory")
Dim dynamicGlyphFactoryObject As System.Object=Activator.CreateInstance(t)
Dim dynamicGlyphFactory As IDynamicGlyphFactory2=TryCast(dynamicGlyphFactoryObject, IDynamicGlyphFactory2)
For more information, see Interacting with singleton objects.
Dynamic display draw methods
Dynamic display draw methods can be used to draw marker, multiple markers, line, multiple lines, polyline, polygon, rectangle, text, and compound items that include a marker and text strings around it.
Each draw method gets a point location and comes in map coordinates or screen (pixel) coordinates. See the following:
- Map coordinates - Items that will be drawn using these methods and moves with the map when panning, rotating, and navigating by changing the visible bounds.
- Screen coordinates - Items that will be drawn using these methods, draws on the screen, and will not be affected by the map's navigation. The screen's origin is the lower left corner, while x values move to the right, and y values move to the top.
The following table shows the correlations of the dynamic display's draw methods on the current state of each dynamic symbol:
Draw method |
Dynamic marker symbol |
Dynamic text symbol |
Dynamic line symbol |
Dynamic fill symbol |
Marker |
+ |
|||
MultipleMarkers |
+ |
|||
Line |
+ |
|||
MultipleLines |
+ |
|||
Polyline |
+ |
|||
Polygon |
+ (Polygon's outline) |
+ Polygon's fill | ||
Rectangle |
+ (Rectangle's outline) |
+ (Rectangle's fill) | ||
Text |
+ |
+ (TextBox outline) |
+ (TextBox fill) | |
CompoundMarker* |
+ |
+ |
+ (TextBox outline) |
+ (TextBox fill) |
ArrayMarker |
+ |
+ |
+ (TextBox outline) |
+ (TextBox fill) |
When drawing markers and lines, if a dynamic glyph created from group ID 0 (or no dynamic glyph) is set to the corresponding dynamic symbol, the dynamic display renders simple points and simple lines.
Dynamic layer
A dynamic layer is a custom layer. At a minimum, each dynamic layer must implement ILayer, IDynamicLayer, and IGeoDataset.
Since a dynamic layer is a custom layer, implement the underlying data structure of the layer, as well as the drawing, selection, identification, and so on.
Since a dynamic layer is implementing IDynamicLayer and ILayer, it has the following drawing methods:
- IDynamicLayer.DrawDynamicLayer gets called when the dynamic map is enabled.
- ILayer.Draw gets called when the dynamic map is disabled.
That way, if your layer must draw both methods in standard drawing and dynamic mode, you can implement both methods. When implementing IDynamicLayer.DrawDynamicLayer, use the dynamic display API and the OpenGL API. When implementing ILayer.Draw, use the standard drawing commands (for example, ISymbol.Draw) and Windows Graphic Device Interface (GDI/GDI+) API.
Immediate versus compiled drawing phases
Dynamic layers can be drawn in two different ways and have two drawing phases - immediate and compiled. In each phase, when a new set of draw commands need to be generated, the DrawDynamicLayer method needs to iterate through the layer's underlying data structure and generate the corresponding draw commands for each item. In the compiled phase, the draw commands are compiled into an internal dedicated display list of the dynamic layer.
In every dynamic cycle, the dynamic map checks each dynamic layer's state. If there is a dynamic layer that needs to be redrawn (the dynamic layer needs to regenerate a new set of draw commands), the dynamic map calls the dynamic layer's DrawDynamicLayer method, with the corresponding draw phase, and the dynamic layer generates a new set of draw commands. If there is at least one dynamic layer that needs to regenerate its drawing commands, the entire dynamic display needs to get repainted.
A dynamic layer needs to regenerate its drawing commands (get redrawn), according to the draw phase it is drawing in. See the following:
- Immediate phase - If it reports that its immediate phase is dirty, the draw commands execute immediately.
- Compiled phase - If it reports that its compiled phase is dirty and its recompile rate interval has elapsed, the display list has expired and the dynamic layer's draw commands recompiled into the display list. The display list will be reused for rendering every dynamic cycle that the dynamic display needs to get repainted - that is, if the dynamic layer is not reporting that the display list has expired; therefore, the display list does not need to get recompiled.
With every dynamic cycle that the dynamic display needs to get repainted, the dynamic map calls all the dynamic layers' DrawDynamicLayer with the immediate phase (reuses the display lists of the dynamic layers with the compiled phase).
The difference between the phases is when the dynamic display needs to repaint, the dynamic map can reuse the display lists generated by the dynamic layers that draw in the compiled phase, but regenerates the drawing commands for the dynamic layers that draw in the immediate phase.
The immediate draw phase is called every cycle the display needs to be repainted, but the compiled draw phase is called only when the dynamic map is notified that the display list has expired (when the DynamicRecompileRate interval elapses and the DynamicLayerDirty dirty flag for the esriDDCompiled phase equals true).
Draw dynamic layers in one of the draw phases, but not in both.
Use the compiled phase since it minimizes the central processing unit (CPU) usage and the bus traffic to the graphic card by minimizing the frequency in which the dynamic layer's draw commands regenerate (layer items are iterated and draw commands are called). For example, to use the compiled phase, see the RSS weather 3D layer sample. If layer items are updated frequently and the compiled phase display list needs to be recompiled frequently, render the layer items in the immediate phase instead of in the compiled phase. For more information about using the immediate phase, see the Dynamic display layer sample.
Another advantage of the compiled phase versus the immediate phase is that when using the immediate phase, every change in the layers (or any reason that causes a repaint of the display) causes all layers that use the immediate phase to regenerate their drawing code, even when there was no change.
In .NET, there is a base class for a dynamic layer that implements most of the necessary functionality of a dynamic layer. When using the base class, the dynamic layer needs to inherit from the ESRI.ArcGIS.ADF.BaseClasses.BaseDynamicLayer base class and override the DrawDynamicLayer method (which is defined as an abstract method). For more information, see the Dynamic logo sample.
Compound marker and array marker
It is frequently required to draw a marker with surrounding text strings by using IDynamicDisplay.DrawMarker and IDynamicDisplayDrawText. It is easier to use IDynamicCompoundMarker2, which provides helper methods that draw a marker with surrounding text strings.
IDynamicCompoundMarker2 also provides methods and properties to control the distance, such as the text from the marker, spacing between the text strings, and so on. DrawCompoundMarkerN draws a marker with a combination of 1, 2, 4, 8, or 10 surrounding text strings. These methods only use a location in map coordinates.
The following illustration shows the layout of the DrawCompoundMarker10 method:
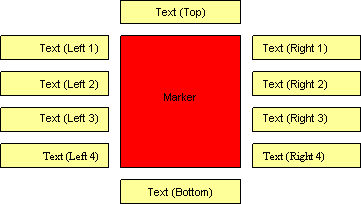
The DrawArrayMarker methods draw a marker with arrays of text strings in each of the following five regions around the marker:
- Center
- Left
- Right
- Top
- Bottom
These methods have a location in the map coordinates and in screen coordinates (pixels).
The following illustration shows the layout of the DrawArrayMarker method with the input of the following array sizes:
- Center[4]
- Left[5]
- Right[5]
- Top[2]
- Bottom[3]
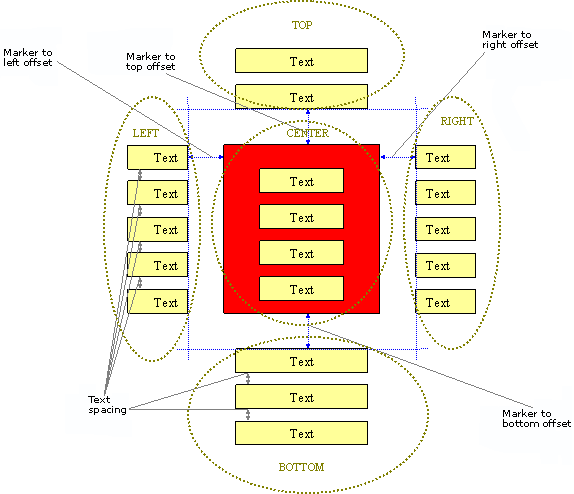
See Also:
Dynamic displayHow dynamic display works
Limitations for dynamic display
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |
ArcGIS for Desktop Basic | |
ArcGIS for Desktop Standard | |
ArcGIS for Desktop Advanced |