- Engine with 3D Analyst
- Engine with Spatial Analyst
- ArcGIS for Desktop Basic with 3D Analyst
- ArcGIS for Desktop Basic with Spatial Analyst
- ArcGIS for Desktop Standard with 3D Analyst
- ArcGIS for Desktop Standard with Spatial Analyst
- ArcGIS for Desktop Advanced with 3D Analyst
- ArcGIS for Desktop Advanced with Spatial Analyst
- Server with 3D Analyst
- Server with Spatial Analyst
Additional library information: Contents, Object Model Diagram
The GeoAnalyst library is a shared object library available to users who have an ArcGIS 3D Analyst or Spatial Analyst extension license. The library provides an easy to customize environment and includes a set of tools for application development. As a shared library, it builds on the raster-related objects of the DataSourcesRaster library by providing objects that are used to access, analyze, manage, convert, and visualize raster data.
The SpatialAnalyst library also includes objects used to process raster datasets; however, those objects are available only to users who have an ArcGIS Spatial extension license. As a shared library, GeoAnalyst includes features that complement the SpatialAnalyst library such as the unified data access and interaction independent format, a high level non-proprietary language.
To increase system performance, raster outputs from some methods are not computed until they are used. This deferred evaluation of output datasets can result in outputs never being calculated if they are never used. Any use of the output, such as creating a layer, examining the height and width of the output, or making the dataset permanent, will trigger evaluation of the expression to create the dataset.
There are many objects in the GeoAnalyst object model and hundreds of methods to perform spatial modeling. Even though there are many objects and methods, there are five main steps when implementing any GeoAnalyst operation, which are as follows:
- Obtaining a 3D Analyst or Spatial Analyst extension license
- Setting the analysis environment
- Accessing the input data
- Performing an operation
- Using the output
See the following sections for more information about this namespace:
Enabling the 3D Analyst or Spatial Analyst license
To access the ArcGIS GeoAnalyst objects, you must obtain a 3D Analyst or Spatial Analyst license. You can call for a license prior to accessing each GeoAnalyst object, once in a single subroutine, or globally in the main routine in a program. Because you only need to call the single subroutine once and because the license is automatically enabled if you call it globally, either of these approaches is a preferred method for obtaining a license as shown in the following code example:
[VB.NET] Public Function CheckOutSpatialAnalystLicense() As Boolean
Dim extManagerAdmin As IExtensionManagerAdmin=New ExtensionManager
Dim uid As UID=New UID
uid.Value="esriGeoAnalyst.SAExtension.1"
Dim obj As New Object
extManagerAdmin.AddExtension(uid, obj)
Dim extManager As IExtensionManager=TryCast(extManagerAdmin, IExtensionManager)
Dim extConfig As IExtensionConfig
extConfig=TryCast(extManager.FindExtension(uid), IExtensionConfig)
extConfig.State=esriExtensionState.esriESEnabled
CheckOutSpatialAnalystLicense=(extConfig.State=esriExtensionState.esriESEnabled)
End Function
[C#] public bool CheckOutSpatialAnalystLicense()
{
IExtensionManagerAdmin extManagerAdmin=new ExtensionManagerClass();
UID uid=new UIDClass();
uid.Value="esriGeoAnalyst.SAExtension.1";
object obj=new object();
extManagerAdmin.AddExtension(uid, ref obj);
IExtensionManager extManager=extManagerAdmin as IExtensionManager;
IExtensionConfig extConfig=null;
extConfig=extManager.FindExtension(uid)as IExtensionConfig;
extConfig.State=esriExtensionState.esriESEnabled;
return (extConfig.State == esriExtensionState.esriESEnabled);
}
GeoAnalyst objects
The GeoAnalyst library objects are detailed in the following diagram:
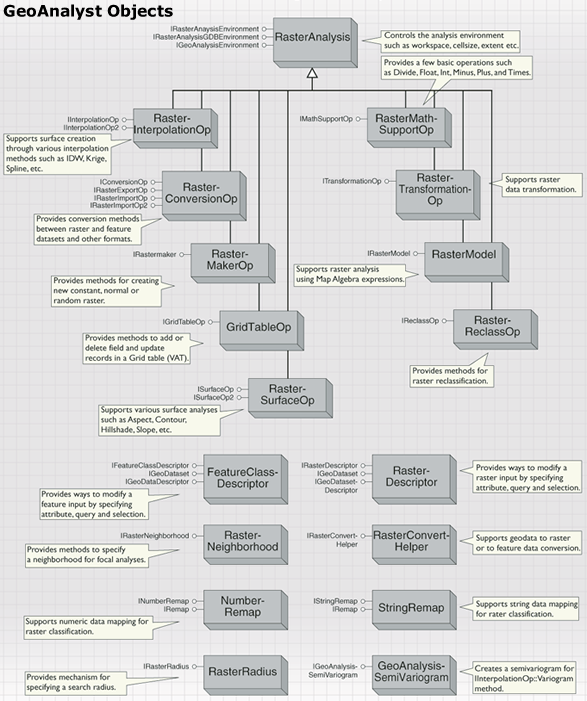
RasterAnalysis
The analysis environment (through the RasterAnalysis object) controls a number of primary properties, such as the cell size, extent, mask, spatial reference, and workspace, when performing analysis. These settings have no affect on the original data; however, the extent and mask do affect the locations where the operation will occur. The extent defines the area on which the operation will be performed. The mask defines the cell locations within the extent on which to perform the operation. The specified cell size controls the resolution of the output.
When first created, the new operator object obtains its parameters from the settings in the RasterAnalysis object. Each operator object can have its own analysis environment settings. You can set the environment parameters individually for each operator object. The following code example demonstrates how to change the default analysis environment settings that subsequent newly created operator objects will inherit. The analysis environment is altered, then set back to the default setting.
[VB.NET] Public Function SetNewDefaultEnvironment(ByVal extentEnv As IEnvelope, ByVal cellSize As Double, _
ByVal mask As IGeoDataset, ByVal workspace As IWorkspace, ByVal prj As ISpatialReference) _
As IRasterAnalysisEnvironment
Try
' Create a new RasterAnalysis object and set it as a new default setting.
Dim rasterAnalysisEnv As IRasterAnalysisEnvironment=New RasterAnalysis
' Set the new default extent.
If Not extentEnv Is Nothing Then
Dim object_extentEnv As Object=TryCast(extentEnv, Object)
rasterAnalysisEnv.SetExtent(esriRasterEnvSettingEnum.esriRasterEnvValue, object_extentEnv)
End If
' Set the new default cellsize.
If cellSize > 0 Then
Dim object_cellSize As Object=TryCast(cellSize, Object)
rasterAnalysisEnv.SetCellSize(esriRasterEnvSettingEnum.esriRasterEnvValue, object_cellSize)
End If
' Set the new default mask for analysis.
If Not mask Is Nothing Then
rasterAnalysisEnv.Mask=mask
End If
' Set the new default output workspace.
If Not workspace Is Nothing Then
rasterAnalysisEnv.OutWorkspace=workspace
End If
' Set the new default output spatial reference.
If Not prj Is Nothing Then
rasterAnalysisEnv.OutSpatialReference=prj
End If
' Set it as the default setting.
rasterAnalysisEnv.SetAsNewDefaultEnvironment()
' Return a reference to the default environment setting.
SetNewDefaultEnvironment=rasterAnalysisEnv
Exit Function
Catch ex As Exception
SetNewDefaultEnvironment=Nothing
End Try
End Function
[C#] public IRasterAnalysisEnvironment SetNewDefaultEnvironment(IEnvelope extentEnv,
double cellSize, IGeoDataset mask, IWorkspace workspace, ISpatialReference prj)
{
IRasterAnalysisEnvironment tempSetNewDefaultEnvironment=null;
try
{
// Create a new RasterAnalysis object and set it as a new default setting.
IRasterAnalysisEnvironment rasterAnalysisEnv=new RasterAnalysis();
// Set the new default extent.
if (extentEnv != null)
{
object object_extentEnv=extentEnv as object;
object object_Missing=Type.Missing;
rasterAnalysisEnv.SetExtent(esriRasterEnvSettingEnum.esriRasterEnvValue,
ref object_extentEnv, ref object_Missing);
}
// Set the new default cellsize.
if (cellSize > 0)
{
object object_cellSize=cellSize as object;
rasterAnalysisEnv.SetCellSize
(esriRasterEnvSettingEnum.esriRasterEnvValue, ref object_cellSize);
}
// Set the new default mask for analysis.
if (mask != null)
{
rasterAnalysisEnv.Mask=mask;
}
// Set the new default output workspace.
if (workspace != null)
{
rasterAnalysisEnv.OutWorkspace=workspace;
}
// Set the new default output spatial reference.
if (prj != null)
{
rasterAnalysisEnv.OutSpatialReference=prj;
}
// Set it as the default setting.
rasterAnalysisEnv.SetAsNewDefaultEnvironment();
// Return a reference to the default environment setting.
return rasterAnalysisEnv;
}
catch (Exception ex)
{
tempSetNewDefaultEnvironment=null;
}
return tempSetNewDefaultEnvironment;
}
RasterDescriptor
The RasterDescriptor object provides access to members that control the raster descriptor. It provides a way of using a field other than the value field in the raster table to perform queries. It also allows you to perform a query, using a where clause, then to use this subset to perform the analysis - for example, in the RasterReclassOp coclass. A selection set can also be created for use in further analysis.
FeatureClassDescriptor
The FeatureClassDescriptor object provides access to members that control the FeatureClass descriptor and also provides a way of using values of a specified field or fields. For example, FeatureClassDescriptor can be used with the members of IInterpolationOp and IDistanceOp.
RasterConversionOp
The RasterConversionOp object provides a means to convert between raster and feature data. The different interfaces have the conversion operations grouped according to similar processes.
In the IConversionOp interface, the RasterDataToLineFeatureData, RasterDataToPointFeatureData, and RasterDataToPolygonFeatureData methods convert raster data to line, point, and polygon feature data respectively. The ToFeatureData method allows you to convert raster data to feature data (FeatureClass or FeatureDataset), and you can specify the geometry. The ToRasterDataset method converts feature data to a raster format.
In the IRasterExportOp interface, the ExportToASCII and ExportToFloat methods convert raster datasets to a flat file of ASCII or binary floating-point files, respectively. The ImportFromASCII and ImportFromFLOAT methods import data from these flat files and create new rasters. The ImportFromUSGSDEM method in the IRasterImportOp interface converts a digital elevation model (DEM) in United States Geological Survey (USGS) format to a raster. The same method in the IRasterImportOp2 interface has an additional parameter that specifies the number of ground x, y units in one surface z unit.
RasterTransformationOp
The RasterTransformationOp object provides a mechanism to transform raster data. The ITransformationOp interface provides access to the following methods to transform the dataset:
- Clip - Cuts out a rectangular portion of a raster.
- Flip - Flips the raster along the horizontal axis.
- Mirror - Mirrors the raster along the vertical axis.
- Mosaic - Creates one raster from multiple input rasters using a user-selectable method to deal with overlapping areas of the neighboring rasters.
- ProjectFast - Projects a raster to a new spatial reference using the esriGeoAnalysisResampleEnum enumerator, which allows you to pick the nearest, bilinear, or cubic resampling technique.
- Resample - Resamples a raster to a new cell size.
- ReScale - Scales a raster by the specified x and y scale factors.
- Rotate - Rotates a raster around the specified pivot point by an angle specified in degrees.
- Shift - Shifts a raster in the x,y direction by specifying the respective distances.
- Warp - Transforms or rubbersheets a raster along a set of links using a polynomial transformation.
RasterMakerOp
The RasterMakerOp object can be used to create new raster datasets. The IRasterMakerOp interface supports the following methods for creating new datasets:
- MakeConstant - Creates a dataset that consists of a single integer or floating point value.
- MakeNormal - Creates a raster of random values from a normal distribution with a mean of 0 and a standard deviation of 1.
- MakeRandom - Creates a raster that consists of random numbers between 0 and 1 with the choice of specifying an optional seed value
RasterMathSupportOp
The RasterMathSupportOp object provides a few basic operations and is shared by the ArcGIS Spatial Analyst and 3D Analyst extensions. For example, RasterMathSupportOp can be used to change z units of an elevation raster in the 3D Analyst environment. The IRasterMathOps interface, however, has the complete list of methods available to users of ArcGIS Spatial Analyst.
NumberRemap
The NumberRemap object can assist in operations involving the reclassification of numerical data. A single value can be mapped onto a range and vise versa. A range can be remapped onto another range by using the methods in the INumberRemap interface. The NumberRemap object can be useful for classifications, which can then lead to vectorization and subsequent vector-based overlay analysis. For example, the IRemap interface allows access to the ReclassByRemap method on the RasterReclassOp object.
StringRemap
The StringRemap object allows you to perform operations that control the reclassification of string data. The StringRemap object can be used, for example, in a suitability study to assign weights to a raster.
RasterReclassOp
The RasterReclassOp object is used to perform the reclass operation. RasterReclassOp can also be used for classification, which can then lead to vectorization and subsequent vector-based overlay analysis.
The IReclassOp interface supports the following reclass operation methods:
- Lookup - Creates a new raster by looking up values in another field in the table of the input raster.
- Reclass - Reclassifies, or changes, the values in a raster.
- ReclassByASCIIFile - Reclassifies the value of the input cells of a raster by using an ASCII remap file.
- ReclassByRemap - Reclassifies the values of the input cells of a raster by using a remap that is built programmatically.
- Slice - "Slices", or changes, a range of values of the input cells by using the enumerators listed in esriGeoAnalysisSliceEnum, which allow you to choose between an equal area or an equal interval method.
RasterRadius
The RasterRadius object defines a mechanism to control the radius used to determine a surface, for example, through interpolation. It is primarily used to define the number of points via a fixed or variable radius. It can, for example, be used in the IDW method on RasterInterpolationOp to specify either the fixed or the variable number of points to consider when estimating a value at a location.
RasterNeighborhood
Focal functions compute an output raster where the output value at each location is a function of the input cells in a specified neighborhood of the location. The IRasterNeighborhood interface contains the following methods that provide the different neighborhood options:
- SetAnnulus - Provides a means for defining the region lying between two concentric circles to be used as a neighborhood.
- SetDefault - Creates a 3 by 3 neighborhood.
- SetIrregular - Sets a neighborhood object, each of whose entries can be turned on or off.
- SetWeight - Sets a neighborhood object each of whose entries can be assigned a weight. It can be used in the PointDensity method associated with the RasterNeighborhood object.
RasterInterpolationOp
The IInterpolationOp interface gives you access to the following methods:
- IDW - Performs an inverse distance weighted interpolation on a point feature data set.
- Krige - Interpolates a surface from a set of points using kriging.
- Spline - Interpolates a surface from a set of points using a minimum curvature spline technique.
- Trend - Performs a trend interpolation on a point dataset.
- Variogram - Similar to Krige except that it allows you to specify additional kriging parameters pertaining to the semivariogram. This is done through the GeoAnalysisSemiVariogram coclass.
The IInterpolationOp2 interface allows access to two additional interpolation methods. The NaturalNeighbor method creates a surface from points using a natural neighbor interpolation technique. The TopoToRasterByFile method allows you to generate a hydrologically correct raster of elevation values from point, line, and polygon datasets.
The IRasterRadius interface on the RasterRadius object defines how the points used in the interpolation method are selected.
The IInterpolationOp3 interface contains the TrendWithRms method that outputs the goodness-of-fit statistics of the trend surface, with an optional root mean square (rms) file, to a text file.
GeoAnalysisSemiVariogram
The GeoAnalysisSemiVariogram object is a mechanism to create a semivariogram object that can be used in the Variogram method in the IInterpolationOp interface. The DefineVariogram method is used to initialize the semivariogram parameters.
RasterConvertHelper
The RasterConvertHelper object assists when converting geodatasets to rasters or feature classes. The methods in the IRasterConvertHelper interface do not require you to specify the workspace or the name and are useful for operations where temporary output is required.
RasterSurfaceOp
RasterSurfaceOp is the raster surface operation object. The ISurfaceOp and ISurfaceOp2 interfaces contain the following methods that control the generation of a raster surface:
- Aspect - Identifies the direction of flow and maximum rate of change in z value from each cell.
- Contour - Creates contours or isolines from a raster surface.
- ContourAsPolyline - Creates a contour or isoline that passes through a specified point on a surface.
- ContourList - Creates contours or isolines based on a list of contour values.
- ContoursAsPolylines - Creates contours or isolines that pass through specified points on a surface.
- Curvature - Calculates the curvature of a surface at each cell center. From an applied viewpoint, output of the Curvature method can be used to describe the physical characteristics of a drainage basin in an effort to understand erosion and runoff processes. The profile curvature affects the acceleration and deceleration of flow, thereby influencing erosion and deposition. The planform curvature influences convergence and divergence of flow.
- CutFill - Creates output with volume information describing surface changes after a cut-and-fill operation.
- HillShade - Creates a shaded relief raster from a raster by considering the illumination angle and shadows.
- Slope - Identifies the rate of maximum change in z value from each cell and affects the overall rate of movement downslope.
- Visibility - Performs visibility analysis on a raster by determining how many observation points can be seen from each cell location of the input raster, or which cell locations can be seen by each observation point.
The Visibility method on the ISurfaceOp2 interface has two additional parameters: the number of ground x, y units in one surface Z unit and a coefficient of the refraction of visible light in air.
RasterModel
The RasterModel object is used to perform raster analysis using map algebra expressions and can include non-raster input/output formats (feature data, tables, and so on). RasterModel also allows multiple lines of GRID syntax.
GridTableOp
The GridTableOp object is used to perform operations on the Table object associated with a GRID dataset. The IGridTableOp interface offers the AddField method to add a Field object to the dataset and the DeleteField method to delete a Field object from the dataset. A Field object can also be updated based on a query filter with the Update method.