In this topic
Casting jumps from one interface to another interface on an object created within a class. You can only cast between specific interfaces on the declared object. To see available interfaces to cast between, view the interfaces area of the class pages in the ArcObjects namespace reference. There are several types of casting that can be utilized in .NET coding. For more information on the different casting styles, see Casting between interfaces.
The following screen shot shows the ArcObjects namespace reference page for EnvelopeClass Class. This page has many interfaces that implement EnvelopeClass; therefore, when you create an EnvelopeClass object, cast (jump) to any available interfaces to access the properties, methods, events, and so on. Available interfaces on the EnvelopeClass page include - IArea, IClone (System), IEnvelope, and so on.
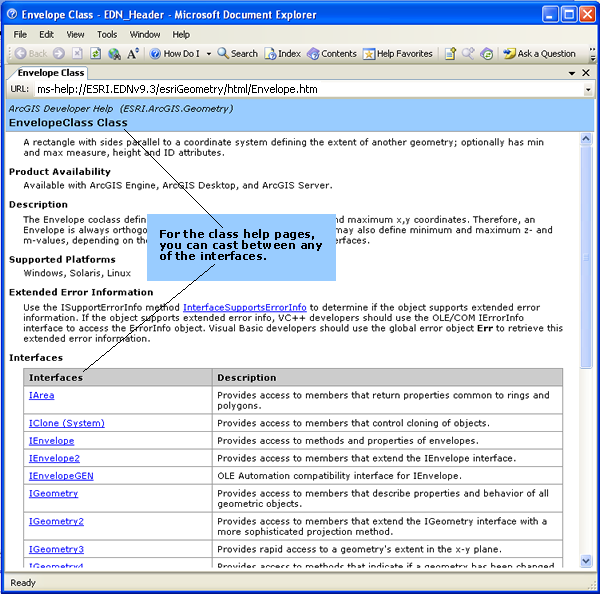
Click any interface link on the class help page to view the ArcObjects namespace reference page that describes that interface's member functionality. The following screen shot shows the page for IArea after you clicked the IArea link on the previous EnvelopeClass page:
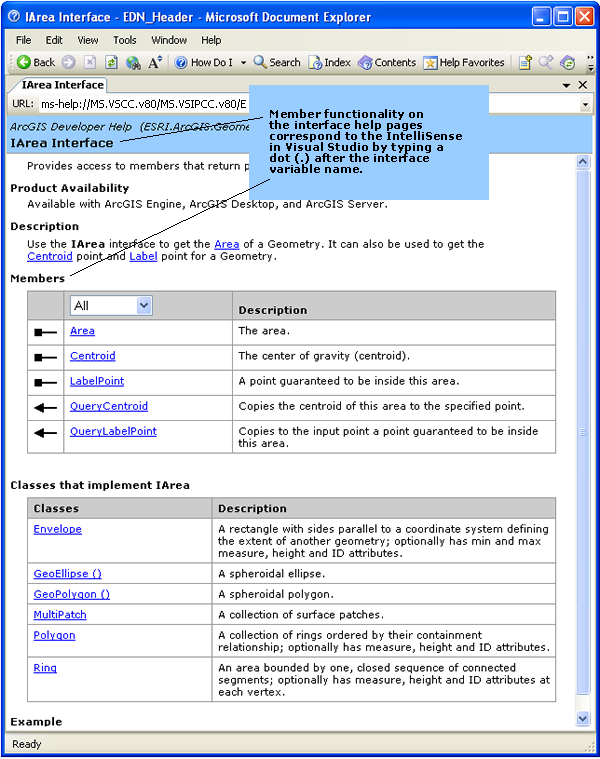
When you have instantiated an ArcObjects based variable, Visual Studio's IntelliSense context menu automatically populates with the available members for that variable. The IntelliSense context menu appears when you type a dot (.) after the variable name.
The following screen shot shows using IArea to gain access to a new EnvelopeClass object. The member information corresponds to what is available on the interface help page in the IArea ArcObjects namespace reference.
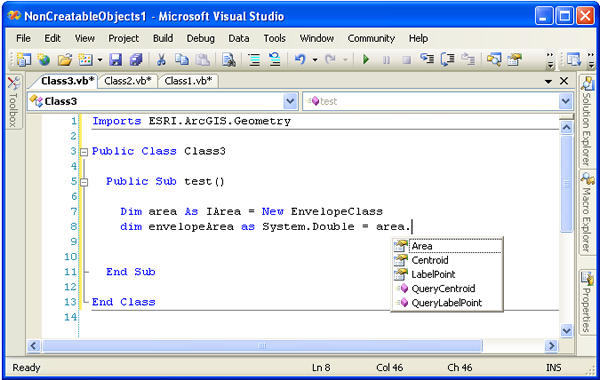
Although the same interface can exist on multiple classes, you cannot cast across different objects (that is, variables). For example, the EnvelopeClass and PolygonClass have IArea interfaces, but you cannot cast across the two objects. Attempting to do so results in an InvalidCastException runtime error as shown in the following EnvelopeClass, Polygon Class, and Visual Studio screen shots:
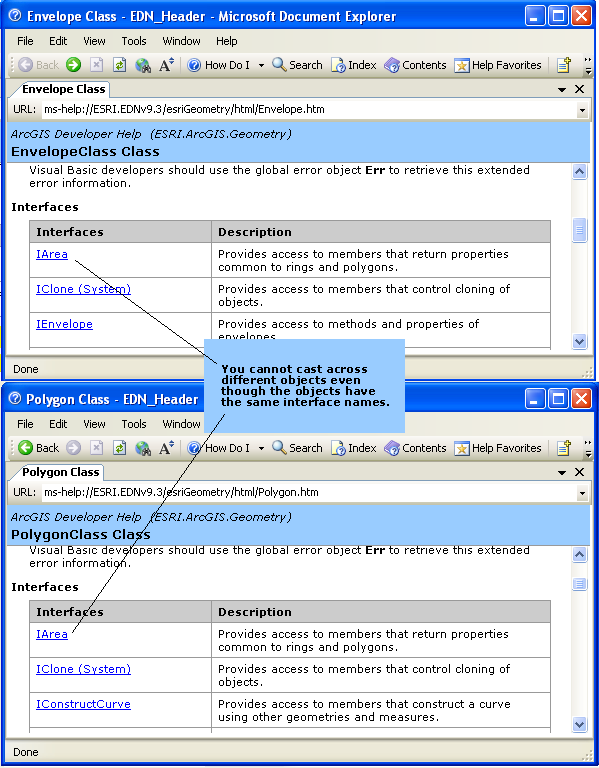
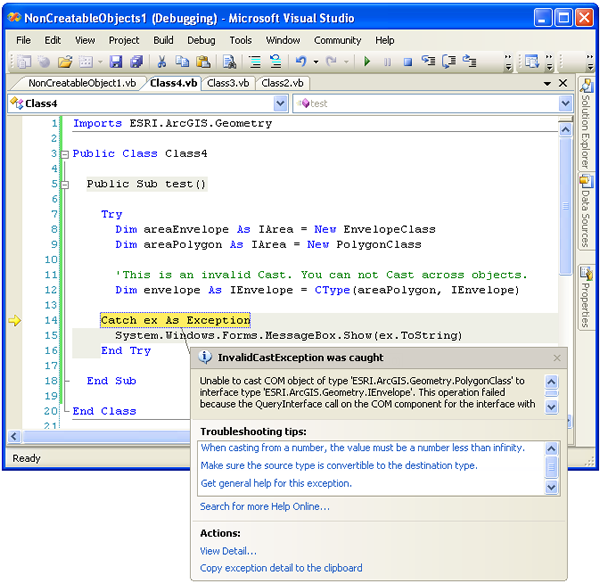
If you need to cast across objects, use the member (that is, property or method) of one object to get the interface into another object. See the following code example that shows one way to do this:
[VB.NET] Public Class Class1
Public Sub Test()
' Create an empty polygon object.
Dim areaPolygon As IArea=New PolygonClass
' Cast to the IGeometry interface of the polygon object.
Dim geometryPolygon As IGeometry=CType(areaPolygon, IGeometry)
' Use the .Envelope property on the IGeometry interface of the
' polygon object to get an envelope object.
Dim envelope As IEnvelope=geometryPolygon.Envelope
' Test to make sure you have an envelope object.
If TypeOf envelope Is Envelope Then
' The polygon object and resulting envelope are empty.
If envelope.IsEmpty Then
System.Windows.Forms.MessageBox.Show("The envelope is empty.")
End If
End If
End Sub
End Class
[C#] class temp
{
public void test()
{
// Create an empty polygon object.
IArea areaPolygon=new PolygonClass();
// Cast to the IGeometry interface of the polygon object.
IGeometry geometryPolygon=(IGeometry)areaPolygon;
// Use the .Envelope property on the IGeometry interface of the
// polygon object to get an envelope object.
IEnvelope envelope=geometryPolygon.Envelope;
// Test to make sure you have an envelope object.
if (envelope is Envelope)
{
// The polygon object and resulting envelope are empty.
if (envelope.IsEmpty)
{
System.Windows.Forms.MessageBox.Show("The envelope is empty.");
}
}
}
}
See Also:
Understanding the ArcObjects namespace referenceCasting between interfaces
To use the code in this topic, reference the following assemblies in your Visual Studio project. In the code files, you will need using (C#) or Imports (VB .NET) directives for the corresponding namespaces (given in parenthesis below if different from the assembly name):
Development licensing | Deployment licensing |
---|---|
ArcGIS Desktop Basic | ArcGIS Desktop Basic |
ArcGIS Desktop Standard | ArcGIS Desktop Standard |
ArcGIS Desktop Advanced | ArcGIS Desktop Advanced |
Engine Developer Kit | Engine |