In this topic
About Dynamic Map Events
The Dynamic Map is an active Display, therefore the dynamic display changes in events like - dirty dynamic layer, map navigation that changes the visible bounds and/or, map refresh. The following Dynamic Map Events occur when the state of the dynamic map changes.
- IDynamicMapEvents.dynamicMapStarted : This event fired at the beginning of every draw cycle. This event will get fired even when the dynamic map is not redrawn.
- IDynamicMapEvents.beforeDynamicDraw & IDynamicMapEvents.afterDynamicDraw : The BeforeDynamicDraw\AfterDynamicDraw events are fired with two different phases (enum esriDynamicMapDrawPhase). Each phase has a different meaning and usage:
- esriDMDPDynamicLayers – This event is fired on each dynamic cycle that the Dynamic Map re-renders the dynamic content. The event is fired just before the first layer is rendered, and AfterDynamicDraw event is fired just after the last layer (including any Graphic Layer) is rendered. In this context, there is an active OpenGL Rendering context (with a preset rendering volume, viewport, etc). You can use this context to plug-in any custom dynamic drawings with the DynamicDisplay API as well as with the OpenGL API. This context can be useful in order to draw dynamic elements behind or on top of the Layers and Graphics, which does not need to be associated with a layer.
-
esriDMDPLayers – When the Dynamic Map is sensing that it needs to fetch new tiles in order to be able to render the base map(static content), the BeforeDynamicDraw event will get fired. When the last missing tile is available and rendered the AfterDynamicDraw event will get fired. It is invalid to use the DynamicDisplay API, nor the OpenGL API in this context, since the OpenGL Rendering Context is not fully setup.
You can use this context to monitor the process of fetching tiles for rendering the display. - IDynamicMapEvents.dynamicMapFinished : This event is fired at the end of every draw cycle, if the Dynamic Map is enabled. This event will get fired even when the dynamic map is not redrawn.
Listening to Dynamic Map Events
You can listen to dynamic Map events by attaching a listener to the dynamic map using the method map.addIDynamicMapEventsListener() and the listener can be removed using the method map.removeIDynamicMapEventsListener().
The following code snippet draws a simple marker at a random point by listening to dynamic map events.
[Java]
// The following class variables are created for the code snippet below
private boolean bOnce = true;
private int drawPhase;
private IDynamicDisplay dynamicDisplay;
private IDynamicGlyphFactory dynamicGlyphFactory;
private IDynamicSymbolProperties2 dynamicSymbolProps;
private IDynamicGlyph markerGlyph;
private IDisplay display;
private Map map;
//obtain the current map and add the listener to listen to events
//Check is the map is in dynamic mode
if (!map.isDynamicMapEnabled())
return ;
else{
map.addIDynamicMapEventsListener(new IDynamicMapEventsAdapter(){
public void dynamicMapStarted(IDynamicMapEventsDynamicMapStartedEvent event)
throws IOException, AutomationException{
System.out.println("Dynamic Map Started");
/*The IDynamicMapEvents.afterDynamicDraw() event is fired
* only when dynamic rendering takes place.
*Therefore to force this event in every draw cycle,
* the map is refreshed
*/
map.refresh();
}
public void beforeDynamicDraw(IDynamicMapEventsBeforeDynamicDrawEvent event)
throws IOException, AutomationException{
System.out.println("Before Dynamic Draw Event");
}
public void afterDynamicDraw(IDynamicMapEventsAfterDynamicDrawEvent event)
throws IOException, AutomationException{
//Draw a simple dynamic symbol at a random location in esriDMDPDynamicLayers phase
if (event.getDynamicMapDrawPhase() ==
esriDynamicMapDrawPhase.esriDMDPDynamicLayers){
dynamicDisplay = event.getDynamicDisplay(); display =
event.getDisplay();
//Create a simple marker from ICharacterMarkerSymbol
if (bOnce){
dynamicGlyphFactory = dynamicDisplay.getDynamicGlyphFactory();
dynamicSymbolProps = (IDynamicSymbolProperties2)
dynamicDisplay;
//Create a dynamic glyph from CharacterMarker symbol
ICharacterMarkerSymbol markerSymbol = new CharacterMarkerSymbol()
; markerSymbol.setCharacterIndex(72); markerSymbol.setSize
(25.0); IColor color = new RgbColor(); color.setRGB(0xFFFFFF)
; markerSymbol.setColor(color); markerGlyph =
dynamicGlyphFactory.createDynamicGlyph((ISymbol)markerSymbol)
; bOnce = false;
// Create glyphs only once, since they are expensive
}
// Set the properties of the dynamic Symbol
dynamicSymbolProps.setColor(esriDynamicSymbolType.esriDSymbolMarker,
1.0f, 0.0f, 0.0f, 1.0f); dynamicSymbolProps.setDynamicGlyphByRef
(esriDynamicSymbolType.esriDSymbolMarker, markerGlyph);
//Select a random point in visible bounds
IEnvelopeGEN visibleExtent = (IEnvelopeGEN)
display.getDisplayTransformation().getVisibleBounds(); Random r
= new Random(); double X = visibleExtent.getXMin() +
r.nextDouble() * visibleExtent.getWidth(); double Y =
visibleExtent.getYMin() + r.nextDouble() *
visibleExtent.getHeight(); Point point = new Point();
point.putCoords(X, Y);
//draw the marker on dynamic display
dynamicDisplay.drawMarker(point);
}
}
public void dynamicMapFinished(IDynamicMapEventsDynamicMapFinishedEvent
event)throws IOException, AutomationException{
System.out.println("Dynamic Map Finished");
}
}
;
}
Understanding Dynamic Map Events
The difference between the BeforeDynamicDraw\AfterDynamicDraw and the DynamicMapStarted\DynamicMapFinished events, is that the BeforeDynamicDraw\AfterDynamicDraw Events will get fired only when the Display (Dynamic Map) needs to be redrawn, while the DynamicMapStarted\DynamicMapFinished events will get fired on every Dynamic Map heart beat (while the Dynamic Map is Enabled), even when the display is not redrawn. The following sequences represents the events fired at different scenarios.
Scenario 1 : The DynamicMapStarted and DynamicMapFinished events are fired in every draw cycle, when the dynamic Map is enabled. See figure below:
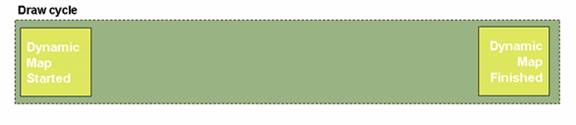
Scenario 2: The BeforeDynamicDraw and AfterDynamicDraw events in esriDMDPDynamicLayers phase are fired, when the dynamic map is enabled and whenever the dynamic layers are rendered. For example, when a dynamic layer is dirty. See figure below:
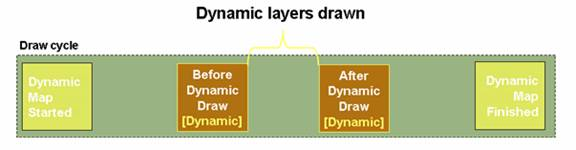
Scenario 3: The BeforeDynamicDraw and AfterDynamicDraw events are fired in esriDMDPLayers phase, when the dynamic map is enabled and when the underlying static layers(of the base map) are rendered as a result of map navigation(pan/zoom,roam), map refresh etc. See figure below:
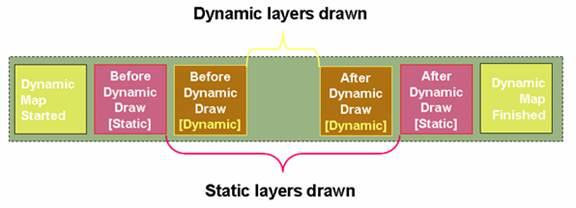
The dynamic layers are also rendered whenever the static layers(of the base map) are rendered irrespective of its dirty property.
This article explains the dynamic map events and how they could be used in a dynamic mode to render dynamic content. It also details the sequence of events that are fired under different scenarios such as, map navigation, map refresh or when a dynamic layer is dirty.
See Also:
Rendering Dynamic ContentDynamic Drawing APIs
Sample: Heads up display using OpenGL
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |