In this topic
- About adding and working with connectivity rules in a geometric network
- Creating edge-junction connectivity rules
- Creating edge-edge connectivity rules
- Accessing connectivity rules
About adding and working with connectivity rules in a geometric network
In most utility and facility networks, not every edge type should be able to connect to every junction type. Similarly, not all edge types should connect to all other edge types through all junction types. Some examples of this include the following:
- In a water network, a hydrant can connect to a hydrant lateral but not to a service lateral.
- In a water network, a 10-inch transmission main can only connect to an 8-inch transmission main through a reducer.
- In a waste water network, a catch basin lead should only connect to other catch basin leads through a manhole.
- In an electric network, a bus bar can connect to other bus bars through an open point or transformer, but not a generator.
While the geometric network allows any edge to connect to any junction, network connectivity rules can be used to help constrain the type of network features that can be connected to each other and the number of features of any particular type that can be connected to features of another type. To this end, the geometric network supports two types of connectivity rules - edge-junction rules and edge-edge rules. As with other rules in the geometric network, connectivity rules can be specified at the class and subtype level.
By establishing these rules, the integrity of the network data can be maintained. At any time, features can be selectively validated and reports can be generated to indicate which features in the network are invalid - that is, violating one of the connectivity rules.
Connectivity rules are only applicable to geometric networks and are not applicable to network datasets.
Creating edge-junction connectivity rules
An edge - junction connectivity rule establishes the possible valid network connections that might exist between a edge and junction pair. Edge - junction rules are created with the JunctionConnectivityRule class and the IJunctionConnectivityRule2 interface.
EdgeClassID and JunctionClassID
The EdgeClassID and JunctionClassID properties correspond to the class IDs for the edge and junction classes. These properties are required.
EdgeSubtypeCode and JunctionSubtypeCode
Since edge-junction rules are defined at the class and subtype level, the class and subtype of the edge and junction must be specified. If subtypes are not specified for either class, use the subtype code of zero. Neglecting to specify the EdgeSubtypeCode or JunctionSubtypeCode values result in an invalid connectivity rule. These properties are required.
Cardinality
Connectivity rules can also govern the cardinalities of the connectivity relationships - the number of edges that can connect to a junction or the number of junctions that can connect to an edge - for example, in a water network with an edge-junction rule, where a hydrant lateral can connect to one, and only one, hydrant feature.
The cardinality of a junction is the number of edge features to which it is connected, with one exception. If a junction is only connected at mid-span to a complex edge feature, the cardinality of that junction is 2. In this instance, the cardinality of the junction is based on the number of connected edge elements, not the number of edge features, for example, for this junction to be considered valid, its cardinality should be 0 to 2.
The minimum and maximum cardinality based properties for the edge and junction classes are not required.
Default junction
Edge - junction connectivity rules can optionally have default junctions associated with them. When the DefaultJunction property is set, the geometric network creates a feature of the type specified as the default junction when edge features are created in a network. For example, with an edge-junction rule between a storm pipe and a manhole, where the manhole is specified as the default junction - when a new storm pipe is created, the geometric network automatically creates a manhole feature and connects to the end point of the storm pipe as shown in the following illustration:
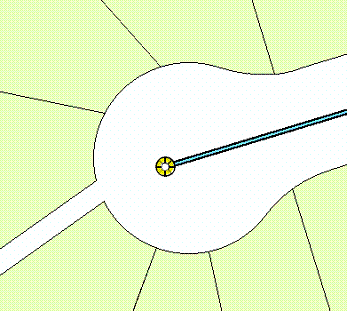
Specifying edge-junction rules with default junctions can simplify the creation of network features by automatically adding the relevant junctions.
If the business rules that drive the creation of connectivity rules do not have a clear default junction class or type, set the Orphan Junction feature class as the default junction class.
While the IJunctionConnectivityRule interface exists, it has been superseded by the IJunctionConnectivityRule2 interface.
The following code example demonstrates how to add an edge-junction connectivity rule to a geometric network:
[C#] public void AddEdgeJunctionConnectivityRule(IGeometricNetwork geometricNetwork,
IFeatureClass edgeFeatureClass, int edgeSubtype, IFeatureClass
junctionFeatureClass, int junctionSubtype, bool defaultJunction)
{
IJunctionConnectivityRule2 junctionConnRule=new JunctionConnectivityRuleClass()
;
// Specify edge information.
junctionConnRule.EdgeClassID=edgeFeatureClass.ObjectClassID;
junctionConnRule.EdgeMaximumCardinality=2;
junctionConnRule.EdgeMinimumCardinality=1;
junctionConnRule.EdgeSubtypeCode=edgeSubtype;
// Specify junction information.
junctionConnRule.JunctionClassID=junctionFeatureClass.ObjectClassID;
junctionConnRule.JunctionMaximumCardinality=1;
junctionConnRule.JunctionMinimumCardinality=0;
junctionConnRule.JunctionSubtypeCode=junctionSubtype;
// Indicate whether this junction is the default junction.
junctionConnRule.DefaultJunction=defaultJunction;
// Add the rule to the geometric network.
geometricNetwork.AddRule(junctionConnRule);
}
[VB.NET] Public Sub AddEdgeJunctionConnectivityRule(ByVal geometricNetwork As IGeometricNetwork, _
ByVal edgeFeatureClass As IFeatureClass, ByVal edgeSubtype As Integer, _
ByVal junctionFeatureClass As IFeatureClass, ByVal junctionSubtype As Integer, _
ByVal defaultJunction As Boolean)
Dim junctionConnRule As IJunctionConnectivityRule2=New JunctionConnectivityRuleClass()
' Specify edge information.
junctionConnRule.EdgeClassID=edgeFeatureClass.ObjectClassID
junctionConnRule.EdgeMaximumCardinality=2
junctionConnRule.EdgeMinimumCardinality=1
junctionConnRule.EdgeSubtypeCode=edgeSubtype
' Specify junction information.
junctionConnRule.JunctionClassID=junctionFeatureClass.ObjectClassID
junctionConnRule.JunctionMaximumCardinality=1
junctionConnRule.JunctionMinimumCardinality=0
junctionConnRule.JunctionSubtypeCode=junctionSubtype
' Indicate whether this junction is the default junction.
junctionConnRule.DefaultJunction=defaultJunction
' Add the rule to the geometric network.
geometricNetwork.AddRule(junctionConnRule)
End Sub
Creating edge-edge connectivity rules
An edge - edge connectivity rule establishes the possible valid network connections that can exist between two edge features and which junction types are valid at the connection point between two edges. Additionally, edge-edge connectivity rules support the notion of a default junction, making it possible to specify the type of junction that should be placed at the point of connectivity between two edge features. Edge - edge rules are created with the EdgeConnectivityRule class and the IEdgeConnectivityRule interface.
FromEdgeClassID and ToEdgeClassID
The FromEdgeClassID and ToEdgeClassID properties specify the class IDs for the two types of edges participating in the rule. The class IDs can point to the feature class. These properties are required.
FromEdgeSubtypeCode and ToEdgeSubtypeCode
Since edge-edge rules are defined at the class and subtype level, specify the class and subtype of the from and to edges. If subtypes are not specified for either class, use the subtype code of zero. Neglecting to specify either value results in an invalid connectivity rule. These properties are required.
Cardinality
Cardinality for edge-edge connectivity rules is governed through the edge-junction rules that are created as a result of the edge-edge rule. Before adding a new junction to a rule, determine if the junction is already associated with the rule through the use of the ContainsJunction method.
While there must be at least one junction associated with an edge-edge rule, there is no limit to the number of junctions that can be associated with a rule.
Junction information
Specifying the valid set of junctions for an edge-edge rule is a two step process - adding the valid set of junctions and specifying which junction class subtype pairing is the default junction.
Use the AddJunction method to add a valid junction class subtype pairing to an edge-edge connectivity rule. To add an entire set of subtypes for a feature class to a rule, add each subtype individually. All subtypes for a feature class cannot be specified with one call.
Once all of the permissible junction class subtype pairings have been added to the rule, indicate which of the class subtype pairings should be the default junction for the rule. If the business rules that drive the creation of connectivity rules do not have a clear default junction class or type, set the Orphan Junction feature class as the default junction class.
When adding junctions and specifying a default class, subtype pairings are required aspects of edge-edge rules. Not specifying these parameters result in an invalid connectivity rule.
It is not possible to remove a junction from an edge-edge rule. If this is required, the rule must be deleted, then re-created minus the junction in question. See the following code example:
Edge - junction rules can be used to make edge - edge rules more flexible. In a waste water network, a rule can be that a Catch Basin Lead must have a Catch Basin junction at one end and a Manhole junction at the other end. To make the edge-edge rule more flexible, add the edge - edge rule between the two junction types so that the edge-junction rules between the Catch Basin Lead - Catch Basin and Catch Basin Lead - Manholes are created. Navigate to each of these edge - junction rules and set the edge - junction cardinality so that only one junction of each type can connect to any edge.
public void AddEdgeConnectivityRule(IGeometricNetwork geometricNetwork,
IFeatureClass fromEdgeFeatureClass, IFeatureClass toEdgeFeatureClass,
IFeatureClass junctionFeatureClass, int fromEdgeSubtype, int toEdgeSubtype, int
junctionSubtype)
{
IEdgeConnectivityRule edgeConnRule=new EdgeConnectivityRuleClass();
// Specify "from" edge information.
edgeConnRule.FromEdgeClassID=fromEdgeFeatureClass.ObjectClassID;
edgeConnRule.FromEdgeSubtypeCode=fromEdgeSubtype;
// Specify "to" edge information.
edgeConnRule.ToEdgeClassID=toEdgeFeatureClass.ObjectClassID;
edgeConnRule.ToEdgeSubtypeCode=toEdgeSubtype;
// Check if the junction is already associated with the rule.
// If not, add a junction class subtype pair to the edge-edge rule.
if (!edgeConnRule.ContainsJunction(junctionFeatureClass.ObjectClassID, 0))
{
edgeConnRule.AddJunction(junctionFeatureClass.ObjectClassID, 0);
}
// Specify a default junction type.
edgeConnRule.DefaultJunctionClassID=junctionFeatureClass.ObjectClassID;
edgeConnRule.DefaultJunctionSubtypeCode=junctionSubtype;
// Add the rule to the geometric network.
geometricNetwork.AddRule(edgeConnRule);
}
[VB.NET] Public Sub AddEdgeConnectivityRule(ByVal geometricNetwork As IGeometricNetwork, _
ByVal fromEdgeFeatureClass As IFeatureClass, ByVal toEdgeFeatureClass As IFeatureClass, _
ByVal junctionFeatureClass As IFeatureClass, ByVal fromEdgeSubtype As Integer, _
ByVal toEdgeSubtype As Integer, ByVal junctionSubtype As Integer)
Dim edgeConnRule As IEdgeConnectivityRule=New EdgeConnectivityRuleClass()
' Specify "from" edge information.
edgeConnRule.FromEdgeClassID=fromEdgeFeatureClass.ObjectClassID
edgeConnRule.FromEdgeSubtypeCode=fromEdgeSubtype
' Specify "to" edge information.
edgeConnRule.ToEdgeClassID=toEdgeFeatureClass.ObjectClassID
edgeConnRule.ToEdgeSubtypeCode=toEdgeSubtype
' Check if the junction is already associated with the rule.
' If not, add a junction class subtype pair to the edge-edge rule.
If Not edgeConnRule.ContainsJunction(junctionFeatureClass.ObjectClassID, 0) Then
edgeConnRule.AddJunction(junctionFeatureClass.ObjectClassID, 0)
End If
' Specify a default junction type.
edgeConnRule.DefaultJunctionClassID=junctionFeatureClass.ObjectClassID
edgeConnRule.DefaultJunctionSubtypeCode=junctionSubtype
' Add the rule to the geometric network.
geometricNetwork.AddRule(edgeConnRule)
End Sub
Accessing connectivity rules
The geodatabase supports numerous types of rules, such as attribute, relationship, connectivity, and topology rules. There are two ways to access connectivity rules given to a geometric network. The IGeometricNetwork.Rules property returns an enumerator containing every connectivity rule for a geometric network. The IGeometricNetwork.RulesByClassandSubtype property returns an enumerator containing only those connectivity rules associated with the specified class and subtype.
The following code example demonstrates how to generate an enumerator of connectivity rules for a geometric network based on specifying a particular class ID and subtype value:
[C#] public void GetConnectivityRulesForClassSubtype(IGeometricNetwork geometricNetwork,
int classID, int subtype)
{
IFeatureClassContainer featureClassContainer=(IFeatureClassContainer)
geometricNetwork;
// Get an enumerator of rules associated with the specified class ID and subtype.
IEnumRule enumRules=geometricNetwork.get_RulesByClassAndSubtype(classID,
subtype);
enumRules.Reset();
IRule rule=null;
int fromClassId= - 1;
int fromSubtypeCode= - 1;
int toClassId= - 1;
int toSubtypeCode= - 1;
while ((rule=enumRules.Next()) != null)
{
// For each rule in the enumerator, determine which type it is and print some information.
switch (rule.Type)
{
case esriRuleType.esriRTEdgeConnectivity:
IEdgeConnectivityRule edgeConnectivityRule=(IEdgeConnectivityRule)
rule;
fromClassId=edgeConnectivityRule.FromEdgeClassID;
toClassId=edgeConnectivityRule.ToEdgeClassID;
fromSubtypeCode=edgeConnectivityRule.FromEdgeSubtypeCode;
toSubtypeCode=edgeConnectivityRule.ToEdgeSubtypeCode;
break;
case esriRuleType.esriRTJunctionConnectivity:
IJunctionConnectivityRule junctionConnectivityRule=
(IJunctionConnectivityRule)rule;
fromClassId=junctionConnectivityRule.EdgeClassID;
toClassId=junctionConnectivityRule.JunctionClassID;
fromSubtypeCode=junctionConnectivityRule.EdgeSubtypeCode;
toSubtypeCode=junctionConnectivityRule.JunctionSubtypeCode;
break;
}
IFeatureClass fromClass=featureClassContainer.get_ClassByID(fromClassId);
IFeatureClass toClass=featureClassContainer.get_ClassByID(toClassId);
Console.WriteLine("{0}:{1}, {2}:{3}", fromClass.AliasName, fromSubtypeCode,
toClass.AliasName, toSubtypeCode);
}
}
[VB.NET] Public Sub GetConnectivityRulesForClassSubtype(ByVal geometricNetwork As IGeometricNetwork, _
ByVal classID As Integer, ByVal subtype As Integer)
Dim featureClassContainer As IFeatureClassContainer=CType(geometricNetwork, IFeatureClassContainer)
' Get an enumerator of rules associated with the specified class ID and subtype.
Dim enumRules As IEnumRule=geometricNetwork.RulesByClassAndSubtype(classID, subtype)
enumRules.Reset()
Dim rule As IRule=Nothing
Dim fromClassId As Integer=-1
Dim fromSubtypeCode As Integer=-1
Dim toClassId As Integer=-1
Dim toSubtypeCode As Integer=-1
rule=enumRules.Next()
While Not rule Is Nothing
' For each rule in the enumerator, determine which type it is and print some information.
Select Case rule.Type
Case esriRuleType.esriRTEdgeConnectivity
Dim edgeConnectivityRule As IEdgeConnectivityRule=CType(rule, IEdgeConnectivityRule)
fromClassId=edgeConnectivityRule.FromEdgeClassID
toClassId=edgeConnectivityRule.ToEdgeClassID
fromSubtypeCode=edgeConnectivityRule.FromEdgeSubtypeCode
toSubtypeCode=edgeConnectivityRule.ToEdgeSubtypeCode
Case esriRuleType.esriRTJunctionConnectivity
Dim junctionConnectivityRule As IJunctionConnectivityRule=CType(rule, IJunctionConnectivityRule)
fromClassId=junctionConnectivityRule.EdgeClassID
toClassId=junctionConnectivityRule.JunctionClassID
fromSubtypeCode=junctionConnectivityRule.EdgeSubtypeCode
toSubtypeCode=junctionConnectivityRule.JunctionSubtypeCode
End Select
Dim fromClass As IFeatureClass=featureClassContainer.ClassByID(fromClassId)
Dim toClass As IFeatureClass=featureClassContainer.ClassByID(toClassId)
Console.WriteLine("{0}:{1}, {2}:{3}", fromClass.AliasName, fromSubtypeCode, _
toClass.AliasName, toSubtypeCode)
rule=enumRules.Next()
End While
End Sub
To use the code in this topic, reference the following assemblies in your Visual Studio project. In the code files, you will need using (C#) or Imports (VB .NET) directives for the corresponding namespaces (given in parenthesis below if different from the assembly name):
Development licensing | Deployment licensing |
---|---|
ArcGIS Desktop Standard | ArcGIS Desktop Standard |
ArcGIS Desktop Advanced | ArcGIS Desktop Advanced |
Engine Developer Kit | Engine: Geodatabase Update |