In this topic
- About consuming custom geoprocessing tools in ArcGIS Engine Java applications
- Create a toolbox
- Add the toolbox to the GeoProcessor object
- Create parameters and execute the tool
- Generate tool wrappers
- Publishing a custom tool in server
About consuming custom geoprocessing tools in Java applications
Once you've built a custom geoprocessing tool and registered it with ArcGIS, it can be consumed and executed in a Java application in the same manner as out-of-the-box ArcGIS geoprocessing function tools. The following diagram shows the three-step workflow to consume custom geoprocessing tools in a Java application:
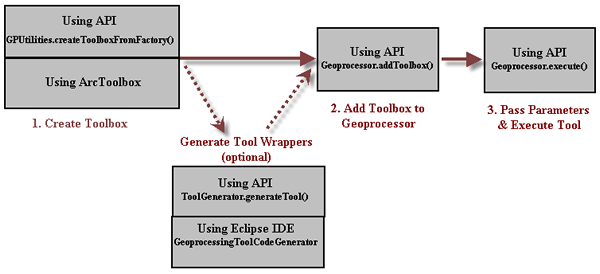
Create a toolbox
In general, the standard ArcGIS geoprocessing tools are bundled together in toolboxes that the ArcGIS framework is aware of by default. These toolboxes and it's tools can be accessed in Engine application. A list of the ArcGIS toolboxes is located at ArcGISHOME\ArcToolbox\Toolboxes. To consume custom geoprocessing function tools in a Java application, you must create a toolbox. A custom toolbox can be created using ArcToolbox (in ArcCatalog or ArcMap), or it can be created programmatically. To learn how to create a toolbox and import a custom GP tool into ArcGIS Desktop, please see the "Developing Extensions" -> "Custom Geoprocessing Tools" -> "Consume" -> "Consuming Custom GP tools in ArcGIS" topic.
A toolbox can also be created programmatically from the custom function factory using the Arcobjects API. When created in this manner, the toolbox contains the tools hosted by the specified custom function factory as shown in the following code snippet:
[Java]
//Use the utility class to create a toolbox.
public class CreateToolbox{
private GPUtilities gpUtilities;
createToolbox(){
try{
//The alias name of the custom function factory.
//Refer to the custom function factory Java class, IGPFunctionFactory.getAlias() method,
//that was created.
String functionFactoryAlias = "customjavafunctionfactory";
//Use any preferred location for your toolbox.
//Confirm the folder exists.
String toolBoxLocation = getOutputDir();
//Use any preferred name for your toolbox.
String toolBoxName = "Custom_Geoprocessing_Tools.tbx";
//Create the toolbox from the function factory.
//The custom function factory must be registered with ArcGIS before creating the toolbox.
gpUtilities = new GPUtilities();
gpUtilities.createToolboxFromFactory(functionFactoryAlias,
toolBoxLocation, toolBoxName);
}
catch (IOException e){
e.printStackTrace();
}
}
public static void main(String[] args){
try{
EngineInitializer.initializeEngine();
AoInitialize aoInit = new AoInitialize();
aoInit.initialize(esriLicenseProductCode.esriLicenseProductCodeEngine);
//Create the toolbox.
CreateToolbox createToolBox = new CreateToolbox();
aoInit.shutdown();
}
catch (Exception e){
e.printStackTrace();
}
}
private String getOutputDir(){
String userDir;
if (System.getProperty("os.name").toLowerCase().indexOf("win") > - 1)
userDir = System.getenv("UserProfile");
else
userDir = System.getenv("HOME");
String outputDir = userDir + "/arcgis_sample_output";
System.out.println("Creating output directory - " + outputDir);
new File(outputDir).mkdir();
return outputDir;
}
}
The custom function factory class and the custom geoprocessing tools classes must be deployed to ArcGIS before creating the toolbox. Also, it is very essential to verify that the name of your custom toolbox does not conflict with existing geoprocessing tool names.
Add the toolbox to the GeoProcessor object
The GeoProcessor class is a coarse grained ArcObjects component that allows you to access and execute ArcGIS geoprocessing tools in Java applications. The GeoProcessor object recognizes the standard ArcGIS geoprocessing tools; however, to access custom toolbox that you created, you must add your custom toolbox to the GeoProcessor object using GeoProcessor.addToolbox() method. See the code snippet below.
Create parameters and execute the tool
Once you've added the custom toolbox to the GeoProcessor object, you can execute the custom geoprocessing tools in your application in the same manner as you would access standard ArcGIS geoprocessing tools as shown in the following code:
[Java]
/* This is a utility class used to consume custom tools in a Java application.*/
public class ExecuteCustomTool{
private GeoProcessor geoprocessor;
public static void main(String args[]){
try{
//Initialize Engine.
EngineInitializer.initializeEngine();
AoInitialize aoInit = new AoInitialize();
aoInit.initialize(esriLicenseProductCode.esriLicenseProductCodeEngine);
ExecuteCustomTool obj = new ExecuteCustomTool();
//Execute DeleteFeatures.
obj.executeDeleteFeaturesTool();
}
catch (IOException e){
e.printStackTrace();
}
}
private void executeDeleteFeaturesTool(){
try{
geoprocessor = new GeoProcessor();
//Add the recently created toolbox.
geoprocessor.addToolbox(getOutputDir() +
"/Custom_Geoprocessing_Tools.tbx");
//Create parameters for the tool.
VarArray parameters = new VarArray();
String arcgishome = System.getenv("ARCGISHOME");
//Make a copy of the data, since executing the tool deletes the features.
String inputFeature = arcgishome + "java/samples/data/usa/states.lyr";
String inputSQLExpression = "\"STATE_NAME\"='Alabama'";
parameters.add(inputFeature);
parameters.add(inputSQLExpression);
//Set OverwriteOutput to true since the output parameter is of type derived.
//Execution of the tool overwrites the input data.
geoprocessor.setOverwriteOutput(true);
geoprocessor.execute("CustomDeleteFeatures", parameters, null);
//Print messages, if any, from the tool.
for (int i = 0; i < geoprocessor.getMessageCount(); i++)
System.out.println(geoprocessor.getMessage(i));
}
catch (IOException e){
e.printStackTrace();
}
}
private String getOutputDir(){
String userDir;
if (System.getProperty("os.name").toLowerCase().indexOf("win") > - 1)
userDir = System.getenv("UserProfile");
else
userDir = System.getenv("HOME");
String outputDir = userDir + "/arcgis_sample_output";
System.out.println("Creating output directory - " + outputDir);
new File(outputDir).mkdir();
return outputDir;
}
}
Generate tool wrappers
Tool wrappers are Java classes that represent geoprocessing tools. Every ArcGIS geoprocessing tool has a wrapper class in the com.esri.arcgis.geoprocessing.tools package. The Java wrapper classes simplify execution of the tool.
The traditional method of executing tools, using the GeoProcessor.execute() method, requires that you construct a VarArray for parameters, then add the parameters in the order required by the tool. This is shown in the previous code.
When executing a tool through a Java wrapper class, the following takes place:
- Tool parameters are defined as properties of the Java wrapper class. The parameters can be defined using setter methods.
- The Java wrapper class constructor accepts mandatory tool parameters.
The Java wrapper classes reduce code clutter and parameter order restrictions of VarArray when executing geoprocessing tools.
The following code snippet shows how to reduce code clutter by using Java wrapper classes, rather than creating a parameter array, to execute the Buffer tool:
[Java]
//Import com.esri.arcgis.geoprocessing.tools.analysistools.Buffer.
private void executeTool(String inputLocation, String outputLocation, double
distance)throws Exception{
Geoprocessor geoprocessor = new Geoprocessor();
Buffer buffer = new Buffer();
buffer.setInFeatures(inputLocation);
buffer.setOutFeatureClass(outputLocation);
buffer.setBufferDistanceOrField(distance);
geoprocessor.execute(buffer, null);
}
You can create tool wrappers for custom geoprocessing tools that were built using the API as well as those built using the Geoprocessing Tool Code Generator that is available as a wizard in the Eclipse integrated development environment (IDE). The following code shows how to create a Java wrapper class for a custom geoprocessing tool:
[Java]
/* This is a utility class that generates a wrapper for the DeleteFeatures tool.*/
public class GenerateWrappers{
private GeoProcessor geoprocessor;
public static void main(String args[]){
try{
//Initialize Engine.
EngineInitializer.initializeEngine();
AoInitialize aoInit = new AoInitialize();
aoInit.initialize(esriLicenseProductCode.esriLicenseProductCodeEngine);
GenerateWrappers obj = new GenerateWrappers();
obj.genWrapperDeleteFeaturesTool();
}
catch (IOException e){
e.printStackTrace();
}
}
public void genWrapperDeleteFeaturesTool(){
try{
this.geoprocessor = new GeoProcessor();
//Ensure that the toolbox is in a given location.
this.geoprocessor.addToolbox(getOutputDir() +
"/Custom_Geoprocessing_Tools.tbx");
String toolName = "CustomDeleteFeatures";
String wrapperPackageName = null;
String toolboxName = "Custom_geoprocessing_tools";
String toolboxLocation = getOutputDir();
String metaDataFileDir = null;
String wrapperOutputLocation = getCurrentSrcDir();
ToolGenerator.generateTool(toolName, wrapperPackageName, toolboxName,
toolboxLocation, metaDataFileDir, wrapperOutputLocation);
}
catch (Exception e){
// TODO Auto-generate the catch block.
e.printStackTrace();
}
}
private String getOutputDir(){
String userDir;
if (System.getProperty("os.name").toLowerCase().indexOf("win") > - 1)
userDir = System.getenv("UserProfile");
else
userDir = System.getenv("HOME");
String outputDir = userDir + "\\arcgis_sample_output";
System.out.println("Creating output directory - " + outputDir);
new File(outputDir).mkdir();
return outputDir;
}
private String getCurrentSrcDir(){
//Get the fully qualified class name.
String fullyQualifiedClassName = this.getClass().getName();
String classFile = fullyQualifiedClassName.substring
(fullyQualifiedClassName.lastIndexOf(".") + 1) + ".class";
//Get the full path for the class file.
String pathIncludingClassFile = this.getClass().getResource(classFile)
.getPath();
//Get src dir from the full path of the class file.
String pathToSrcDir = null;
String osName = System.getProperty("os.name");
fullyQualifiedClassName = "bin/" + fullyQualifiedClassName.replace(".", "/");
if (osName.toLowerCase().startsWith("win")){
pathToSrcDir = pathIncludingClassFile.substring(1,
pathIncludingClassFile.lastIndexOf(fullyQualifiedClassName) - 1);
}
else{
pathToSrcDir = pathIncludingClassFile.substring(0,
pathIncludingClassFile.indexOf(fullyQualifiedClassName) - 1);
}
return fullyQualifiedClassName;
}
}
Publishing custom tool in Server
The toolbox that contains the custom geoprocessing tool can be published to the server and consumed in server applications in the same manner as other ArcGIS geoprocessing tools. The custom tools can also be included in Geoprocessing Models and published to ArcGIS server. Some of the related topics that you could refer on publishing the toolbox and model to the Server are listed below.
- Key Concepts for geoprocessing services
- Publishing Geoprocessing Services
- Creating Models for geoprocessing services
See Also:
How to consume custom tools in ArcToolboxDevelopment licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |
Server | Server |
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |