Creating a multipart polyline
This example uses an existing polyline to define a new, multipart polyline. In the following illustration, the input polyline is shown in the background, and the new polyline is shown in dark green with its vertices marked. Each part of the new polyline is a single segment, normal to a segment from the original polyline, incident at its midpoint, and one-third of its length.
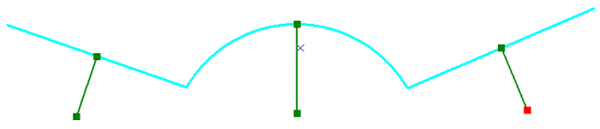
See the following code example:
[C#] public IPolyline ConstructMultiPartPolyline(IPolyline inputPolyline)
{
IGeometry outGeometry=new PolylineClass();
//Always associate new, top-level geometries with an appropriate spatial reference.
outGeometry.SpatialReference=inputPolyline.SpatialReference;
IGeometryCollection geometryCollection=outGeometry as IGeometryCollection;
ISegmentCollection segmentCollection=inputPolyline as ISegmentCollection;
//Iterate over existing polyline segments using a segment enumerator.
IEnumSegment segments=segmentCollection.EnumSegments;
ISegment currentSegment;
int partIndex=0;
;
int segmentIndex=0;
;
segments.Next(out currentSegment, ref partIndex, ref segmentIndex);
while (currentSegment != null)
{
ILine normal=new LineClass();
//Geometry methods with _Query_ in their name expect to modify existing geometries.
//In this case, the QueryNormal method modifies an existing line
//segment (normal) to be the normal vector to
//currentSegment at the specified location along currentSegment.
currentSegment.QueryNormal(esriSegmentExtension.esriNoExtension, 0.5, true,
currentSegment.Length / 3, normal);
//Since each normal vector is not connected to others, create a new path for each one.
ISegmentCollection newPath=new PathClass();
object missing=Type.Missing;
newPath.AddSegment(normal as ISegment, ref missing, ref missing);
//The spatial reference associated with geometryCollection will be assigned to all incoming
//paths and segments.
geometryCollection.AddGeometry(newPath as IGeometry, ref missing, ref
missing);
segments.Next(out currentSegment, ref partIndex, ref segmentIndex);
}
//The geometryCollection now contains the new, multipart polyline.
return geometryCollection as IPolyline;
}
[VB.NET] Private Function ConstructMulltiPartPolyline(ByVal inputPolyline As IPolyline) As IPolyline
Dim outGeometry As IGeometry=New PolylineClass()
Dim geometryCollection As IGeometryCollection=CType(outGeometry, IGeometryCollection)
Dim segmentCollection As ISegmentCollection=CType(inputPolyline, ISegmentCollection)
'Iterate over existing polyline segments using a segment enumerator.
Dim segments As IEnumSegment=segmentCollection.EnumSegments
Dim currentSegment As ISegment
Dim partIndex As Integer=0
Dim segmentIndex As Integer=0
segments.Next(currentSegment, partIndex, segmentIndex)
While Not currentSegment Is Nothing
Dim Normal As ILine=New LineClass()
'Geometry methods with _Query_ in their name expect to modify existing geometries.
'In this case, the QueryNormal method modifies an existing line
'segment (normal) to be the normal vector to
'currentSegment at the specified location along currentSegment.
currentSegment.QueryNormal(esriSegmentExtension.esriNoExtension, 0.5, True, currentSegment.Length / 3, Normal)
'Since each normal vector is not connected to others, create a new path for each one.
Dim newPath As ISegmentCollection=New PathClass()
Dim missing As Object=Type.Missing
newPath.AddSegment(Normal, missing, missing)
'The spatial reference associated with geometryCollection will be assigned to all incoming
'paths and segments.
geometryCollection.AddGeometry(newPath, missing, missing)
segments.Next(currentSegment, partIndex, segmentIndex)
End While
'The geometryCollection now contains the new, multipart polyline.
Return geometryCollection
End Function
To use the code in this topic, reference the following assemblies in your Visual Studio project. In the code files, you will need using (C#) or Imports (VB .NET) directives for the corresponding namespaces (given in parenthesis below if different from the assembly name):
Development licensing | Deployment licensing |
---|---|
ArcGIS Desktop Basic | ArcGIS Desktop Basic |
ArcGIS Desktop Standard | ArcGIS Desktop Standard |
ArcGIS Desktop Advanced | ArcGIS Desktop Advanced |
Engine Developer Kit | Engine |