In this topic
- About building a custom geoprocessing tool in Eclipse IDE
- Create a Java project
- Auto-generate a geoprocessing tool implementation
- Specify the license requirements and create a help file
- Define the tool's operation
- Export the tool as a JAR file
- Deploy the tool
- Consume the tool
About building a custom geoprocessing tool in Eclipse IDE
Building a custom function tool requires implementing the following tasks:
For more information about creating custom function tool classes and custom function factory classes, see How to build custom geoprocessing tools.
In this topic, you'll build a custom geoprocessing function tool using the ESRI Geoprocessing Extension wizard in Eclipse IDE. The Geoprocessing Extension Wizard accepts user inputs and auto-generates code for the custom function factory class and the custom function tool class.
Prior to creating a custom geoprocessing tool using the Eclipse wizard, please ensure that:
- JDK version 1.6.30 is installed on your computer and <JDK Home>/bin is added to the PATH environment variable.
- The Java ArcObjects SDK 10.1 is installed on your computer. The SDK provides access to libraries required for developing ArcObjects Java extensions, as well ArcGIS Eclipse plugins that hold Java extension creation and export wizards.
- ArcGIS Eclipse plugins are installed in your copy of Eclipse. The minimum supported version of Eclipse at ArcGIS 10.1 is Helios Update 2 and Indigo Update 1. For instructions on how to install ArcGIS Eclipse plugins, see the "Using ESRI Eclipse Plugins" topic in "Developing Desktop Applications" -> "Using ESRI Eclipse Plugins" section of the ArcGIS Java Developer Help.
- A Java project is already created and the Arcobjects library is added to its build path. The Arcobjects library is available as part of the ArcGIS Eclipse plugins.
Auto-generate a geoprocessing tool implementation
To create both a geoprocessing tool Java class that defines the input and output parameters as well as the operation of the tool, and a custom function factory Java class that hosts the geoprocessing function tool in ArcToolbox using the Geoprocessing Extension wizard in Eclipse IDE, perform the following steps:
-
Open Eclipse IDE. With your Java project open, click File, click New, and click Other as shown in the following screen shot:
The New, Select a wizard dialog box opens.
-
Expand the ESRI Templates -> ArcGIS Extensions, select Geoprocessing Tool, and click Next as shown in the following screen shot:
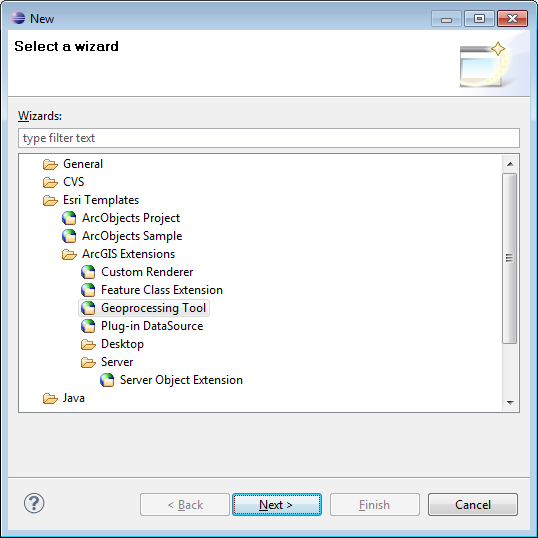
The New Geoprocessing Extension, Geoprocessing Tool dialog box opens.
-
Type the name, or browse to the source folder, of the Java project you created.
-
Type in or browse to the Java Package name.
-
Fill in the tool Name, Display Name, and Description parameters as shown in the following screen shot:
A Java class for the geoprocessing function tool is created in the source folder and package. -
To change the function factory default settings, click the Advanced tab, check the Rename Function Factory or use an existing Function Factory check box, and type in the function factory name to which the tool belongs. Click the browse button if the custom function factory already exists in the same project.
-
Type in the Toolset category and click Next as shown in the following screen shot:
The Add Geoprocessing Input Parameters dialog box opens.
The Delete Features tool can delete features from a feature class, a feature layer, or a shapefile. Features are deleted based on the SQL Expression you provide. The parameters for the Delete Features tool are as follows: - Input parameters
- in_features—A feature layer (.lyr) file or feature class from which the features will be deleted
- in_SQLExpression—The SQL expression dependent on the in_features parameter that queries the features to be deleted
- Output Parameter
- out_feature—The derived output feature class
- To create these parameters, in the Add Geoprocessing Input Parameters dialog box, click New as shown in the following screen shot:
The Parameter Properties dialog box opens. - Define the Name, Display Name, and Description parameter properties for in_features.
- For the Data Type parameter, click the browse button. The Geoprocessing Data Types dialog box opens so you can select the appropriate parameter values. The in_feature parameter accepts feature classes and feature layers.
- Select the DEFeatureClass and GPFeatureLayer values as shown in the following screen shot:
If you select more than one value for the parameter, the wizard automatically designates it a composite data type. For more information about data types, see Defining data types for tool parameters.
The Direction parameter property identifies whether the parameter is an input or an output parameter. Since you are creating input features, the wizard automatically identifies it as input. - Select Required from the Type drop-down box since in_features is a required parameter for execution of the tool. For more information about setting parameter properties, see Creating a custom function tool class.
- Click OK. The in_features parameter that you created is listed under Input Parameters as shown in the following screen shot:
- Click New to create another input parameter using the following parameter properties:
- Name: in_sqlExpression
- Display Name: Input SQL Expression
- Description: The SQL Expression to select the features to be deleted
- Data Type: GPSQLExpression
- Direction: Input
- Type: Required
- Dependency: in_Features (The SQL expression is dependent on the input features.)
- Click OK.
The Add Geoprocessing Input Parameters dialog box opens. - Click Next as shown in the following screen shot:
The Add Geoprocessing Output Parameters dialog box opens. - Click New. See the following screen shot:
The Parameter Properties dialog box opens. - Fill in the parameter properties for the out_features output parameter as shown in the following screen shot:
The Direction property is defined as output by the wizard. This property is disabled on the dialog box and cannot be modified. The Type property is defined as derived because the out_features parameter is dependent on the in_features parameter. - Click OK. The Add Geoprocessing Output Parameters dialog box opens as shown in the following screen shot. The out_features parameter is now listed under Output Parameters.
- Click Next.
The Parameter Properties dialog box will look like the following screen shot:
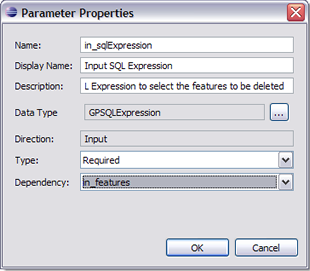
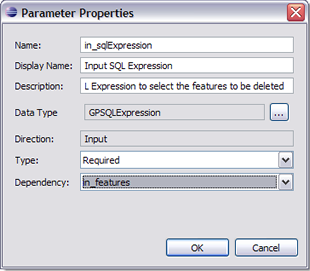
Specify the license requirements and create a help file
To specify the license required for your tool and to create a help file for your tool, perform the following steps:
- In the Specify help and license information dialog box, select the appropriate product license and extension license required for the operation of your tool.
- If necessary, modify the default tool help file name that will be created by the wizard at <EngineInstallDir>\help\gp.
- Click Finish.
These steps are shown in the following screen shot:
The wizard creates the custom geoprocessing function tool class and the custom function factory Java class in the specified Eclipse project based on your inputs. The wizard also auto-generates some of the method implementation based on those inputs as shown in the following screen shot:
The custom function tool class and the getName(), getDisplayName(), and getFullName() implementations are generated by the information you provided in the Geoprocessing Tool dialog boxes. The getParameterInfo() implementation is based on the input and output parameter properties you provided in the Add Geoprocessing Input Parameters and Add Geoprocessing Output Parameters dialog boxes. The getMetaDataFile() and isLicensed() implementations are based on the information you provided in the Specify help and license information dialog box.
The @ArcGISExtension annotation is added to your class definition. The auto-generated getName() method returns the function factory name that you provided. The getFunction() method returns the function tool associated with the factory and the getFunctionName() and getFunctionNames() methods create FunctionName objects based on the input tools created through the wizard.
Define the tool's operation
The Java Delete Features tool deletes features selected by the SQL Expression from the input features. The BaseGeoprocessingTool.execute() method defines a tool's operation. To define the Delete Features tool's operation, perform the following steps:
- Check for an overwrite output value since the output parameter (out_features) is a derived type parameter and it overwrites the input parameter data (in_features).
-
Unpack the parameter values.
-
Create a query based on the SQL Expression and delete the features.
These steps are shown in the following code:
[Java]
public class CustomDeleteFeatures extends BaseGeoprocessingTool{
//...
/**
* Execute the tool.
*/
public void execute(IArray paramvalues, ITrackCancel trackcancel,
IGPEnvironmentManager envMgr, IGPMessages messages){
try{
//Get the overwrite output value.
IGeoProcessorSettings gpEnv = new IGeoProcessorSettingsProxy(envMgr);
if (!gpEnv.isOverwriteOutput()){
messages.addError(esriGPMessageSeverity.esriGPMessageSeverityError,
"The Geoprocessing settings did not allow OverwriteOutput");
}
if (messages.getMaxSeverity() ==
esriGPMessageSeverity.esriGPMessageSeverityError){
return ;
}
else{
//Input features parameter.
IGPParameter inputFeaturesParameter = (IGPParameter)
paramvalues.getElement(0);
IGPValue inputFeaturesValue = this.gpUtilities.unpackGPValue
(inputFeaturesParameter);
//Retrieve the input as a feature class.
IFeatureClass[] ifc = new IFeatureClass[1];
ifc[0] = new IFeatureClassProxy();
this.gpUtilities.decodeFeatureLayer(inputFeaturesValue, ifc, null);
FeatureClass inputFeatureClass = new FeatureClass(ifc[0]);
//SQL Expression parameter.
IGPParameter expressionParameter = (IGPParameter)
paramvalues.getElement(1);
IGPValue expressionValue = this.gpUtilities.unpackGPValue
(expressionParameter);
String expression = expressionValue.getAsText();
messages.addMessage("\tDeleting selected feature(s)...");
//Delete the features.
Workspace wsEdit = null;
try{
wsEdit = new Workspace(inputFeatureClass.getWorkspace());
wsEdit.startEditing(false);
wsEdit.startEditOperation();
messages.addMessage(
"\nexecute() in DeleteFeatures: Num features before delete :" + inputFeatureClass.featureCount(null));
//Use the SQL Expression provided to determine the features to delete.
QueryFilter deleteFilter = new QueryFilter();
deleteFilter.setWhereClause(expression);
inputFeatureClass.deleteSearchedRows(deleteFilter);
messages.addMessage(
"\nexecute() in DeleteFeatures: Num features after delete : " + inputFeatureClass.featureCount(null));
wsEdit.stopEditOperation();
wsEdit.stopEditing(true);
}
catch (Exception e){
if (wsEdit != null && wsEdit.isBeingEdited()){
wsEdit.stopEditing(false);
}
e.printStackTrace();
}
}
}
catch (IOException e){
e.printStackTrace();
}
}
//...
}
The custom geoprocessing tool you created must be bundled as a Java Archive (JAR) file before it can be deployed to ArcGIS. Export the function factory Java class and the custom function tool Java class as a JAR file—for example, as custom_delete_features.jar.
For more information about creating a JAR file in Eclipse IDE, see How to export extension classes as a JAR file.
Deploy the tool
Before the custom tool is consumed in ArcGIS Engine, Desktop or Server, it's jar file must be deployed to the appropriate ArcGIS environment. To deploy the tool to ArcGIS Engine or Desktop, place the JAR file in the <ArcGIS Engine or Desktop Install Dir>\java\lib\ext folder and restart ArcGIS Engine/Desktop app.
If you wish to deploy the tool to ArcGIS Server 10.1, then the tool must first be deployed to ArcGIS Desktop, successfully executed and then published as a GP service to Server. However, before publishing the tool to ArcGIS Server as a service, the jar file containing the tool must be placed in <ArcGIS Server Home>/usr/lib/ext folder and Server must be restarted. If your ArcGIS Server site has multiple machines registered, the jar file must be placed manually in the /usr/lib/ext folder of all the Server machines. ArcGIS Server 10.1 does not automatically farm out GP tool jar files to Servers that are newly added to the Server site.
The following is the workflow for deploying a custom tool to Server:
- Deploy the tool to Desktop
- Execute it successfully.
- Click Geoprocessing menu -> Results. The Results window will open up.
- Expand Current Session. Right click on your custom GP tool (DeleteFeatures, in the screen shot below), and click "Share As -> Geoprocessing Service.
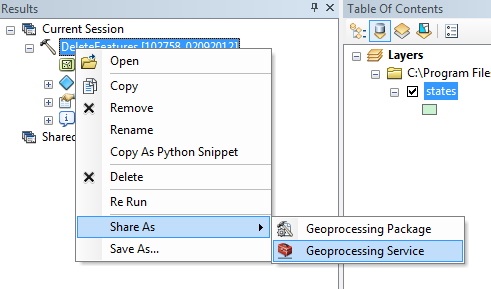
- Publish the tool as a GP service by following the publishing wizard. This workflow is common to custom and as well as System GP tools. For more details on this workflow, please consult the Geoprocessing documentation in ArcGIS Resource Center.
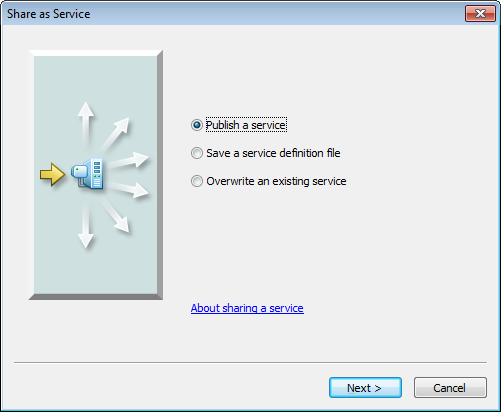
In general, the ArcGIS system recognizes a JAR file as a custom geoprocessing function tool when it (re)starts. Thus, the Engine, Desktop application or Server will recognize and register a GP tool in a jar file when its (re)started. Therefore, if the ArcGIS application or Server is already running, it must be restarted after the JAR file is placed in the well-known location (/java/lib/ext for Engine and Desktop and /usr/lib/ext for Server). In the case of testing, if you modify the code in the Java classes bundled in the JAR file, the JAR file must be recreated, redeployed and ArcGIS restarted.
Consume the tool
To learn how to consume a custom Java tool, please see the "Developing Extensions" -> "Custom Geoprocessing Tools" -> "Consume" -> "Consuming GP tools in ArcGIS" topic.
You can also test the tool by consuming it in a Java application. For more information, see How to consume custom geoprocessing tools in Java applications.
See Also:
How to build custom geoprocessing toolsCreating a custom function tool class
Creating a custom function factory
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |
Server | Server |
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |