- Engine
- ArcGIS for Desktop Basic
- ArcGIS for Desktop Standard
- ArcGIS for Desktop Advanced
- Server
Additional library information: Contents, Object Model Diagram
The Server library contains objects that allow you to connect and work with ArcGIS servers. Developers gain access to an ArcGIS server using the ServerConnection object. The ServerConnection object gives access to the ServerObjectManager. Using this object, a developer works with ServerContext objects to manipulate ArcObjects running on the server.
- The application programming interface (API) in the Server library can be used in any Java SE or Java EE container. Developers can build thin-client Web applications or Swing-based applications to invoke ArcObjects functionality on remote servers.
- The Server library is not extended by developers.
See the following sections for more information about this namespace:
Server connection objects
To use the geographic information system (GIS) server to host ArcObjects by your application, connect the application to the GIS server—connect to the server object manager (SOM). Connections to the GIS server are made through the ServerConnection object. The ServerConnection object supports a single interface (IServerConnection). IServerConnection has a Connect method that connects the application to the GIS server. Call the Connect method and provide the name or Internet protocol (IP) address of the machine on which the server object manager is running.
Once connected to the GIS server, IServerConnection has properties that hand out references to the ServerObjectManager and the ServerObjectAdmin for making use of server objects and administering server objects, respectively. See the following illustration:

To successfully connect to the GIS server using ServerConnection, the user account running the application must be a member of the agsusers group on the GIS server. If the account running the application is not a member of this group, the Connect method on IServerConnection throws an IOException. If the user account running the application is a member of the agsadmin user group on the GIS server, the ServerObjectAdmin property on IServerConnection can be used to get a reference on the ServerObjectAdmin. If the user account running the application is not in the agsadmin user group, the ServerObjectAdmin property throws an IOException. See the following illustration:
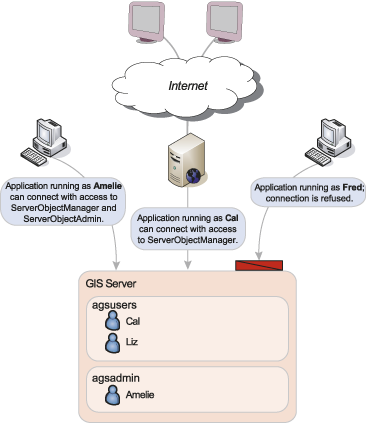
The following code example shows how to use the ServerConnection to connect to a GIS server running on a machine (husker) and print the names and types of the server object configurations configured on the server:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IEnumServerObjectConfigurationInfo servObjConfInfoEnum = null;
IServerObjectConfigurationInfo configurationInfo = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
// Initialize with <domain> <username> <password>...
serverInitializer.initializeServer(".", "admin", "admin");
try{
// Open connection to server.
connection = new ServerConnection();
connection.connect("capistrano");
// Get reference to ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
IServerContext context = som.createServerContext("usa", "MapServer");
MapServer ms = (MapServer)context.getServerObject();
System.out.println("The map service has " + ms.getServerInfo
(ms.getDefaultMapName()).getMapLayerInfos().getCount() + " layers.");
context.releaseContext();
}
catch (AutomationException ae){
System.err.println("Caught AutomationException: " + ae.getMessage() + "\n");
ae.printStackTrace();
}
catch (IOException e){
System.err.println("Caught IOException: " + e.getMessage() + "\n");
e.printStackTrace();
}
Server consumer objects
When developing applications that connect to a GIS server, access objects running on the server and create objects in the server for your application's use. If the user your application runs is a member of the agsusers user group on the GIS server, that application can connect to the GIS server using the ServerConnection object and can get a reference to the set of objects that provide the ability for the application to use ArcObjects running on the server. See the following illustration:
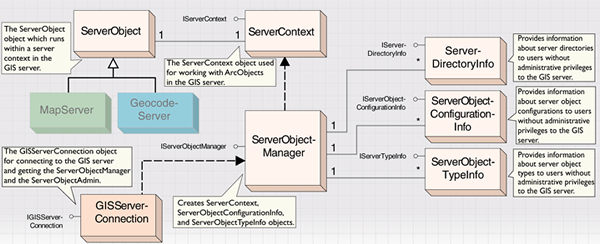
Server object manager
The ServerObjectManager class provides access to information about the GIS server to nonadministrators and creates ServerContexts for use by applications. Any application that runs as a user account in the agsusers user group on the GIS server can connect to the GIS server and get a reference to the ServerObjectManager. See the following illustration:
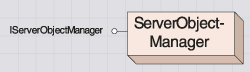
The ServerObjectManager is used for gaining access to and working with server objects. Each server object has a server object configuration. The IServerObjectManager interface has the necessary methods for an application to get the collection of server object configurations, server object types, and server directories configured in the server as ServerObjectConfigurationInfo, ServerObjectTypeInfo, and ServerDirectoryInfo objects, respectively.
The CreateServerContext method on IServerObjectManager is used to get a reference to a context on the server. A context is a process managed by the server where a server object runs. You can use CreateServerContext to create a context based on a server object configuration or you can create empty contexts to create ArcObjects on-the-fly in the server.
When using CreateServerContext to create a context based on a server object configuration, if the server object configuration is pooled, you can get a reference to a context that is already created and running on the server. When you have completed using that context, it is important to release it explicitly by calling the ReleaseContext method on IServerContext to return it to the pool. When using CreateServerContext to create a context based on a nonpooled server object configuration, or when creating an empty context, a new context is created on the server. Call ReleaseContext when you are finished using it and the context is destroyed on the server. See the following illustration:
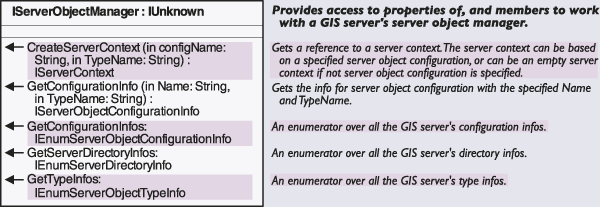
The following code example shows how to connect to the GIS server (husker), create a server context based on the RedlandsMap server object configuration, get a reference to the USA MapServer object running in the context, and print its DefaultMapName property. Note the call to ReleaseContext when the use of the context is complete.
[Java]
//Open connection to server.
connection = new ServerConnection();
connection.connect("husker");
//Get reference to ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
IServerContext context = som.createServerContext("USA", "MapServer");
MapServer ms = (MapServer)context.getServerObject();
System.out.println(ms.getDefaultMapName());
context.releaseContext();
The following code example shows how to create an empty server context and within that context, create a new polygon and print its area. Note the call to ReleaseContext when the use of the context is complete:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IEnumServerObjectConfigurationInfo servObjConfInfoEnum = null;
IServerObjectConfigurationInfo configurationInfo = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
// Initialize with <domain> <username> <password>...
serverInitializer.initializeServer(".", "admin", "admin");
//Open connection to server.
try{
connection = new ServerConnection();
connection.connect("capistrano");
// Get reference to ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
IServerContext context = som.createServerContext("", "");
// Create a new polygon in the server context.
Polygon ptCol = (Polygon)context.createObject(Polygon.getClsid());
// Create the points in the server context.
Point pt0 = (Point)context.createObject(Point.getClsid());
Point pt1 = (Point)context.createObject(Point.getClsid());
Point pt2 = (Point)context.createObject(Point.getClsid());
Point pt3 = (Point)context.createObject(Point.getClsid());
pt0.putCoords(0.0, 0.0);
pt1.putCoords(10.0, 0.0);
pt2.putCoords(10.0, 10.0);
pt3.putCoords(0.0, 10.0);
// Add the points to the polygon.
ptCol.addPoint(pt0, null, null);
ptCol.addPoint(pt1, null, null);
ptCol.addPoint(pt2, null, null);
ptCol.addPoint(pt3, null, null);
IArea area = (IArea)ptCol;
System.out.println(area.getArea());
context.releaseContext();
}
catch (UnknownHostException e){
e.printStackTrace();
}
catch (AutomationException e){
e.printStackTrace();
}
catch (IOException e){
e.printStackTrace();
}
Server contexts
A server context is a reserved space in the server dedicated to a set of running objects. GIS server objects also reside in a server context. When developing applications with ArcGIS for Server, all ArcObjects that your application creates and uses reside in a server context. To obtain a server object, get a reference to its context and get the server object from the context. You get a server context by calling the CreateServerContext method on IServerObjectManager. See the following illustration:
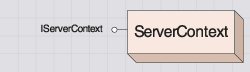
IServerContext contains methods for creating and managing objects running in the ServerContext. You can get at the server object running in a server context by calling GetServerObject on IServerContext.
The following code example shows creating a server context and getting a reference to the MapServer server object running in the server context:
[Java]
connection = new ServerConnection();
connection.connect("husker");
// Get reference to ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
IServerContext context = som.createServerContext("USA", "MapServer")MapServer ms =
(MapServer)context.getServerObject();
You can also create empty server contexts. You can use an empty context to create ArcObjects on-the-fly on the server to do ad hoc GIS processing.
All ArcObjects that your application uses should be created in a server context using the CreateObject method on IServerContext. Also, objects that are used together should be in the same context. For example, if you create a Point object to use in a spatial selection to query features in a feature class, the point should be in the same context as the feature class.
ArcGIS for Server applications should not use new to create ArcObjects but should always create objects by calling CreateObject on IServerContext. See the following code example:
[Java]
//Incorrect:
Point pt = new Point();
//Correct:
Point pt0 = (Point)context.createObject(Point.getClsid());
When your application is finished working with a server context, release it back to the server by calling the ReleaseContext method. If you allow the context to go out of scope without explicitly releasing it, it remains in use and is unavailable to other applications until it is garbage collected. Once a context is released, the application cannot make use of any objects in that context. This includes both objects that you obtained from the context or objects that you created in the context. See the following code example:
[Java]
// Get reference to ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
IServerContext context = som.createServerContext("USA", "MapServer");
// Do something with the object.
doSomething();
// Release the context when finished doing something.
context.releaseContext();
IServerContext provides useful methods that allow you to create and manage GIS objects within the context that can be used in your applications. See the following code example:
[Java]
IServerContext context = som.createServerContext("", "");
// Create a new polygon in the server context.
Polygon ptCol = (Polygon)context.createObject(Polygon.getClsid());
The CreateObject method returns a proxy to the object that is in the server context. Your application can make use of the proxy as if the object were created locally within its process. If you call a method on the proxy that hands back another object, that object will be in the server context and your application will be handed back a proxy to that object. In the preceding code example, if you get a SpatialReference object from the polygon using GetSpatialReference() , the object returned will be in the same context as the polygon.
In this case, if you add points to a polygon using GeometryEnvironment.setPoints, create and use the multipoint in the same context as the polygon. See the following code example:
[Java]
Polygon poly = (Polygon)context.createObject(Polygon.getClsid());
Point pt1 = (Point)context.createObject(Point.getClsid());
Point pt2 = (Point)context.createObject(Point.getClsid());
Point pt3 = (Point)context.createObject(Point.getClsid());
Point pt4 = (Point)context.createObject(Point.getClsid());
GeometryEnvironment ge = (GeometryEnvironment)context.createObject
(GeometryEnvironment.getClsid());
pt1.setX( - 118.556);
pt1.setY(35.044);
pt2.setX( - 119.5);
pt2.setY(35.9);
pt3.setX( - 119.0);
pt3.setY(37.0);
pt4.setX( - 118.556);
pt4.setY(35.044);
IPoint[] pointArray = new IPoint[4];
pointArray[0] = pt1;
pointArray[1] = pt2;
pointArray[2] = pt3;
pointArray[3] = pt4;
//Insert the Points into the Polygon
ge.setPoints(poly, pointArray);
Do not directly use objects in a server context with local objects in your application and vice versa. You can indirectly use objects or make copies of them. For example, if you have a Point object in a server context, you can get its x,y properties and use them with local objects or use them to create a new local point. Don't directly use the point in the server context, for example, the geometry of a local graphic element object.
In the following code example, assume that objects with Remote in their names are objects in a server context:
[Java]
Point remotePoint = new Point(context.createObject(Point.getClsid());
// While objects with Local in their name are objects created locally.
Point localPoint = new Point();
You can't set a local object to a remote object. The following is incorrect:
[Java]
localPoint = remotePoint;
The following is also incorrect:
[Java]
Point localPoint = new Point(remotePoint);
Do not set a local object or a property of a local object to be an object obtained from a remote object. The following is incorrect.
[Java]
Point localPoint = (Point)remotePointCollection.getPoint(2);
When calling a method on a remote object, don’t pass in local objects as parameters:
The following is incorrect.
[Java]The following is incorrect.
sde4Workspace = remoteWorkspaceFactory.open(localPropertySet, 0);
Get simple data types (double, long, string, and so on) that are passed by value from a remote object and use them as properties of a local object. The following is OK.
[Java]
localPoint.setX(remotePoint.getX());
SetObject and GetObject allow you to store references to objects in the server context. A context contains a dictionary that you can use as a convenient place to store objects that you create within the context. This dictionary is valid as long as you keep the server context and it is emptied when you release the context. Use this dictionary to share objects created within a context between different parts of your application that have access to the context.
SetObject adds objects to the dictionary, and GetObject retrieves them. An object that is set in the context will be available until it is removed (by calling the Remove or RemoveAll method) or until the context is released. See the following code example:
[Java]
Polygon polygon = (Polygon)context.createObject(Polygon.getClsid());
context.setObject("myPolygon", polygon);
Polygon p = (Polygon)context.getObject("myPolygon");
Use the Remove and RemoveAll methods to remove objects from a context that have been set using SetObject. Once an object is removed, a reference cannot be made using GetObject.
If you do not explicitly call the Remove or RemoveAll methods, you cannot get references to objects set in the context after the context has been released. See the following code example:
context.remove("myPolygon");
The SaveObject and LoadObject methods serialize objects in the server context to strings, then deserialize them back into objects. Any object that supports IPersistStream can be saved and loaded using these methods. These methods allow you to copy objects between contexts. For example, if you use a GeocodeServer object to locate an address and you want to draw the point that GeocodeAddress returns on your map, copy the point into your MapServer's context. See the following code example:
[Java]
new ServerInitializer().initializeServer(domain, user, password);
ServerConnection connection = new ServerConnection();
connection.connect("husker");
IServerObjectManager som = connection.getServerObjectManager();
IServerContext scGC = som.createServerContext("PortlandGC", "GeocodeServer");
IServerContext scMap = som.createServerContext("PortlandMap", "MapServer");
GeocodeServer geocodeServer = (GeocodeServer)scGC.getServerObject();
PropertySet propSet = (PropertySet)scGC.createObject(PropertySet.getClsid());
propSet.setProperty("Street", "2111 Division St");
propSet.setProperty("Zone", "97202");
PropertySet results = (PropertySet)geocodeServer.geocodeAddress(propSet, null);
Object[] names = new Object[5];
Object[] values = new Object[5];
results.getAllProperties(names, values);
for (int i = 0; i < names.length; i++){
if (names[i] != null){
System.out.println(names[i]);
}
}
Object pt = results.getProperty("Shape");
// Save and load.
String pointString = scGC.saveObject(pt);
Point pointCopy = (Point)scMap.loadObject(pointString);
System.out.println(pointCopy.getX());
SaveObject and LoadObject
The following illustration shows the use of the SaveObject and LoadObject methods to copy objects between server contexts:
- The client application gets or creates an object within a server context.
- The application uses the SaveObject method on the object's context to serialize the object as a string that is held in the application's session state.
- The client application gets a reference to another server context and calls the LoadObject method, passing in the string created by SaveObject. LoadObject creates a new instance of the object in the new server context.
Another important use of these methods is to manage state in your application while making stateless use of a pooled server object. A good example of this is in a mapping application. The initial session state for all users is the same and is equal to the map description for the map server object. Each user can then change map description properties, such as the extent and layer visibility, which needs to be maintained in the user's session state. The application does this by saving a serialized map description as part of each user's session state. Using the serialized string representation allows the application to take advantage of the standard session state management facilities of the Web server.
The application uses the LoadObject and SaveObject methods to reconstitute the session's map description when it needs to make edits in response to user changes or when it needs to pass the map descriptor to the map server object to draw the map according to the user's specifications. See the following illustration:
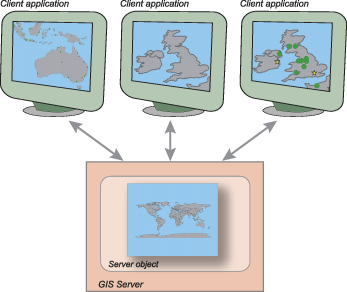
ServerObjects
A ServerObject is a coarse-grained ArcObjects component, which is a high-level object that simplifies the programming model for performing certain operations and hides the fine-grained ArcObjects that do the work. ServerObjects support coarse-grained interfaces that support methods that do large units of work, such as draw a map or geocode a set of addresses. ServerObjects also have Simple Object Access Protocol (SOAP) interfaces, which makes it possible to expose server objects as Web services that can be consumed by clients across the Internet. See the following illustration:
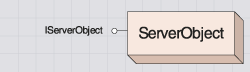
ArcGIS Server has the following types of ServerObjects:
-
MapServer—The MapServer object is a coarse-grained server object that provides access to the contents of a map document and methods for querying and drawing the map. See the following illustration:
-
GeocodeServer—The GeocodeServer object is a coarse-grained server object that provides access to an address locator and methods for a single address and batch geocoding. See the following illustration:
- GeodataServer—The GeodataServer object is a coarse-grained server object that provides access to a geodatabase and methods for data management.
- GlobeServer—The GlobeServer object is a coarse-grained server object that provides access to a globe document and methods for displaying the globe.
- GPServer—The GPServer object is a coarse-grained server object that provides access to one or more geoprocessing tools and methods for executing those tools in the GIS server.
Server objects and extensions can be used in ArcGIS for Server applications—the server object manager creates and manages instances of the server object and its extensions in processes running on the server. You get a reference to a server object running in the GIS server from its ServerContext.
IServerObject is an interface implemented by all server objects, such as the MapServer and GeocodeServer. The IServerObject is returned by the GetServerObject() method on IServerContext. The IServerObject interface has properties to indicate the name and type of the server object configuration that created the server object. You can cast the ServerObject to IMapServer for a MapServer object or IGeocodeServer for a GeocodeServer object.
The following code example shows how to connect to a GIS server, create a server context based on a server object configuration, and use the ServerObject property to get the IServerObject interface on the server context's server object:
[Java]
new ServerInitializer().initializeServer(domain, user, password);
ServerConnection connection = new ServerConnection();
connection.connect(host);
IServerObjectManager som = connection.getServerObjectManager();
IServerContext scGC = som.createServerContext("PortlandGC", "GeocodeServer");
GeocodeServer geocodeServer = (GeocodeServer)scGC.getServerObject();
// Do something with the GeocodeServer object.
scGC.releaseContext();
Server object extensions
Server objects can have extensions that extend their base functionality for more specialized uses. Each type of server object can have a set of extensions that can be enabled or disabled based on its configuration. For example, the MapServer object includes a network analysis server object extension that can be enabled for map servers that include network analysis layers and are intended for use in applications that include routing, service area, or some other network analysis type of functionality. ArcGIS for Server includes a number of out-of-the-box server object extensions, and developers can extend ArcGIS for Server by writing server object extensions.
Server objects extensions also have SOAP interfaces for handling SOAP requests to execute methods and returning results as SOAP responses. This support for SOAP request handling makes it possible to expose server object extensions as Web services that can be consumed by clients across the Internet.
ArcGIS Server includes the following server object extensions to the MapServer:
- NAServer extends the MapServer object to include network analysis functions for operations, such as routing and service area analysis.
- Mobile server extends the MapServer object to allow mobile devices to extract data from a map on the server.
- Web Map Service (WMS) extends the MapServer object to provide required capabilies to publish the MapServer as an Open Geospatial Consortium (OGC) WMS Web service.
- Keyhole Markup Language (KML) extends the MapServer object to return a stream of vector or raster layers to any client capable of reading zipped KML (KMZ) documents.
IServerObjectExtensionManager is an interface supported by all server objects. Once you have a reference to a server object, use the methods on IServerObjectExtensionManager to find enabled extensions by name (findExtensionByName) or by class identifier (CLSID); findExtensionByCLSID. Once you have a reference to the server object extension, you can query interface (QI) any of its interfaces.
The following code example shows how to get the nework analysis extension to a MapServer and find the network analysis layers in the map:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer(domain, user, password);
try{
//Open connection to the server.
connection = new ServerConnection();
connection.connect("mercy");
// Get reference to ServerObjectManager and the MapServer context.
IServerObjectManager som = connection.getServerObjectManager();
IServerContext context = som.createServerContext("SanFran_NA", "MapServer");
// Get the MapServer object and the server object extension.
MapServer ms = (MapServer)context.getServerObject();
IServerObjectExtensionManager soeManager = new
IServerObjectExtensionManagerProxy(ms);
IServerObjectExtension soe = soeManager.findExtensionByTypeName("NAServer");
INAServer naServer = (INAServer)soe;
// Get the NALayerNames.
String[] layers = naServer.getNALayerNames
(esriNAServerLayerType.esriNAServerClosestFacilityLayer);
context.releaseContext();
}
catch (Exception ae){
ae.printStackTrace();
}
Info classes
The Server object library's Info classes provide read-only access to users and developers who are not administrators to the properties of server directories, server types, and server object configurations. These properties are those that are necessary for developing applications using the GIS server.
Each Info class has a corresponding class that is only accessible to administrators (users in the agsadmin user group), which exposes the properties of the Info object with read/write access, as well as additional properties. See the following illustration:
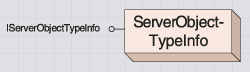
The ServerObjectConfigurationInfo class provides read-only access to some of the properties of a server object configuration. See the following illustration:
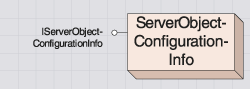
The ServerObjectTypeInfo class provides read-only access to some of the properties of a server object type.
A ServerDirectoryInfo object is an Info object that describes the properties of a server directory. See the following illustration:
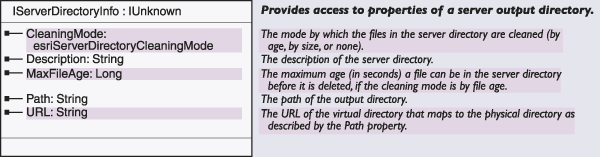
The GIS server manages a set of server directories. A server directory is a location on a file system that the GIS server is configured to clean up the files it writes. The ServerDirectoryInfo class gives users and developers who are not administrators access to the list of server directories and the set of their properties that are necessary for programming applications that use them as locations to write output. You can get information about server directories using the GetServerDirectoryInfos method on IServerObjectManager to get the IServerDirectoryInfo interface.
Files in a server directory can be cleaned based on file age or the last accessed date. The maximum file age or last accessed date is a property of a server directory. If the CleaningMode is esriDCAbsolute, all files created by the GIS server that are older than the maximum age are automatically cleaned by the GIS server. If the CleaningMode is esriDCSliding, all files created by the GIS server that have not been accessed for a duration defined by maximum age are automatically cleaned by the GIS server.
When creating files in a server directory, prefix the files to be cleaned by the GIS server with _ags_. Any files in a server directory not prefixed with _ags_ will not be cleaned. IServerDirectoryInfo provides read–only access to a subset of the server directory's properties. The following includes these properties:
- Path—The physical path of the directory on disk.
- URL—The uniform resource locator (URL) of the virtual directory corresponding to the physical directory.
- Description—The description of the server directory.
- CleaningMode—Indicates whether the directory is cleaned by file age, last accessed, or if its contents are not cleaned.
- MaxFileAge—Indicates the maximum age or last accessed date that files can be in the server directory before they are cleaned.
The preceding properties are necessary for developers of server applications to make use of the various GIS server directories.
The following code example shows how to list the server directories of a GIS server using the ServerDirectoryInfo class:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IEnumServerDirectoryInfo servDirInfoEnum = null;
IServerDirectoryInfo serverDirInfo = null;
// Initialize the server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to the ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
servDirInfoEnum = som.getServerDirectoryInfos();
serverDirInfo = servDirInfoEnum.next();
while (serverDirInfo != null){
System.out.println(serverDirInfo.getPath());
serverDirInfo = servDirInfoEnum.next();
}
}
catch (AutomationException ae){
System.err.println("Caught AutomationException: " + ae.getMessage() + "\n");
ae.printStackTrace();
}
catch (IOException e){
System.err.println("Caught IOException: " + e.getMessage() + "\n");
e.printStackTrace();
}
A ServerObjectConfigurationInfo object is an Info object that describes the properties of a server object configuration.
The GIS server manages a set of server objects running across one or more host (container) machines. How those server objects are configured and run is defined by a set of server object configurations. The ServerObjectConfigurationInfo class gives users and developers who are not administrators access to the list of server object configurations and properties that are necessary for programming applications. You can get information about server object configurations using the GetConfigurationInfos method on IServerObjectManager to get the IServerObjectConfigurationInfo interface. The GetConfigurationInfos method returns only server object configurations that are started.
IServerObjectConfigurationInfo provides read–only access to a subset of the server object configuration's properties. The following includes these properties:
- Name—The name of the server object configuration.
- TypeName—The type of server object configuration, for example, MapServer, GeocodeServer, and so on.
- Description—The description of the server object configuration.
- IsPooled—Indicates whether the server objects described by this configuration are pooled or nonpooled.
The preceding properties are necessary for developers of server applications to make use of the various server objects configured on the GIS server.
The following code example shows how to connect to a GIS server and use the IServerObjectConfigurationInfo interface to print the name and type of all the server object configurations:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IEnumServerObjectConfigurationInfo servObjConfInfoEnum = null;
IServerObjectConfigurationInfo serverObjectConfInfo = null;
// Initialize the server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get reference to the ServerObjectManager class.
IServerObjectManager som = connection.getServerObjectManager();
servObjConfInfoEnum = som.getConfigurationInfos();
serverObjectConfInfo = servObjConfInfoEnum.next();
while (serverObjectConfInfo != null){
System.out.println(serverObjectConfInfo.getName() + " : " +
serverObjectConfInfo.getTypeName());
serverObjectConfInfo = servObjConfInfoEnum.next();
}
}
catch (Exception ae){
ae.printStackTrace();
}
A ServerObjectTypeInfo object is an Info object that describes the properties of a server object type.
The ServerObjectTypeInfo class gives users and developers who are not administrators access to the necessary list of server object types and properties for programming applications. You can get information about server object types using the GetTypeInfos method on IServerObjectManager to get the IServerObjectTypeInfo interface.
IServerObjectTypeInfo provides read–only access to a subset of the server object type's properties. The following includes these properties:
- Name—The name of the server object type, for example, MapServer, GeocodeServer, and so on.
- Description—The description of the server object type.
Server administration objects
When developing applications that connect to a GIS server for the purposes of administering the GIS server and its server objects, get access to the objects for administering these aspects of the GIS server. If the user your application runs as is a member of the agsadmin user group on the GIS server, that application can connect to the GIS server using the GISServerConnection object and can get a reference to the set of objects that provide the ability for the application to administrate the GIS server and its server objects. See the following illustration:
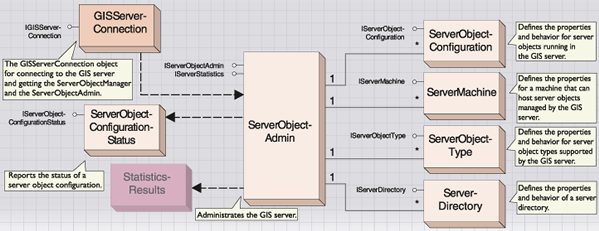
Applications that make use of ArcObjects running in the server do not require access to the administration objects.
The ServerObjectAdmin class administrates a GIS server. Any application that runs as a user account in the agsadmin user group on the GIS server can use the IGISServerConnection interface to connect to the GIS server and to get a reference to the ServerObjectAdmin. If the user account is not part of the agsadmin user group, the ServerObjectAdmin property on IGISServerConnection returns an error. Applications that are running as accounts that can connect to the server but are not part of the agsadmin user group can use the ServerObjectManager property on IGISServerConnection to get a reference on the ServerObjectManager. See the following illustration:
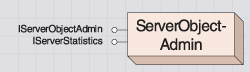
Use ServerObjectAdmin to administrate the set of server object configurations and types associated with the server and to administer aspects of the server. The following administration functionality of the GIS server is provided by ServerObjectAdmin:
Administer server object configurations:
- Add and delete server object configurations
- Update a server object configuration's properties
- Start, stop, and pause server object configurations
- Report the status of a server object configuration
- Get all server object configurations and properties
- Get all server object types and properties
Administer aspects of the server:
- Add and remove server container machines
- Get all server container machines
- Add and remove server directories
- Get all server directories
- Configure the server's logging properties
- Get statistics about events in the server
You can use IServerObjectAdmin to administrate the server's set of server object configurations, types, and aspects of the server, such as the list of machines that can host server objects. If your application is connecting to the server to make use of objects in the server, use the IServerObjectManager interface. See the following illustration:
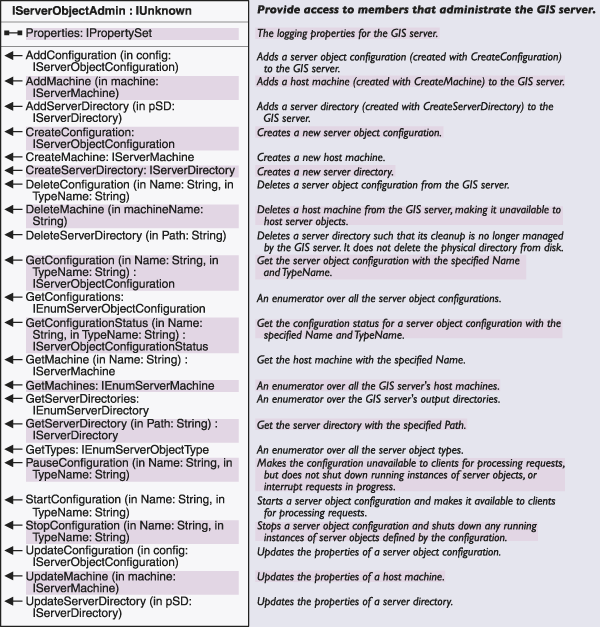
Administer server object configurations
The AddConfiguration method adds a ServerObjectConfiguration to your GIS server. A new ServerObjectConfiguration can be created using the CreateConfiguration method. Use the IServerObjectConfiguration interface to set the various properties of the configuration, then use the AddConfiguration method on IServerObjectAdmin to add the new configuration to the GIS server. Once a configuration is added to the server, use StartConfiguration to make it available for applications to use.
The following code example shows how to connect to the GIS server (grid10) and use the CreateConfiguration, AddConfiguration, and StartConfiguration methods to create a new geocode server object configuration, add it to the server, and make it available for use:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IServerObjectConfiguration serverObjectConf = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer(".", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("grid10");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
serverObjectConf = soa.createConfiguration();
serverObjectConf.setName("PortlandGC");
serverObjectConf.setTypeName("GeocodeServer");
IPropertySet props = serverObjectConf.getProperties();
props.setProperty("LocatorWorkspacePath",
"/arcgis/java/samples/data/ServerData/toxic");
props.setProperty("Locator", "portland_shp");
props.setProperty("SuggestedBatchSize", "500");
serverObjectConf.setIsPooled(true);
serverObjectConf.setMaxInstances(1);
serverObjectConf.setMinInstances(1);
serverObjectConf.setWaitTimeout(10);
serverObjectConf.setUsageTimeout(120);
// Add the configuration to the server.
soa.addConfiguration(serverObjectConf);
}
catch (Exception ae){
ae.printStackTrace();
}
The UpdateConfiguration method updates the specified ServerObjectConfiguration when the method is called. Use the GetConfiguration or GetConfigurations methods on IServerObjectAdmin to get a reference to the ServerObjectConfiguration you want to update.
The server object configuration must be stopped before you call UpdateConfiguration. You can use StopConfiguration to stop the server object configuration.
The following code example shows how to connect to the GIS server (husker) and use GetConfiguration to get a ServerObjectConfiguration, change its MaxInstances property, then update the configuration using the UpdateConfiguration method:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IServerObjectConfiguration serverObjectConf = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
serverObjectConf = soa.getConfiguration("PortlandMap", "MapServer");
serverObjectConf.setMaxInstances(3);
soa.updateConfiguration(serverObjectConf);
}
catch (Exception ae){
ae.printStackTrace();
}
Use DeleteConfiguration to delete a server object configuration from your GIS server.
To call DeleteConfiguration, stop the server object configuration. If it is not stopped, DeleteConfiguration returns an error.
The following code example shows how to stop, then delete a server object configuration (PortlandMap):
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
soa.stopConfiguration("PortlandMap", "MapServer");
soa.deleteConfiguration("PortlandMap", "MapServer");
}
catch (Exception ae){
ae.printStackTrace();
}
The ServerObjectConfiguration class describes the configuration for a server object that is managed by the GIS server. ServerObjectConfigurations can be added, removed, and modified by users or developers who are members of the agsadmin users group; therefore, have administrator privileges on the GIS server. See the following illustration:
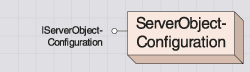
The following are the administrator level properties of ServerObjectConfiguration:
- Properties
- RecycleProperties
- MinInstances
- MaxInstances
- IsolationLevel
- StartupType
- WaitTimeout
- UsageTimeout
A read-only subset of properties of a ServerObjectConfiguration are available to nonadministrators via the GISServerConnectionInfo object. The following are the nonadministrator level properties:
- Name
- TypeName
- Description
- IsPooled
See the following illustration:
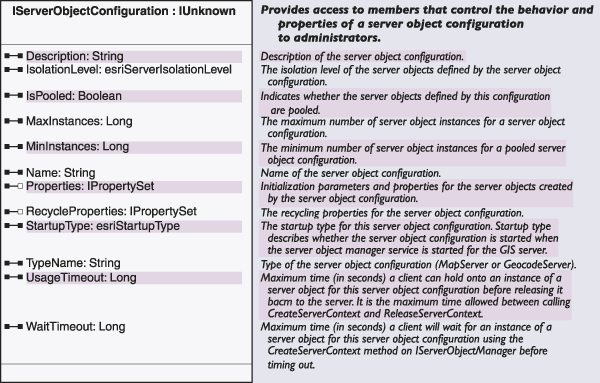
The IServerObjectConfiguration interface is a read/write interface on a server object configuration that allows administrators to configure new server object configurations to add to the server, update existing server object configurations, and view the configuration properties of a server object configuration.
If you use IServerObjectConfiguration to modify any of a configuration’s properties, call UpdateConfiguration on IServerObjectAdmin for the changes to be reflected on the server.
Use the IsolationLevel property to get the server object isolation, set it for a new configuration, or update an existing configuration.
Server objects can have high isolation (esriServerIsolationLevelHigh) or low isolation (esriIsolationLevelLow). Each instance of a server object with high isolation runs in a dedicated process on the server that it does not share with other server objects. Instances of server objects with low isolation can share the same process with other server object instances of the same configuration. Use the IsPooled property to indicate if the server objects created by this server object configuration are pooled or nonpooled.
Server objects can be pooled or nonpooled. Pooled server objects can be shared across multiple sessions and applications and are kept by an application for the duration of a single request. Pooled server objects are meant for applications that make stateless use of those objects.
Nonpooled server objects are dedicated to a single application session and are kept for the duration of an application session. Nonpooled server objects are not shared between application sessions and are meant for applications that make stateful use of those objects.
When StartConfiguration is called on a server object configuration whose IsPooled property is true, a set of server objects will be preloaded based on the MinInstances property of the server object configuration. When StartConfiguration is called on a server object configuration whose IsPooled property is false, no server objects are preloaded. Server objects are loaded and initialized when an application gets one from the server using CreateServerContext.
The MaxInstances property indicates the maximum number of server objects that can run and handle requests. If the maximum number of server objects are running and busy, additional requests will be queued until a server object becomes free.
For a pooled server object, the MaxInstances represents the maximum simultaneous requests that can be processed by the server object configuration. For a nonpooled server object, the MaxInstances represents the maximum number of simultaneous application users of that particular server object configuration. The MaxInstances property must be greater than 0 and must be greater than the MinInstances property. The MinInstances property applies to only pooled server object configurations. It represents the number of server object instances that are preloaded when the server object configuration is started. The GIS server ensures that the minimum number of instances are always running on the server for a configuration. When there are more simultaneous requests than server object instances running, additional server object instances start until MaxInstances is reached.
Nonpooled server object configurations always have a MinInstances property of 0. The MinInstances property must be less than the MaxInstances property. The Name property in combination with the TypeName property is used to identify a server object configuration in methods, such as GetConfiguration, UpdateConfiguration, StartConfiguration, and so on.
The Name property is case sensitive and can have a maximum of 120 characters. Names only contain the following characters:
- A–Z
- a–z
- 0–9
- _ (underscore)
- – (minus)
The TypeName property indicates the type of server object that this configuration creates and runs—examples are MapServer and GeocodeServer.
Server objects defined by server object configurations have an associated collection of initialization parameters and properties. An example of an initialization parameter is the map document associated with a MapServer object. An example of a property is the batch geocode size for a GeocodeServer object. You can get and change these properties using the Properties property on the server object configuration. The Properties property returns an IPropertySet. Use GetProperty and SetProperty on IPropertySet to get and set these properties. If you change these properties, call UpdateConfiguration to change the properties in the server object configuration.
You also use the Properties property to get a reference on the PropertySet for a new server object configuration to set its properties before adding it to the server by calling AddConfiguration.
Recycling allows for server objects that have become unusable to be destroyed and replaced with fresh server objects and to reclaim resources taken up by stale server objects.
Pooled server objects are typically shared between multiple applications and users of those applications. Through reuse, a number of things can happen to a server object to make them unavailable for use by applications. For example, an application may incorrectly modify a server object's state, or an application may incorrectly hold a reference to a server object, making it unavailable to other applications or sessions. In some cases, a server object may become corrupted and unusable.
Recycling keeps the pool of server objects fresh and removes stale or unusable server objects. You can get and change the recycling properties using RecyclingProperties on the server object configuration. RecyclingProperties returns an IPropertySet. Use GetProperty and SetProperty on IPropertySet to get and set these properties. If you change these properties, call UpdateConfiguration to change them in the server object configuration.
The following are the properties associated with recycling:
- StartTime—The time the recycling interval is initialized. The time specified is in 24-hour notation. For example, to set the start time at 2:00 p.m., the StartTime property is 14:00.
- Interval—The time between recycling operations in seconds. For example, to recycle the configuration every hour, this property is 3600.
The StartupType indicates if the configuration is automatically started (esriSTAutomatic) when the server object manager window's service is started. Server object configurations that are not configured to start automatically (esriSTManual) must be started manually using ArcCatalog or by calling the StartConfiguration method on IServerObjectAdmin.
The time it takes between a client requesting a server object (using the CreateServerContext method on IServerObjectManager) and getting a server object is the wait time. A server object can be configured to have a maximum wait time by specifying the WaitTimeout property on IServerObjectConfiguration. If a client's wait time exceeds the maximum wait time for a server object, the request times out. The WaitTimeout property is in seconds.
Once a client gets a reference to a server object, it can hold that server object before releasing it. The amount of time between when a client gets a reference to a server object and when it is released is the usage time. To ensure that clients don't hold references to server objects too long (for example, don't correctly release server objects), a server object can be configured to have a maximum usage time by specifying the UsageTimeout property on IServerObjectConfiguration. If a client holds a server object longer than the maximum usage time, the server object is automatically released and the client loses the reference to the server object. The UsageTimeout is in seconds.
Administer server machines
ArcGIS for Server is a distributed system. Server objects managed by the GIS server can run on one or more host machines. A machine that can host server objects must have the server object container (SOC) installed and the machine must be added to the list of host machines managed by the SOM.
Use the AddMachine method to add new host machines to the GIS server. Once a machine has been added to the GIS server as new server object instances are created, the SOM uses the new machine. Use the CreateMachine method to create a new server machine to pass as an argument to the AddMachine method and add new host machines to the GIS server.
Use the GetMachines method to get the names of the machines that have been added to the server to host server objects.
The DeleteMachine method removes a machine from the machines that can host server objects for the GIS server. When you delete a machine, any instances of server objects running on that machine shuts down and is replaced with instances running on the GIS server's other host machines.
The ServerMachine class is used to define a machine that can host server objects managed by the GIS server. See the following illustration:
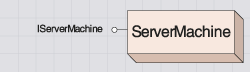
IServerMachine configures the properties of a machine to add it to the GIS server. Set the Name property for the machine (becomes the name of the machine on the network). The description is optional. See the following illustration:

Use the AddMachine method to add new machines to the GIS server. All server objects configured in the GIS server can run on any of the host machines; therefore, all host machines must have access to the necessary data and output directories used by all the server objects.
The following code example shows how to use the IServerMachine interface and AddMachine method to add a machine to the GIS server:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IServerMachine machine = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
// Create and add a new machine to the ServerObjectAdmin.
machine = soa.createMachine();
machine.setName("herbie");
soa.addMachine(machine);
}
catch (Exception ae){
ae.printStackTrace();
}
Administer server directories
Use IServerObjectAdmin to add and remove server directories from the GIS server. Both server objects and server applications need to write temporary or result data to a location for it to be delivered or presented to the end user. For example, a map server object's ExportMapImage method can create an image file that displays on a Web application (these files are usually transient and temporary). For example, when a map server writes an image to satisfy a request from a Web application, that image is only needed for the time it takes to display on the Web application. An application that creates checked out personal geodatabases for download provides a finite amount of time during which that geodatabase is created and when it can be downloaded.
Because server applications support many user sessions, these output files can accumulate and need to be periodically cleaned. The server provides the capability to automatically clean these output files if they are written to one of the server's output directories.
Use the CreateServerDirectory method to create a new server directory to pass as an argument to the AddServerDirectory method and add new server directories to the GIS server. Once you have added the server directory, configure your server objects and server applications to use the server directory.
Server directories must be accessible by all host machines configured in the GIS server.
The following code example shows how to use the CreateServerDirectory and AddServerDirectory methods to add a directory to the GIS server:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IServerDirectory dir = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
// Create and add a new directory to the server.
dir = soa.createServerDirectory();
dir.setCleaningMode(esriServerDirectoryCleaningMode.esriSDCSliding);
dir.setDescription("Default output directory");
dir.setPath("C:\\Inetpub\\wwwroot\\ArcGIS");
dir.setURL("http://husker:8399/ArcGIS");
dir.setMaxFileAge(100);
soa.addServerDirectory(dir);
}
catch (Exception ae){
ae.printStackTrace();
}
The UpdateServerDirectory method updates the ServerDirectory that is specified when the method is called. Use the GetServerDirectory or GetServerDirectories methods on IServerObjectAdmin to get a reference to the ServerDirectory you want to update.
The UpdateServerDirectory is useful for modifying the cleanup mode (CleaningMode) and cleanup schedule.
The following code example shows how to connect to the GIS server (husker) and use the GetServerDirectory method to get a ServerDirectory, change its MaxFileAge property, then update the directory using the UpdateServerDirectory method:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
IServerDirectory dir = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
// Get a server directory and change the MaxFileAge.
dir = soa.getServerDirectory("C:\\Inetpub\\wwwroot\\ArcGIS");
dir.setMaxFileAge(500);
soa.updateServerDirectory(dir);
}
catch (Exception ae){
ae.printStackTrace();
}
The DeleteServerDirectory method removes a directory from the set of directories managed by the GIS server. The DeleteServerDirectory method will not affect the physical directory.
When a server directory is removed with this method, the GIS server will not manage the cleaning of output files written to that directory. Applications or server objects that are configured to write output to the physical directory that is referenced by the server directory continue to work, but the written files will not be cleaned by the server.
The ServerDirectory class defines the properties of a server directory. A server directory is a location on a file system that the GIS server is configured to clean the written files. By definition, a server directory can be written to by all container machines. See the following illustration:
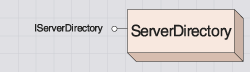
The GIS server hosts and manages server objects and other ArcObjects for use in applications. In many cases, the use of those objects requires writing output to files. For example, when a map server object draws a map, it writes images to disk on the server machine. Other applications can write data, for example, an application that checks out data from a geodatabase can write the checked out personal geodatabase to disk on the server.
Typically, these files are transient and need to be available to the application for a short period, for example, the time for the application to draw the map or the time required to download the checked out database. As applications process and write data, these files can accumulate quickly. The GIS server automatically cleans its output if that output is written to a server directory.
Files in a server directory can be cleaned based on file age or last accessed date. The maximum file age or last accessed date is a property of a server directory. If the CleaningMode is esriDCAbsolute, all files created by the GIS server that are older than the maximum age are automatically cleaned by the GIS server. If the CleaningMode is esriDCSliding, all files created by the GIS server that have not been accessed for a duration defined by maximum age are automatically cleaned by the GIS server.
When creating files in a server directory, prefix the files to be cleaned by the GIS server with _ags_. Any files in a server directory not prefixed with _ags_ will not be cleaned. See the following illustration:
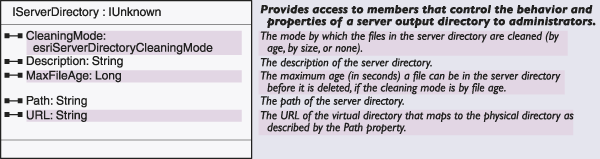
The IServerDirectory interface configures the properties of a server directory to add it to the GIS server. Set the Path, CleaningMode, and MaxFileAge (if cleaning mode is absolute or sliding) properties for the server directory, which will be the directories' path on disk. The Description and URL properties are optional.
The URL property is the virtual directory that corresponds to the physical directory specified by the Name property. Server objects, such as a map server object, can use the Name property to write output files to a directory where they will be cleaned and can pass back to clients the URL for the location of the written files. Clients, for example, Web applications will not require direct access to the physical directory.
Use the AddServerDirectory method on IServerObjectAdmin to add the new server directory to the GIS server.
Administer server logging and time-out properties
The Properties property on IServerObjectAdmin returns the properties for the GIS server. The properties are for the GIS server's logging and for server object creation timeout.
The GIS server logs its activity, including server object configuration startup, shutdown, server context creation and shutdown, and errors generated through failed operations or requests in the GIS server.
You can control the logging properties through the PropertySet returned by Properties. The following is a description of the logging properties:
- LogPath—The path to the location on disk to which log files are written. By default, the LogPath is /log.
- LogSize—The size to which a single log file can grow (in megabytes) before a new log file is created. By default, the LogSize is 10.
- LogLevel—A number between 0 and 5 indicating the level of detail that the server logs. By default, the LogLevel is 3. The following is a description of each log level:
- 0 (None)—No logging
- 1 (Error)—Serious problems that require immediate attention
- 2 (Warning)—Problems that require attention
- 3 (Normal)—Common administrative messages of the server
- 4 (Detailed)—Common messages from a user's use of the server, including server objects
- 5 (Debug)—Verbose messages to aid in troubleshooting
All aspects of logging can be changed when the GIS server is running. When they are changed, the server immediately uses the new logging settings.
The following code example shows how to use the Properties property on IServerObjectAdmin to modify the logging properties of the GIS server:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer("husker", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("husker");
// Get a reference to ServerObjectAdmin.
IServerObjectAdmin soa = connection.getServerObjectAdmin();
IPropertySet props = soa.getProperties();
props.setProperty("LogPath", "c:/ServerLogs");
props.setProperty("LogLevel", new Integer(5));
soa.setProperties(props);
}
catch (Exception ae){
ae.printStackTrace();
}
Server object creation can hang for many reasons. To prevent this from adversely affecting the GIS server, it has a ConfigurationStartTimeout property that defines the maximum time in seconds a server object instance has to initialize before its creation is cancelled.
Get statistics about events in the server
As the GIS server creates and destroys server objects, handles client requests and so on, statistics about these events are logged in the GIS server's logs. In addition to the log, statistics are also kept in memory and can be queried using the IServerStatistcs interface. See the following illustration:
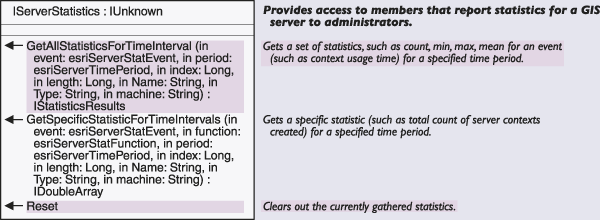
ArcCatalog provides administrators with a user interface for querying the GIS server's statistics. See the following screen shot:
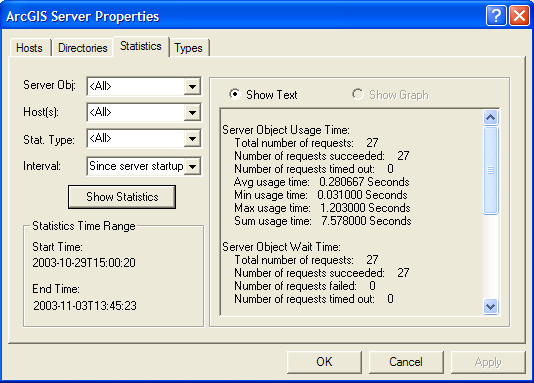
You can query the GIS server for statistics on the following events described by esriServerStatEvent. See the following table:
Value
|
Description
|
esriSSEContextCreated | A client made a call to CreateServerContext on IServerObjectManager and got a reference to a server context. |
esriSSEContextCreationFailed | CreateServerContext failed due to an error. Errors will be logged in the GIS server's log files. |
esriSSEContextCreationTimeout | CreateServerContext timed out because there were no available server objects for the requested configuration for a duration longer than the server object configuration's WaitTimeout. |
esriSSEContextReleased | A client released the server context by calling ReleaseServerContext. The time measured is the time the client held onto the context (the time between when they called CreateServerContext and receives a reference to the server context and the time they release the server context). |
esriSSEContextUsageTimeout | A client did not release the server context by calling ReleaseServerContext before the context's server object configuration's UsageTimeout was reached. |
esriSSEServerObjectCreated | A new server object was created. This can happen when a pooled configuration is started and the object pool is populated, when a server object is recycled, or in response to a call to CreateServerContext. The time measured is the time to create the server object. |
esriSSEServerObjectCreationFailed | The creation of a new server object instance failed due to an error. Errors will be logged in the GIS server's log files. |
You can query the following events using the statistical functions described by esriServerStatFunction:
- esriSSFCount
- esriSSFMinimum
- esriSSFMaximum
- esriSSFSum
- esriSSFSumSquares
- esriSSFMean
- esriSSFStandardDeviation
For esriSSEContextCreationFailed, esriSSEContextCreationTimeout, esriSSEContextUsageTimeout, and esriSSEServerObjectCreationFailed, the only relevant statistical function is esriSSFCount, as these events do not have time associated with them. The other functions reflect the statistics of the elapsed time associated with the event.
While the GIS server's logs maintain a record of all events in the server, the set of statistics in memory that can be queried are accumulated summaries of time slices since the GIS server was started. The granularity of these time slices is coarser the further back in time you go. These statistics can be queried for the following time intervals:
- By second for the current minute
- By minute for the current hour
- By hour for the current day
- By day for events that happened previous to the current day
Each time period is an accumulated total of the statistics for that time period. For example, if you query the total number of requests to create server contexts for the last 30 days, you would get statistics from now to the beginning of the day 30 days ago (not to the current time on that day). This is because the in-memory statistics have been combined for the entire day. This indicates that you can get statistics for a longer period than you specified in your query. When you query the GIS server for statistics, use the IServerTimeRange interface to get a report of the actual time period that your query results reflect.
The IServerStatistics interface has methods for querying a specific statistical function for an event or for querying all statistical functions for an event.
Use the GetSpecificStatisticForTimeIntervals method to query the GIS server for a specific statistic for an event at discrete time intervals. For example, use this method to get the count of all server contexts that were created for each minute of the last hour.
Use the GetAllStatisticsForTimeInterval to query the GIS server for all statistics for an event. For example, use this method to get the sum, mean, and so on, of server contexts usage time for the last two days.
Use these metods to query based on the events occurring in the server (that is, across all machines) or for those occurring on a specific machine. In addition, these methods can be used to query based on the events using all server objects or for events on a particular server object.
You specify the time interval for which you want to query using an index of time periods relative to the current time based on the time period described by esriServerTimePeriod. The index argument to the GetSpecificStatisticForTimeIntervals and GetAllStatisticsForTimeInterval methods describe the index of the time period to start from and the length argument describes the number of time periods to query.
For example, to query all statistics in the last two hours, specify a time period of esriSTPHour, an index of 0, and a length of 2.
To query all statistics since the server started, specify a time period of esriSTPNone, an index of 0, and a length of 1.
If you are unsure of the time period that the results of your query reflect, use the IServerTimeRange interface to get a report of the time period that your query results reflect. See the following illustration:

Use the Reset method to clear the statistics in memory.
The following code example shows how to query the GIS server for statistics on the create context event for all host machines and all configurations since the GIS server was started. It also shows the usage of the IServerTimeRange interface to report the time the statistics represent:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer(".", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("grid10");
// Get a reference to ServerObjectAdmin.
ServerObjectAdmin soa = new ServerObjectAdmin(connection.getServerObjectAdmin());
// Get the statistics.
ServerStatisticsResults stats = new ServerStatisticsResults
(soa.getAllStatisticsForTimeInterval
(esriServerStatEvent.esriSSEContextCreated, esriServerTimePeriod.esriSTPNone,
0, 1, "", "", ""));
System.out.println(stats.getCount() + " : " + stats.getStartTime() + " to " +
stats.getEndTime());
}
catch (Exception ae){
ae.printStackTrace();
}
The following code example shows the same query but for statistics for the last two hours:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer(".", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("grid10");
// Get a reference to ServerObjectAdmin.
ServerObjectAdmin soa = new ServerObjectAdmin(connection.getServerObjectAdmin());
// Get the statistics.
ServerStatisticsResults stats = new ServerStatisticsResults
(soa.getAllStatisticsForTimeInterval
(esriServerStatEvent.esriSSEContextCreated, esriServerTimePeriod.esriSTPHour,
0, 2, "", "", ""));
System.out.println(stats.getCount() + " : " + stats.getStartTime() + " to " +
stats.getEndTime());
}
catch (Exception ae){
ae.printStackTrace();
}
The following code example shows the query for statistics since the server started for the USA map server object:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer(".", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("grid10");
// Get a reference to ServerObjectAdmin.
ServerObjectAdmin soa = new ServerObjectAdmin(connection.getServerObjectAdmin());
// Get the statistics.
ServerStatisticsResults stats = new ServerStatisticsResults
(soa.getAllStatisticsForTimeInterval
(esriServerStatEvent.esriSSEContextCreated, esriServerTimePeriod.esriSTPNone,
0, 1, "USA", "MapServer", ""));
System.out.println(stats.getCount() + " : " + stats.getStartTime() + " to " +
stats.getEndTime());
}
catch (Exception ae){
ae.printStackTrace();
}
The following code example shows the query for the count of server contexts created for each minute in the last 60 minutes for all host machines and all server objects:
[Java]
ServerInitializer serverInitializer = null;
ServerConnection connection = null;
// Initialize server with impersonation information.
serverInitializer = new ServerInitializer();
serverInitializer.initializeServer(".", "admin", "admin");
try{
// Open the connection to the server.
connection = new ServerConnection();
connection.connect("grid10");
// Get a reference to ServerObjectAdmin.
ServerObjectAdmin soa = new ServerObjectAdmin(connection.getServerObjectAdmin());
// Get the statistics.
IDoubleArray dar = soa.getSpecificStatisticForTimeIntervals
(esriServerStatEvent.esriSSEContextCreated,
esriServerStatFunction.esriSSFCount, esriServerTimePeriod.esriSTPMinute, 0,
60, "", "", "");
for (int i = 0; i < dar.getCount(); i++){
System.out.println(dar.getElement(i));
}
}
catch (Exception ae){
ae.printStackTrace();
}