Click here to get the sample associated with this walkthrough.
In this topic
- Create a stand-alone MapControl application
- Add additional tools that interact with the dynamic display
- Create a command that enables dynamic display mode
- Test the application
- Persist the dynamic display cache by saving the map document
- Implement a dynamic layer
- Implement a command to load the dynamic layer
- Run the completed application
Create a stand-alone MapControl application
Complete the following steps to use the Visual Studio ArcGIS integration framework to create a stand-alone ArcGIS Engine application:
-
Start Visual Studio.
-
Click File, click New, and click Project. The New Project dialog box appears.
-
Depending on the language you use (C# or VB .NET), on the Project types pane, click to expand the language node, click ArcGIS, then click Extending ArcObjects.
-
On the Templates pane, click the MapControl Application template.
-
Name the application MyDynamicDisplayApp and click OK. When you click OK on the New Project dialog box, Visual Studio creates a solution that has a basic MapControl application.
See the following screen shot:
Add additional tools that interact with the dynamic display
To take advantage of dynamic display functionality, complete the following steps to add additional tools to roam the map, continuously zoom in and out, and rotate the map display:
-
Right-click MainForm.cs on the Solution Explorer or MainForm.vb if you created the application using Visual Basic.
-
Click View Designer to open the application in design mode. See the following screen shot:
- In design mode, right-click the application's toolbar and click Properties. The toolbar's Properties dialog box appears.
- Under the Items tab, click Add. The Controls Commands (customize) dialog box appears.
- Under the Commands tab on the Controls Commands dialog box, click Map Navigation on the Category pane.
- On the Commands pane, drag the following tools on to the toolbar on the Properties dialog box:
- Continuous Zoom and Pan
- Roam
- Rotate Data Frame
- Clear Rotation
See the following screen shot:
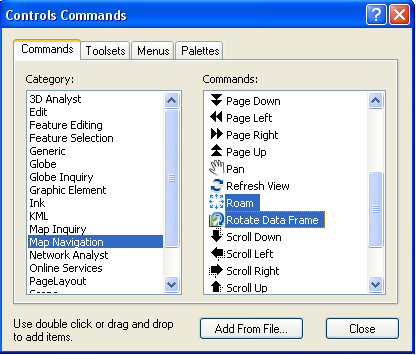
When done, the toolbar on the Properties dialog box resembles the following screen shot:
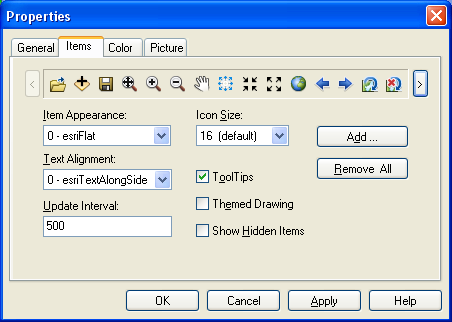
You now have the tools that can interact with the dynamic display. Next, create a custom command to enable dynamic display for the map.
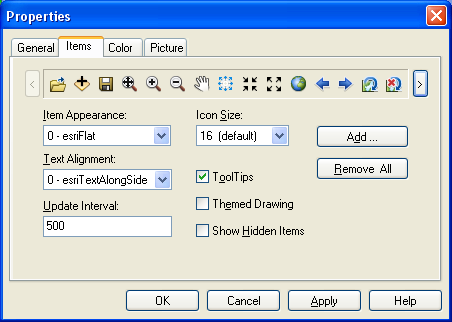
You now have the tools that can interact with the dynamic display. Next, create a custom command to enable dynamic display for the map.
Create a command that enables dynamic display mode
Complete the following steps to implement a custom command by extending the ArcGIS Engine BaseCommand class:
-
Right-click MyDynamicDisplayApp on the Solution Explorer, click Add, then click New Item. The Add New Item dialog box appears.
See the following screen shot that shows the Solution Explorer with the Add and New Item options:
- On the Add New Item dialog box, under the Categories pane, click to expand the Visual C# or Visual Basic node, click the ArcGIS node, then click the Extending ArcObjects node.
- On the Templates pane, click the Base Command template. Name the command ToggleDynamicDisplayCmd.cs (or ToogleDynamicDisplayCmd.vb) and click Add. See the following screen shot:
- On the ArcGIS New Item Wizard Options dialog box, click the ArcMap, MapControl, or PageLayoutControl command, and click OK.
- Visual Studio creates a command that inherits from the ArcGIS BaseCommad class.
- To avoid using fully qualified names, add a using statement (Import in VB. NET) to your command class.
- On the Solution Explorer, double-click ToggleDynamicDisplayCmd to view its code. At the top of the class (above the namespace block), add the following code example:
using ESRI.ArcGIS.Carto;
[VB.NET] Imports ESRI.ArcGIS.Carto
- Set the command properties. In the class constructor, set the base class members that define command properties, such as name, caption, ToolTip, and so on. See the following code example:
base.m_category="Toggle dynamic display";
base.m_caption="Toggle dynamic mode";
base.m_message="Toggle dynamic mode on and off";
base.m_toolTip="Toggle dynamic mode";
base.m_name="MyDynamicDisplayApp_ToggleDynamicDisplayCmd";
[VB.NET] MyBase.m_category="Toggle dynamic display"
MyBase.m_caption="Toggle dynamic mode"
MyBase.m_message="Toggle dynamic mode on and off"
MyBase.m_toolTip="Toggle dynamic mode"
MyBase.m_name="MyDynamicDisplayApp_ToggleDynamicDisplayCmd"
- Add a class member to reference the dynamic map. Before the class constructor (below the hookhelper class member), add the class member in the following code example:
private IDynamicMap m_dynamicMap;
[VB.NET] Private m_dynamicMap As IDynamicMap
- Implement the OnClick method. When the user clicks the command, verify that the focus map supports dynamic map. Next, set the dynamic display draw rate (in milliseconds) and toggle dynamic mode. See the following code example:
public override void OnClick()
{
m_dynamicMap=m_hookHelper.FocusMap as IDynamicMap;
if (m_dynamicMap == null)
return ;
m_dynamicMap.DynamicDrawRate=15;
m_dynamicMap.DynamicMapEnabled=!m_dynamicMap.DynamicMapEnabled;
}
[VB.NET] m_dynamicMap=CType(m_hookHelper.FocusMap, IDynamicMap)
If (m_dynamicMap Is Nothing) Then Return
m_dynamicMap.DynamicDrawRate=15
m_dynamicMap.DynamicMapEnabled=Not (m_dynamicMap.DynamicMapEnabled)
- Override the command's Checked property to indicate whether or not the map is in dynamic display mode. This displays the command as "pushed in" when the map is in dynamic display mode. See the following code example:
public override bool Checked{
}
[VB.NET] Public Overrides ReadOnly Property Checked() As Boolean
You can use Visual Studio Intellisense to automatically override the property. After typing public override and pressing the spacebar, Visual Studio suggests the available methods and properties to override.
- In the Checked property, add the following code example to check or uncheck the command:
public override bool Checked
{
get
{
if (m_dynamicMap == null)
return false;
return m_dynamicMap.DynamicMapEnabled;
}
}
[VB.NET] Public Overrides ReadOnly Property Checked() As Boolean
Get
If (m_dynamicMap Is Nothing) Then Return False
Return m_dynamicMap.DynamicMapEnabled
End Get
End Property
- Add the command to the application toolbar. Since the command has been created inside an executable application, it cannot register to the proper command category; therefore, add the command to the application's toolbar at run time.
Switch to MainForm.cs (or MainForm.vb) code view and scroll to the MainForm_Load method. At the end of the method, provide the following code example to add the command to the application toolbar:
axToolbarControl1.AddItem(new ToggleDynamicDisplayCmd());
[VB.NET] axToolbarControl1.AddItem(New ToggleDynamicDisplayCmd())
Test the application
To test the application, add data from ArcGIS Online services. Complete the following steps:
- In Visual Studio, press F5 to launch the application.
-
Click the Add Data command and click the Servers category on the Add Data dialog box.
-
At the top of the dialog box, click ArcGIS Server to create an ArcGIS server connection.
-
On the Add Server connection dialog box, select the Internet ArcGIS Server radio button and set the following server uniform resource locator (URL) to http://services.arcgisonline.com/v92.
-
Click OK to add the connection and close the dialog box.
-
From the ArcGIS connection layers list, click I3_Imagery_Prime_World_2D, then click Open to add the ArcGIS server layer to the map.
-
Click your custom Toggle dynamic mode command to switch to dynamic display mode.
-
Zoom, pan, and roam the map. Experiment with the Continuous Pan and Zoom tool, and the Rotate Data Frame tool.
-
When done, toggle off dynamic mode.
-
Leave the application running for the next step.
-
The Roam tool requires the map to be in dynamic display mode and becomes disabled if it is not. Other commands, such as the Continuous Pan and Zoom tool, work in dynamic and nondynamic display modes.
In dynamic mode, there are no blank envelopes as you pan the map. When you zoom or pan to a new location, the map initially displays at a coarse resolution and refines it gradually.
Persist the dynamic display cache by saving the map document
To persist the cache that dynamic display creates for each layer, complete the following steps to save your map or alternatively, save individual layers as layer files:
-
In your application, click File, and click Save As.
-
Save the map locally as MyDynamicDisplayDoc.mxd.
-
Shut down the application and rerun it by pressing F5 (while in Visual Studio).
-
Click File, and click Open Document.
-
Browse to the document you saved earlier and open it.
-
To switch to dynamic mode, on the application toolbar, click the Toggle Dynamic Mode button.
This time the data is loaded almost immediately.
-
Pan and zoom to areas that you used in the section, Test the application. Each tile you previously viewed loads almost immediately.
-
Close the application.
When you enable the dynamic display, it creates a separate cache for each layer in your map. To reuse the cache on the next session of your application, enable dynamic mode once, and only then save your map as a map document or save individual layers as layer files.
Implement a dynamic layer
Complete the following steps to create a simple dynamic layer that uses the dynamic layer base class of the ESRI.ArcGIS.ADF.Local assembly (the dynamic layer draws from a simulated dynamic feed):
-
On the Solution Explorer, expand the References folder, and double-click the ESRI.ArcGIS.ADF.Local assembly reference to open the Object Browser.
-
On the Object Browser tree, click the ESRI.ArcGIS.ADF.Local node, then click to expand the ESRI.ArcGIS.ADF.BaseClasses node.
-
Click the BaseDynamicLayer node to view the methods and properties it implements. Also, click the Base Types node and view the base types it implements. See the following screen shot:
- Click the DrawDynamicLayer method. This method is defined as an abstract method, which means to implement the base class, you must override and implement the method.
- Close the Object Browser.
- Add a new standard .NET class and name it MyDynamicLayer.cs (or MyDynamicLayer.vb). See the following screen shot:
- On the Solution Explorer, right-click the References folder, click Add ArcGIS Reference, click to expand the Engine group, and select the following assemblies to add to the application references:
- ESRI.ArcGIS.ADF.Connection.Local
- ESRI.ArcGIS.Geodatabase
- ESRI.ArcGIS.Geometry
- On the Solution Explorer, right-click the References folder and click Add References to the stdole and System Timers assemblies. Use objects in these assemblies to create character marker symbols and to signal the dynamic display to redraw at a specified time interval.
- Add using statements in the following code example to avoid having to use fully qualified names:
using System.Drawing;
using System.Timers;
using ESRI.ArcGIS.ADF;
using ESRI.ArcGIS.ADF.BaseClasses;
using ESRI.ArcGIS.Geodatabase;
using ESRI.ArcGIS.Geometry;
using ESRI.ArcGIS.Carto;
using ESRI.ArcGIS.Display;
[VB.NET] Imports System.Drawing
Imports ESRI.ArcGIS.ADF
Imports ESRI.ArcGIS.ADF.BaseClasses
Imports ESRI.ArcGIS.Geodatabase
Imports ESRI.ArcGIS.Geometry
Imports ESRI.ArcGIS.Carto
Imports ESRI.ArcGIS.Display
- Inherit your class from BaseDynamicLayer. Make the class public in scope to create an instance of it later. Designate the class as a sealed class, which means it cannot be inherited in the future. See the following code example:
public sealed class MyDynamicLayer: BaseDynamicLayer{
}
[VB.NET] Public Class MyDynamicLayer
Inherits BaseDynamicLayer
End Class
- Add class members to create several member variables for the class. These variables store geometry and symbols used by the layer, ensure the (one time) initialization of objects, and control the interval where the layer is redrawn. See the following code example:
public bool m_bOnce=true;
private IDynamicGlyph m_myGlyph=null;
private IDynamicSymbolProperties2 m_dynamicSymbolProps=null;
private IPoint m_point=null;
private double m_stepX=0;
private double m_stepY=0;
private Timer m_updateTimer=null;
[VB.NET] Public m_bOnce As Boolean=True
Private m_myGlyph As IDynamicGlyph=Nothing
Private m_dynamicSymbolProps As IDynamicSymbolProperties2=Nothing
Private m_point As IPoint=Nothing
Private m_stepX As Double=0
Private m_stepY As Double=0
Private m_updateTimer As System.Timers.Timer=Nothing
- Create a class constructor that calls the constructor for the base class. Your constructor also provides a default name for the layer and initializes the timer object that controls the layer redraw interval. See the following code example:
public MyDynamicLayer(): base()
{
base.m_sName="My Dynamic layer";
m_updateTimer=new Timer(15);
m_updateTimer.Enabled=false;
m_updateTimer.Elapsed += new ElapsedEventHandler(OnTimerElapsed);
}
[VB.NET] Public Sub New()
MyBase.New()
MyBase.m_sName="My Dynamic layer"
m_updateTimer=New System.Timers.Timer(15)
m_updateTimer.Enabled=False
AddHandler m_updateTimer.Elapsed, AddressOf OnTimerElapsed
End Sub
- Implement the timer update method. The timer elapsed event handler is where you will set the layer's dirty flag to true. Setting this flag signals to the dynamic display that the layer was updated and needs to be redrawn. This way, the dynamic display on its next cycle draws all the dynamic layers that support the given draw phase that was set to true (esriDDPImmediate or esriDDPCompiled).
Normally, the update method is where you update your dynamic items. It can be an event that gets fired while listening to an external feed that updates the dynamic layer.
Remember, most .NET event handlers are executed on a thread other than the main thread. For that reason, take measures to avoid calling ArcObjects directly from the event handler, since ArcObjects are Component Object Model (COM) objects and therefore, cannot be shared across multiple threads. For more information, see Writing multithreaded ArcObjects code and How to use IGeometryBridge to update dynamic geometries.
See the following code example:
void OnTimerElapsed(object sender, ElapsedEventArgs e)
{
// Set the dirty flag to true to ensure the next drawing cycle.
base.m_bIsImmediateDirty=true;
}
[VB.NET] Private Sub OnTimerElapsed(ByVal Source As Object, ByVal e As Timers.ElapsedEventArgs)
MyBase.m_bIsImmediateDirty=True
End Sub
- Override the DrawDynamicLayer method since it is marked as an abstract class. If you are using C#, right-click BaseDynamicLayer in the class definition and choose Implement Abstract Class. If you are using Visual Basic, select the DrawDynamicLayer method from the method list at the top of the code document. Visual Studio implements the overridden method and adds code that throws an exception, which marks the method as not implemented. Delete the default code. See the following screen shot:
See the following code example:
public override void DrawDynamicLayer(esriDynamicDrawPhase DynamicDrawPhase,
IDisplay Display, IDynamicDisplay DynamicDisplay){
}
[VB.NET] Public Overrides Sub DrawDynamicLayer(ByVal DynamicDrawPhase As esriDynamicDrawPhase, ByVal Display As IDisplay, ByVal DynamicDisplay As IDynamicDisplay)
End Sub
In the following steps, implement the DrawDynamicLayer method, which is the core of the dynamic layer:
- Ensure the layer is valid and visible. See the following code example:
if (!m_bValid || !m_visible)
return ;
[VB.NET] If ((Not m_bValid) Or (Not m_visible)) Then Return
- Ensure the input draw phase is immediate (esriDDPImmediate). Dynamic drawing methods have two supported draw phases—compiled and immediate—(esriDDPCompiled and esriDDPImmediate, respectively). Use the immediate draw phase for dynamic layers whose data is highly dynamic and when changes should take place as soon as they happen. The compiled mode can be used for dynamic layers that are less dynamic by nature. Ensure your layer is only drawing in one of the two draw phases; otherwise, your layer draws twice on each drawing cycle. See the following code example:
if (DynamicDrawPhase != esriDynamicDrawPhase.esriDDPImmediate)
return ;
[VB.NET] If (DynamicDrawPhase <> esriDynamicDrawPhase.esriDDPImmediate) Then Return
- Get the visible extent. Set the speed on the dynamic item and update the position of the dynamic item in the map. See the following code example:
IEnvelope visibleExtent=Display.DisplayTransformation.FittedBounds;
[VB.NET] Dim visibleExtent As IEnvelope=Display.DisplayTransformation.FittedBounds
- Perform a one-time initialization of objects. The first time the dynamic display calls the dynamic layer drawing command, required objects will be initialized. Subsequent calls use the existing class member variables rather than reinitializing them. Begin by checking the m_bOnce flag to determine if object variables need to be initialized. See the following code example:
if (m_bOnce)
{
[VB.NET] If (m_bOnce) Then
- If this is the first call to the method, get the dynamic glyph factory. This object will be used to create the dynamic glyph and cache the dynamic symbol properties. See the following code example:
IDynamicGlyphFactory dynamicGlyphFactory=DynamicDisplay.DynamicGlyphFactory;
m_dynamicSymbolProps=DynamicDisplay as IDynamicSymbolProperties2;
[VB.NET] Dim dynamicGlyphFactory As IDynamicGlyphFactory=DynamicDisplay.DynamicGlyphFactory
m_dynamicSymbolProps=CType(DynamicDisplay, IDynamicSymbolProperties2)
- Create a marker glyph from a white-character marker symbol. Choosing the color white for your glyph allows you to set to it any color; therefore, allows you to reuse the same glyph over and over, save resources, and gain performance. See the following code example:
ICharacterMarkerSymbol markerSymbol=new CharacterMarkerSymbolClass();
markerSymbol.Font=ESRI.ArcGIS.ADF.Connection.Local.Converter.ToStdFont(new Font(
"ESRI Default Marker", 25.0f, FontStyle.Bold));
markerSymbol.Size=25.0;
// Set the symbol color to white.
markerSymbol.Color=(IColor)ESRI.ArcGIS.ADF.Connection.Local.Converter.ToRGBColor
(Color.FromArgb(255, 255, 255));
markerSymbol.CharacterIndex=92;
// Create the dynamic glyph.
m_myGlyph=dynamicGlyphFactory.CreateDynamicGlyph((ISymbol)markerSymbol);
[VB.NET] Dim markerSymbol As ICharacterMarkerSymbol=New CharacterMarkerSymbolClass()
markerSymbol.Font=Converter.ToStdFont(New Font("ESRI Default Marker", 25.0F, FontStyle.Bold))
markerSymbol.Size=25.0
' Set the symbol color to white.
markerSymbol.Color=CType(Converter.ToRGBColor(Color.FromArgb(255, 255, 255)), IColor)
markerSymbol.CharacterIndex=92
' Create the dynamic glyph.
m_myGlyph=dynamicGlyphFactory.CreateDynamicGlyph(CType(markerSymbol, ISymbol))
Creating dynamic glyphs using white as the color allows you to save resources and gain performance by reusing the same glyph over and over again. You can color the dynamic glyph to any color using IDynamicSymbolProperties.SetColor and scale the dynamic glyph using IDynamicsymbolProperties.SetScale.
- Initialize the dynamic item's geometry and initialize its step size (the interval in map units by which you increment the item's location on each drawing cycle). See the following code example:
Random r=new Random();
double X=visibleExtent.XMin + r.NextDouble() * visibleExtent.Width;
double Y=visibleExtent.YMin + r.NextDouble() * visibleExtent.Height;
m_point=new PointClass();
m_point.PutCoords(X, Y);
m_stepX=visibleExtent.Width / 250;
m_stepY=visibleExtent.Height / 250;
[VB.NET] Dim r As Random=New Random()
Dim X As Double=visibleExtent.XMin + r.NextDouble() * visibleExtent.Width
Dim Y As Double=visibleExtent.YMin + r.NextDouble() * visibleExtent.Height
m_point=New PointClass()
m_point.PutCoords(X, Y)
m_stepX=visibleExtent.Width / 250
m_stepY=visibleExtent.Height / 250
- Enable the update timer. See the following code example:
m_updateTimer.Enabled=true;
[VB.NET] m_updateTimer.Enabled=True
- Finish the one-time initialization step by setting the Once flag to false and closing the initialization code block. See the following code example:
m_bOnce=false;
}
[VB.NET] m_bOnce=False
End If
- Draw the dynamic element. Each time the dynamic display calls the layer's DrawDynamicLayer method, it redraws the dynamic items if it is using the immediate draw phase. Since dynamic display is a state machine, each time before drawing, set the dynamic symbol. In the case of a marker symbol, set the dynamic glyph, and color and scale of the symbol. Eventually, you will be drawing the geometry. See the following code example:
// Draw the marker.
m_dynamicSymbolProps.set_DynamicGlyph(esriDynamicSymbolType.esriDSymbolMarker,
m_myGlyph);
m_dynamicSymbolProps.SetColor(esriDynamicSymbolType.esriDSymbolMarker, 1.0f, 0.0f,
0.0f, 1.0f);
m_dynamicSymbolProps.SetScale(esriDynamicSymbolType.esriDSymbolMarker, 1.0f, 1.0f);
DynamicDisplay.DrawMarker(m_point);
[VB.NET] ' Draw the marker.
m_dynamicSymbolProps.DynamicGlyph(esriDynamicSymbolType.esriDSymbolMarker)=m_myGlyph
m_dynamicSymbolProps.SetColor(esriDynamicSymbolType.esriDSymbolMarker, 1.0F, 0.0F, 0.0F, 1.0F)
m_dynamicSymbolProps.SetScale(esriDynamicSymbolType.esriDSymbolMarker, 1.0F, 1.0F)
DynamicDisplay.DrawMarker(m_point)
- Update the dynamic item. In the following code example, use synthetic information that simulates a dynamic data feed to update the location of the dynamic item:
// Update the point location for the next draw cycle.
m_point.X += m_stepX;
m_point.Y += m_stepY;
// Ensure the point falls within the visible extent.
if (m_point.X > visibleExtent.XMax)
m_stepX= - Math.Abs(m_stepX);
if (m_point.X < visibleExtent.XMin)
m_stepX=Math.Abs(m_stepX);
if (m_point.Y > visibleExtent.YMax)
m_stepY= - Math.Abs(m_stepY);
if (m_point.Y < visibleExtent.YMin)
m_stepY=Math.Abs(m_stepY);
[VB.NET] ' Update the point location for the next draw cycle.
m_point.X +=m_stepX
m_point.Y +=m_stepY
' Ensure the point falls within the visible extent.
If (m_point.X > visibleExtent.XMax) Then m_stepX=- Math.Abs(m_stepX)
If (m_point.X < visibleExtent.XMin) Then m_stepX=Math.Abs(m_stepX)
If (m_point.Y > visibleExtent.YMax) Then m_stepY=- Math.Abs(m_stepY)
If (m_point.Y < visibleExtent.YMin) Then m_stepY=Math.Abs(m_stepY)
- Set the layer's dirty flag to signal the dynamic display that the layer has been updated and needs to redraw. Set the dirty flag to false at the end of the draw method (since drawing is done). Set the dirty flag to true only when an item of the layer has changed and the layer needs to be redrawn. See the following code example:
// Set the dirty flag to true to ensure the next drawing cycle.
base.m_bIsImmediateDirty=false;
[VB.NET] ' Set the dirty flag to true to ensure the next drawing cycle.
MyBase.m_bIsImmediateDirty=False
Setting the dynamic layer's dirty flag to true signals to the dynamic display that the layer needs to be redrawn. According to the draw phase that your dynamic layer supports, set the appropriate draw flag only when an item of your layer has been updated.
Implement a command to load the dynamic layer
Since a dynamic layer is a custom layer, write code to load it to the map (by default, you cannot browse to it using the Add Data command). See the following steps:
-
Use the integration framework to add a new ArcGIS command. Name the new command, LoadDynamicLayerCmd. For more information on how to create commands using the integration framework, see the following section in this topic, Create a command that enables dynamic mode.
-
Add a using statement to avoid fully qualified names. See the following code example:
using ESRI.ArcGIS.Carto;
[VB.NET] Imports ESRI.ArcGIS.Carto
- In the class constructor, set the command properties. See the following code example:
base.m_category=".NET Samples";
base.m_caption="Add dynamic layer";
base.m_message="Add dynamic layer";
base.m_toolTip="Add dynamic layer";
base.m_name="MyDynamicDisplayApp.LoadDynamicLayerCmd";
[VB.NET] MyBase.m_category=".NET Samples"
MyBase.m_caption="Add dynamic layer"
MyBase.m_message="Add dynamic layer"
MyBase.m_toolTip="Add dynamic layer"
MyBase.m_name="MyDynamicDisplayApp.LoadDynamicLayerCmd"
- Implement the OnClick method to load the dynamic layer to the map.
- Verify the current focus map supports that dynamic display is enabled; otherwise, there is no point in loading your dynamic layer, since it will not draw in standard mode. See the following code example:
- Verify the current focus map supports that dynamic display is enabled; otherwise, there is no point in loading your dynamic layer, since it will not draw in standard mode. See the following code example:
IDynamicMap dynamicMap=m_hookHelper.FocusMap as IDynamicMap;
if (dynamicMap == null || dynamicMap.DynamicMapEnabled == false)
return ;
[VB.NET] Dim dynamicMap As IDynamicMap=CType(m_hookHelper.FocusMap, IDynamicMap)
If (dynamicMap Is Nothing Or dynamicMap.DynamicMapEnabled=False) Then Return
- Create an instance of the dynamic layer and load it to the map. See the following code example:
MyDynamicLayer dynamicLayer=new MyDynamicLayer();
m_hookHelper.FocusMap.AddLayer(dynamicLayer);
[VB.NET] Dim dynamicLayer As MyDynamicLayer=New MyDynamicLayer()
m_hookHelper.FocusMap.AddLayer(dynamicLayer)
- Add the command to the application toolbar. Switch to the code view for MainForm and scroll to the MainForm_Load method. At the end of the method, include the following code example to add the command to the application toolbar:
axToolbarControl1.AddItem(new LoadDynamicLayerCmd());
[VB.NET] axToolbarControl1.AddItem(New LoadDynamicLayerCmd())
Run the completed application
Complete the following steps to run the completed application:
- In Visual Studio, save your work and press F5 to run the application.
- Load MyDynamicDisplayDoc.mxd and click ToggleDynamicDisplayCmd to enable dynamic display mode.
- Zoom in and click LoadDynamicLayerCmd to load the dynamic layer to the map.
- A layer (My dynamic layer) is added to the table of contents (TOC) and a red circle bounces back and forth on the map. Clicking LoadDynamicLayerCmd again adds additional instances of your dynamic layer to the map.
- When done, toggle off dynamic mode and close the application.
See Also:
Dynamic displayBest practices for using dynamic display
How dynamic display works
How to use IGeometryBridge to update dynamic geometries
To use the code in this topic, reference the following assemblies in your Visual Studio project. In the code files, you will need using (C#) or Imports (VB .NET) directives for the corresponding namespaces (given in parenthesis below if different from the assembly name):
- System.Drawing
- ESRI.ArcGIS.ADF.Local
- ESRI.ArcGIS.Geodatabase
- ESRI.ArcGIS.Geometry
- ESRI.ArcGIS.Carto
- ESRI.ArcGIS.Display
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |