In this topic
- Integrated ArcObjects SDK
- Architectural modifications
- Supported platforms
- New features
- ArcGIS Engine concurrent licensing
- Customizing ArcGIS Desktop using add-ins
- New Eclipse plug-ins
- Server object extensions
- New environmental variables
- Map automation using Python
- Background execution of geoprocessing tools
- GraphicTracker for moving objects
- Basemap layers for improved map navigation and display
- Visualizing spatial datasets in ArcGIS
- ArcMap editing enhancements
- Display expressions for advanced MapTips
- Attribute-driven symbology
- PNG and alpha-blending bitmap support for icons
- New packages for arcobjects.jar
- Enhancement to existing packages
- arcmapui
- carto
- controls
- datasourcesraster
- display
- framework
- geodatabase
- geometry
- location
- networkanalyst
- schematic
- systemUI
- Deprecated or removed
Integrated ArcObjects SDK
At ArcGIS 10, there is a single software development kit (SDK) for ArcObjects. It combines the content previously contained in the ArcGIS Engine Java SDK along with ArcObjects development specific to ArcGIS Server. The ArcObjects SDK for Java includes documentation, samples, developer tools, and Eclipse plug-ins for ArcObjects development. It focuses on the following major categories of ArcObjects applications:
- Building extensions for ArcGIS Desktop
- Building stand-alone applications
- Developing server object extensions (SOEs) with ArcGIS Server
The included documentation has been enhanced with a reorganized table of contents (TOC) that focuses on the tasks developers want to accomplish.
Architectural modifications
ArcGIS 10 includes several significant product architecture modifications. One of the major advantages of the new architecture is autonomous ArcGIS Desktop and Engine runtimes. You can now install ArcGIS Desktop and ArcGIS Engine products in independent locations on your machine. You can also install service packs for Engine and Desktop products independently. However, these architectural changes require you to explicitly bind your stand-alone (Engine) applications and custom components to a specific ArcGIS product on your machine.
A stand-alone application can be bound to a Desktop or Engine runtime. In Eclipse, when you create a project and add the arcobjects.jar library to your classpath, Eclipse asks you which runtime to bind to, if both products are installed. Outside of Eclipse, the binding is achieved by including the following code example before using any ArcObjects.
For ArcGIS Engine, the following code example can be used to bootstrap the arcobjects.jar library:
[Java]
import java.io.File;
import java.lang.reflect.Method;
import java.net.URL;
import java.net.URLClassLoader;
public class Main{
public static void main(String[] args)throws Exception{
bootstrapArcobjectsJar();
ApplicationName.main(args);
//Replace ApplicationName with your application's main class.
}
public static void bootstrapArcobjectsJar(){
//Get the ArcGIS Engine runtime, if it is available.
String arcObjectsHome = System.getenv("AGSENGINEJAVA");
//If no runtime is available, exit application gracefully.
if (arcObjectsHome == null){
System.err.println(
"You must have the ArcGIS Engine Runtime installed in order to execute this application.");
System.err.println(
"Install the product above, then re-run this application.");
System.err.println("Exiting execution of this application...");
System.exit( - 1);
}
//Obtain the relative path to the arcobjects.jar file.
String jarPath = arcObjectsHome + "java" + File.separator + "lib" +
File.separator + "arcobjects.jar";
//Create a file.
File jarFile = new File(jarPath);
//Test for file existence.
if (!jarFile.exists()){
System.err.println(
"The arcobjects.jar was not found in the following location: " +
jarFile.getParent());
System.err.println(
"Verify that arcobjects.jar can be located in the specified folder.")
;
System.err.println(
"If not present, try uninstalling your ArcGIS software and reinstalling it.");
System.err.println("Exiting execution of this application...");
System.exit( - 1);
}
//Helps load classes and resources from a search path of URLs.
URLClassLoader sysloader = (URLClassLoader)ClassLoader.getSystemClassLoader()
;
Class < URLClassLoader > sysclass = URLClassLoader.class;
try{
Method method = sysclass.getDeclaredMethod("addURL", new Class[]{
URL.class
}
);
method.setAccessible(true);
method.invoke(sysloader, new Object[]{
jarFile.toURI().toURL()
}
);
}
catch (Throwable throwable){
throwable.printStackTrace();
System.err.println("Could not add arcobjects.jar to system classloader");
System.err.println("Exiting execution of this application...");
System.exit( - 1);
}
}
}
For ArcGIS Desktop (Windows machines only), use the following code example:
[Java]
import java.io.File;
import java.lang.reflect.Method;
import java.net.URL;
import java.net.URLClassLoader;
public class Main{
public static void main(String[] args)throws Exception{
bootstrapArcobjectsJar();
ApplicationName.main(args);
//Replace ApplicationName with your application's main class.
}
public static void bootstrapArcobjectsJar(){
//Get the ArcGIS Desktop runtime, if it is available.
String arcObjectsHome = System.getenv("AGSDESKTOPJAVA");
//If no runtime is available, exit application gracefully.
if (arcObjectsHome == null){
System.err.println(
"You must have ArcGIS Desktop installed in order to execute this application.");
System.err.println(
"Install the product above, then re-run this application.");
System.err.println("Exiting execution of this application...");
System.exit( - 1);
}
//Obtain the relative path to the arcobjects.jar file.
String jarPath = arcObjectsHome + "java" + File.separator + "lib" +
File.separator + "arcobjects.jar";
//Create a file.
File jarFile = new File(jarPath);
//Test for file existence.
if (!jarFile.exists()){
System.err.println(
"The arcobjects.jar was not found in the following location: " +
jarFile.getParent());
System.err.println(
"Verify that arcobjects.jar can be located in the specified folder.")
;
System.err.println(
"If not present, try uninstalling your ArcGIS software and reinstalling it.");
System.err.println("Exiting execution of this application...");
System.exit( - 1);
}
//Helps load classes and resources from a search path of URLs.
URLClassLoader sysloader = (URLClassLoader)ClassLoader.getSystemClassLoader()
;
Class < URLClassLoader > sysclass = URLClassLoader.class;
try{
Method method = sysclass.getDeclaredMethod("addURL", new Class[]{
URL.class
}
);
method.setAccessible(true);
method.invoke(sysloader, new Object[]{
jarFile.toURI().toURL()
}
);
}
catch (Throwable throwable){
throwable.printStackTrace();
System.err.println("Could not add arcobjects.jar to system classloader");
System.err.println("Exiting execution of this application...");
System.exit( - 1);
}
}
}
Supported platforms
For information on all Java supported platforms, and hardware and software requirements for the current release, see ArcObjects SDK System Requirements.
New features
The following subsections provide an overview of new features in ArcGIS 10.
ArcGIS Engine concurrent licensing
You can now obtain concurrent Engine licenses via a license server. Previous releases of ArcGIS Engine supported only a single-user, per machine license. The concurrent licensing model allows Engine applications to withdraw licenses from a server that can host a fixed number of licenses.
Customizing ArcGIS Desktop using add-ins
An add-in is a supplemental program that is simple to build, share, and deploy within ArcGIS Desktop applications (that is, ArcMap, ArcCatalog, ArcScene, and ArcGlobe). In addition, you can also develop add-ins to add custom functionality and special features to ArcGIS Desktop applications by accessing the underlying ArcObjects programmatically. After building an add-in, deploy them to a well-known folder location and the ArcGIS Desktop applications will recognize and consume them at runtime.
At ArcGIS Desktop 10, you can create the following types of add-ins using Java:
- Button—Simplest form of functionality that executes some business logic when the button is clicked.
- Tool—Requires user interaction with the application's display first, then executes some business logic based on the action.
- Combo box—Used to provide a set of choices from which a selection can be made and acted upon. A combo box can also allow the user to add a value that can be acted upon.
- Toolbar—Container for buttons or tools, menus, combo boxes, and tool palettes. Toolbars can be floating or docked in the aforementioned desktop applications.
- Menu—Container for buttons and additional menus.
- Tool palette—Container for tools.
- Dockable window—Can exist in a floating state or be attached to the main application window.
- Application extension—Provides users with additional geographic information system (GIS) functionality. Typically, functions that perform a specific task can be grouped into and made available through an extension.
New Eclipse plug-ins
To help expedite the development of ArcGIS Desktop add-ins, several new Eclipse plug-in wizards have been created at ArcGIS 10. For the add-in work previously described, this includes the follow new project wizards:
- ArcCatalog add-in project
- ArcGlobe add-in project
- ArcMap add-in project
- ArcScene add-in project
Each wizard guides you through all the required and optional components for extending any ArcGIS Desktop application.
ArcObjects code validator
Developers programming with ArcObjects need to be aware of a small number of classes and interfaces that are not amenable to Java style casting. In order to avoid ClassCastExceptions and subtle logical errors in your programs up until the release of ArcGIS 10, ESRI has published a list of such classes and interfaces. This list is tedious to consult for every ArcObjects used in an application to remove such potential errors. To address this problem and simplify a developer's experience, a plug-in for Eclipse can be used to detect statements using unsafe casting and runtime type checks, and provide suggestions to rectify such potential problems. The ArcObjects code validator can be used with existing or new projects at 10.
Server object extensions
SOEs are a custom components that can be developed in Java, .NET, and C++ to extend the ArcGIS Map Server Object. An SOE is created and initialized at the time the Server Object instance is created and is re-used at the request level, like the Server Object itself. If the SOE is costly to initialize, that cost is paid only once when the Server Object instance is created. Also, since an instance of an SOE remains alive as long as the Server Object's instance, it is able to cache information that can be re-used from request to request.
SOEs are best suited for applications that make several fine-grained ArcObjects calls between client and server processes. Developers can move the most expensive code to the SOE, thus reducing the number of fine grained calls between the client and server, and thereby increasing efficiency and responsiveness of client applications.
The following applies at ArcGIS 10:
- SOEs can be exposed as a Web Service and consumed through a Simple Object Access Protocol (SOAP).
- Eclipse integrated development environment (IDE) based tools are provided to generate standard Web service artifacts, such as Web Service Description Languages (WSDLs) and client stubs.
- SOEs support use of Java and ArcObjects types as parameters and return types in operations exposed by a SOE based Web service.
- Developers can extend from a base class that handles processing of SOAP requests and response for them.
- Developers do not need to explicitly create instances of an SOE in a context. The SOE is created by the GIS server when the Server Object instance is created.
- If the functionality that is exposed by an SOE can benefit from some caching logic, it is possible to implement such logic, because the cache remains for as long as the Server Object instance remains in the pool.
- SOEs can utilize ArcGIS Server log files to provide status and troubleshooting content, like other ArcGIS Server components.
- ArcGIS Server extensibility framework also allows developers to add custom behavior to their SOE at different points in its life cycle, so that their SOE has the following:
- Can have custom behavior when a ServerContext is created and released.
- Can have properties that can be modified to change the SOEs runtime behavior. These properties can be modified using ArcCatalog or ArcGIS Manager. For example, the SOE can be configured to work with a different layer in the associated MapServer object.
- Can have functionality declared in custom interfaces creating ArcObjects types to be implemented by SOEs.
- Can have "operations" that can be configured for access based on security rules.
New environment variables
With the release of previous versions of ArcGIS, the ARCGISHOME environment variable was used to determine the location of ArcGIS Engine runtime and SDKs. This environment variable operated under the assumption that all ArcGIS software was installed on one location on a given machine. At the release of ArcGIS 10, the Java ArcObjects SDK will not be required to be installed in the same location as ArcGIS Desktop or ArcGIS Engine runtimes. Thus, the ARCGISHOME environment variable has been updated and changed into the following new environment variables:
- AGSDEVKITJAVA—Points to the location of the developer kit install location on disk.
- AGSDESKTOPJAVA—Points to the location of ArcGIS Desktop install location on disk.
- AGSENGINEJAVA—Points to the location of ArcGIS Engine runtime install location on disk.
Map automation using Python
A new Python mapping module provides commands to interact with map (.mxd) documents and layer (.lyr) files. You can create Python scripts using these commands to open map documents and layers, query and alter contents, then print, export, or save the modified document. These commands are also useful for implementing multiple document workflows, such as updating and fixing data source locations, and for creating reports based on layers, data sources, or symbology. You can also combine several map documents to output a map book (similar to the functionality previously provided in the DS Map Book sample) or atlas to the printer or a .pdf file.
Background execution of geoprocessing tools
Since ArcGIS 9.2, developers have been able to run geoprocessing jobs (tools or models) using the IGeoprocessor2s execute() method, but the progress of the application was stalled during execution. At ArcGIS 10, the new IGeoprocessor2s executeASync() method can be used to execute geoprocessing jobs in the background of an application—which means the application can progress and respond to user interaction—while a geoprocessing job is being executed. You can also run more than one background geoprocessing job in an application. For more information, see How to run a geoprocessing tool in the background.
GraphicTracker for moving objects
The new GraphicTracker APIs allow you to visualize dynamic tracking of 100 moving objects on a two-dimensional (2D) map (.mxd) or three-dimensional (3D) globe (.3dd) without flickering. Each moving object can also be assigned a text label and optional text symbol. The GraphicTracker implementation minimizes refresh of display and provides smooth transition when a moving object is added to or removed from the display. Additionally, the moving object symbols can be made transparent, highlighted, oriented to x-, y-, and z-axes, or floated above the display according to the z-value. The object symbols can also be scaled based on the symbol's size or automatically shrunk when the display becomes large scale (bill-boarded).
Basemap layers for improved map navigation and display
A basemap layer is a special type of group layer for storing reference layers used for visualization or navigation purposes (for example, aerial imagery, streets, and land parcels). The basemap layer is drawn using optimized display logic and provides a continuous display that makes it easy to navigate around a map. You can create a basemap layer programmatically using the BasemapLayer class. A basemap layer can be added to or removed from a map document (.mxd file) the same way as any other layer.
Visualizing spatial datasets in ArcGIS
Temporal visualization is now supported in ArcGIS. Features layers, graphic layers, and other layers can be configured to render based on time attributes. Many of the layers can use existing time-related data that is properly formatted. Multiple layers can participate in time view at once. ArcGIS provides a time control user interface (UI) element, but the map can also be controlled programmatically.
ArcMap editing enhancements
The following editing enhancements are available:
- UI—The editing environment in ArcGIS Desktop has been redesigned to provide a more simplified and streamlined workflow. The workflows for the edit task, edit target, and sketch tools have been supplemented by templates, edit tools, construction tools, and shape constructors. The majority of changes and additions to the editor assembly support these user experience changes.
- Templates—Feature templates are a central concept in the editing environment in ArcGIS. Creating features using the editor relies on the use of feature templates. Feature templates provide an easy way to simplify and streamline the creation of features using the editor. Because a number of the properties required to set up the editing environment and attribute the feature are predefined, the user can easily select a template, and a number of steps are performed automatically making the user's task easier. For more information, see Using feature templates.
- Snapping—A new snapping environment that can be used by ArcMap and ArcGIS Engine developers is available. This snapping environment allows you to enable snapping in all your custom tools, not just those used with the editor.
-
TOC customizations—The TOC window and TOC views have been re-architected. TOC views, such as display and source, now appear as icons on the TOC toolbar rather than as tabs, as in previous versions. The TOC window is now also part of the dockable window framework allowing it to slide, pin, and dock with other windows in ArcGIS. Custom TOC views can be created by implementing the IContentsView3 interface, which exposes two additional methods including the getBitmap() method for the icon and a getToolTip() method, which returns a string.
Existing custom views should only be migrated to the new TOC framework if they directly manipulate or are associated with the layers on the map. If you were previously using the TOC framework to host custom views for their docking capability, you should create a proper dockable window instead. To migrate existing custom views to the new framework, implement the IContentsView3 interface in your class, and update the bitmap and ToolTip attributes using the methods previously mentioned.
Display expressions for advanced MapTips
A Display field is used to identify a feature when using the Identify window or to provide text for MapTips. You can now customize the text string of MapTips by creating a display expression using the IDisplayExpressionProperties interface. Using display expressions, you can concatenate or modify the attribute values of one or more fields, or include additional text strings to create more advanced MapTips. A display expression can also contain Visual Basic script or JavaScript to add logic and text processing for advanced MapTips.
Attribute-driven symbology
You can define the symbols for a feature layer based on its attribute value. See the following:
- Rotating point features—Existing rotation renderer has been improved to provide support for applying rotation to point features based on a rotation value specified in an attribute field or by using an expression. You can rotate points randomly within a 0-360 degree range. The IRotationRenderer2 interface provides methods for rotating points in maps, as well as in 3D views, such as scene and globe. In scene and globe, rotation is supported for the x-, y-, and z-axes. However, in map, the rotation is only supported in the 2D plane.
- Size of point features—Size renderer interface allows you to size point features based on a size value specified in an attribute field or by using an expression. You can also size points randomly within a range. The ISizeRenderer interface provides methods for sizing points in map, as well as in 3D views, such as scene and globe.
PNG and alpha-blending bitmap support for icons
Higher quality icons are supported that uses alpha-blending bitmaps and Portable Network Graphics (PNG) image formats. These icons are available when you install the ArcObjects SDK and can be used for developing commands and tools in both ArcGIS Engine and ArcGIS Desktop applications.
Enhancements to the Java-COM interop
The following is an enhancement made to the VisualBean mode of stand-alone applications:
New VisualBean mode architecture
In prior releases, the ArcGIS Java-COM interop relied upon Component Object Model (COM) marshalling to synchronize access to ArcObjects hosted in the ArcGIS single threaded apartment (STA) for Visual Beans applications. For an explanation of the old architecture, see Understanding Java-COM interop. In particular, refer to the section, Java interop and VisualBeans application.
At the release of ArcGIS 10, the internal architecture has been revamped to ensure thread affinity and reentrancy requirements of ArcObjects in multi-threaded visual Java applications without relying upon the services of the COM runtime (in particular, COM marshalling). In the new architecture, Java threads do not enter the process wide COM multiple threaded apartment (MTA). Instead, ArcObjects creation and method invocation is intercepted in the Java tier and performed in a dedicated STA thread created by the Java interop to house ArcObjects. The advantage of this new architecture is vastly improved performance for fine-grained methods calls on ArcObjects, and the ability to create and use ArcObjects that did not have marshalling related infrastructure (that is, proxy-stub dynamic-link libraries [DLLs] for components that did not rely upon type library marshalling).
At the release of ArcGIS 10, the internal architecture has been revamped to ensure thread affinity and reentrancy requirements of ArcObjects in multi-threaded visual Java applications without relying upon the services of the COM runtime (in particular, COM marshalling). In the new architecture, Java threads do not enter the process wide COM multiple threaded apartment (MTA). Instead, ArcObjects creation and method invocation is intercepted in the Java tier and performed in a dedicated STA thread created by the Java interop to house ArcObjects. The advantage of this new architecture is vastly improved performance for fine-grained methods calls on ArcObjects, and the ability to create and use ArcObjects that did not have marshalling related infrastructure (that is, proxy-stub dynamic-link libraries [DLLs] for components that did not rely upon type library marshalling).
Performance improvements
At ArcGIS 10, the performance of Java applications has been improved in the following significant ways:
- The new VisualBeans mode architecture eliminates the need for COM marshalling and vastly improves performance for fine grained ArcObjects calls in visual Java applications. This performance optimization is applied by default for all Java applications that have been initialized in "VisualBeans mode" (EngineIntializer.initializeVisualBeans();).
- An optimized mode of casting has been introduced. This optimized mode of casting uses metadata within arcobjects.jar to determine whether or not to attempt wrapping ArcObjects proxies into corresponding Java classes, thereby enabling normal Java casts and the use of the instanceof operator to succeed. Earlier, the Java-COM interop always attempted to wrap proxies into corresponding classes, even though the wrapping and pre-casting would have failed anyway. Your Java code needs to opt-in to take advantage of this optimized casting feature. To get optimized casting, set the runtime property before calling Engineinitializer with the following code example:
System.setProperty("ARCGIS_OPTIMIZED_CASTING", "");
EngineInitializer.initializeEngine();
You can also set this property using java -DARCGIS_OPTIMIZED_CASTING or by passing it in as a VM argument. A side effect of this benefit is that developers must pay careful attention in their use of the instanceof and casting operators when programming with ArcObjects. In particular, Java casts and instanceof operators will not work for ArcObjects that have a non-deprecated constructor (a constructor that takes an object as its only argument to convert that object to the type of that ArcObjects).
To help quantify some of the performance improvements, see the following illustrations that show the performance improvements observed in representative applications:
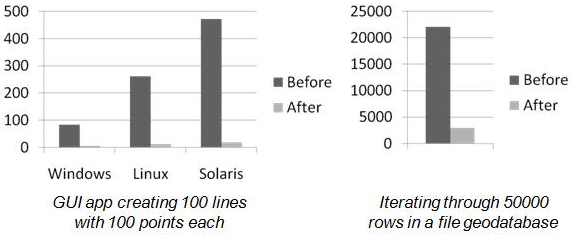
New packages for arcobjects.jar
The following new packages have been added to the arcobjects.jar file:
- com.esri.arcgis.enginecore—The engine core package contains APIs that allow you to build and manage collections of graphics in a map or globe using the GraphicTracker class.
- com.esri.arcgis.schematiccontrols—The schematic controls package contains the schematic control commands, which are a set of commands that works with the ArcGIS Engine controls and enable you to build schematic applications. All the schematic related commands in the com.esri.arcgis.controls package have been moved to schematic control commands.
Enhancements to existing packages
Various packages have been updated with new interfaces and classes. The following sections provide an overview of the key enhancements.
arcmapui
The ITableWindow3 interface has been added to the com.esri.arcgis.arcmapui package. This interface has three methods—isOpen(), findOpenTableWindows(), and getActiveWindowTable()—to interrogate active table windows in ArcMap.
carto
The MosaicLayer class for displaying mosaic datasets has been added to the com.esri.arcgis.Carto package.
controls
The following enhancements have been made to the com.esri.arcgis.controls package:
- A new ControlsFindRouteCommand class has been added. This command opens the Find Route dialog box, which lets you find routes between points using a variety of methods. You can find an optimized route, which is the most efficient travel route between the points you select. You can set the points or stops, by clicking the map, by adding them from a layer, or by geocoding them.
- In previous releases, an ArcGIS Engine Developer Kit license is (and has been) required to develop applications that embed ArcGIS Engine Java VisualBeans, such as SceneBean, GlobeBean, TOCBean, and ToolbarBean only. At ArcGIS 10, an ArcGIS Engine Developer Kit license is also required to develop applications using MapBean and PageLayoutBean as well.
datasourcesraster
The following enhancements have been made to the DataSources packages:
- The following classes that can manage a large collection of images and provide dynamic image processing capabilities are new at 10:
- The FunctionRasterDataset class is a special type of raster dataset that encapsulates RasterFunction (a class that describes the required arguments for the operations) together. When it is accessed, the FunctionRasterDataset creates an output raster at run time by applying the raster function to the input data, which provides a mechanism for dynamic raster processing. A set of raster function classes have been added that includes HillShadeFunction, ClipFunction, ConvolutionFunction, SlopeFunction, StretchFunction, ArithmeticFunction, and PanSharingFunction.
- MosaicDataset is a new raster data model that is used to manage a large collection of images with multiple formats and multiple sensors. It is supported in file geodatabases, personal geodatabases, and enterprise geodatabases. The RasterType class and a set of raster builder classes, such as LandsatBuilder, QuickBirdBuilder, and IkonosBuilder, have been developed to support various sensor types. A number of mosaic dataset operation parameter classes, including AddRasterParameters, BuildBoundaryParameters, and DefineOverviewsParameters, have been added to be used when authoring a mosaic dataset. (The MosaicLayer class, for displaying mosaic datasets, has also been added to the com.esri.arcgis.carto package).
- The following classes were added to support sensor camera models:
- LSRXform (local space rectangle transform)
- FrameXform (standard frame camera transform)
- The following interfaces have also been added:
- IRasterColormap3—Used to read and write color map files (.clr).
- IRawBlocks—Used to read and write pixel blocks based on storage blocks.
display
The following enhancement has been made to the com.esri.arcgis.display package:
- ServerStyleGalleryItem supports the IStyleGalleryItem2 interface. IStyleGalleryItem2 provides access to methods that define items in the style gallery. Symbols and map elements are stored in the style gallery. Symbol or map element tables within a style have a new field and tags that contain references to the graphic properties of each symbol. Tags can be read from the item in the style gallery using the getTags() method from IStyleGalleryItem2.
framework
The following interfaces have been added to support dockable windows:
- IDockableWindowInitialPlacement—Specifies where a dockview appears the first time it shows.
- IDockableWindowImageDef—Associates an icon with the dockview.
geodatabase
The following enhancements have been made to the com.esri.arcgis.geodatabase package:
- The workspace editing event model has been extended to include the IWorkspaceEditEvents2 interface. This interface exposes an event method, onBeginStopEditing(), that is triggered when client code calls the stopEditing() method on the IWorkspaceEdit interface and allows custom behavior to occur before the edit session has stopped. This is in contrast to the onStopEditing() method on the IWorkspaceEditEvents interface that is triggered after the edit session has already been stopped.
- IQueryDef2 interface has been added to allow for support of postfix clauses, such as Order By and Group By, through the postfixClause attribute; prefix clauses, such as Distinct, through the prefixClause attribute and recycling cursors, through the evaluate2() method. The IQueryFilterDefinition2 interface has also been added to query filters to support prefix clauses.
- Several triangulated irregular network (TIN) related interfaces, such as ITinAdvanced3, ITinEdit2, ITinImporter, and ITinSurface3, for data management and analysis have been added. New terrain related interfaces, such as IDynamicSurface3, ITerrain2, ITerrainDataSource2, ITerrainEdit3, ITerrainEmbeddedDataSource3, and ITerrainFieldStatistics, for data management and analysis have also been added.
- NetworkForwardStar—The INetworkForwardStarEx interface has been added to support the new barrier types (polygon, polyline, and point barriers that do not block the whole edge). INetworkForwardStarEx also centralizes the setup and querying used for network traversal that was previously spread across the INetworkForwardStar and INetworkForwardStarSetup interfaces.
- Network dataset—The DENetworkDataset class has a new IDENetworkDataset2 interface that allows you to specify new properties added to the network dataset, such as the elevation connectivity model and traffic data. There is a new NetworkEdgeTrafficEvaluator class that allows a network attribute to return travel time values that vary based on the time of day. The NetworkAttribute class has a new property that indicates whether the attribute is time aware and the network elements have new properties that allow you to query attribute values for a specific time of day. This might be of interest to developers using NetworkForwardStar.
geometry
The following enhancements have been made to the geometry library:
- The IConstructClothoid interface has been added to support clothoid curves. A clothoid curve (or Euler spiral) is often used in railway and highway engineering to allow gradual transition between tangents or circular curves. Also at ArcGIS 10, 3D polylines can support vertical line segments.
- The IPolycurveGeodetic and IConstructGeodetic interfaces have been added to support the construction of densified approximations of geodetic curves. These interfaces allow you to construct circles and ellipses that are defined using geodesic distance. Also, you can now compute distance between points on geodesic, loxodrome, great elliptic, or normal section curves.
- The IGeometryServer2 interface provides access to more standard operations on arrays of geometric value objects. Similar to IGeometryServer methods, the input geometries are never modified by these operations.
location
The following interfaces and classes have been added to the com.esri.arcgis.location package:
- ISingleLineAddressInput—Used to determine if a locator supports single line geocoding. It contains the singleLineAddressField attribute, which specifies the field that the locator uses to geocode addresses in a single line format. It also contains the defaultInputFieldNames attribute to determine which field in an address table contains the full address for single line geocoding.
- IGeocodingProperties2—Used to specify the end offset distance as a double and specify end offset distance units as esriUnits for locators that were built using ArcGIS 10 and above.
- ESRIGen2AddressLocator—A locator (class) that uses the ESRI geocoding engine introduced in ArcGIS 10. This address locator supports single line input for geocoding.
- IBatchGeocoding—Implemented by every locator and contains a subset of the methods that IAdvancedGeocoding contains. Use IBatchGeocoding instead of IAdvancedGeocoding when there is no need to access standardization methods or when a locator does not support standardization. IAdvancedGeocoding contains the rematchTable() method, while IAdvancedGeocoding2 contains the matchRecordSet() method.
networkanalyst
The following enhancements have been made to the com.esri.arcgis.networkanalyst package:
- Location-Allocation solver—A new Network Analyst (NA) solver, NALocationAllocationSolver, has been added. The Location-Allocation solver is often used to locate a set of warehouses to minimize transportation costs or to locate a set of locations, such as fire stations, to provide the best coverage in a specified travel time, or to locate retail outlets to best capture market shares in presence of competitor locations using a Huff model.
- Polygon and polyline barriers—To provide support for polygon and polyline barriers, NALocationRangesObject, NALocationRangesFeature, and NALocationRanges track the lists of junctions and edge ranges, with the associated geometry, that make up barriers. The INALocator3 and INALocatorAgent3 interfaces have been added to use shapes and rows from feature classes to generate the location ranges associated with polygon and polyline barriers. The INAClassDefinition2 interface has been added to the NAClassDefinition class to help you find the location range field in the barrier NAClasses.
- Locating—Sometimes, when locating analysis objects, it is important to exclude elements that are restricted due to network attributes or barriers. CacheRestrictedElements and ExcludeRestrictedElements have been added to INALocator3 to restrict the NALocator location queries from returning network locations that fall on restricted network elements.
schematic
The schematic package adopts a new architecture to simplify development and to take advantage of standard ArcGIS features. In the new architecture, the NGO engine which handled the schematic graphical core and formed the foundation for the in-memory schematic objects has been removed. Some of the significant improvements in the new architecture are:
- Database objects—All schematic elements are now stored as point, polyline, or polygon feature classes. You can take advantage of the standard ArcGIS Symbology and Labeling to represent the schematic elements in a schematic diagram (SchematicDiagram).
- In-memory objects—Schematic diagrams can now be edited. When a schematic diagram is edited, the diagram, as well as its features are loaded as in-memory objects. When the edits are saved, the changes are automatically reported on the corresponding schematic objects in the schematic database.
- Schematic events—Schematic events are now managed from the ISchematicDatasetEvents interface.
- Schematic controls—All the schematic related commands have been moved from the Controls package to the new com.esri.arcgis.schematiccontrols package. Also, a set of new commands that manage schematic diagram editing and managing layer properties have been added. Some of them are as follows:
- ControlsSchematicImportLayerPropertiesCommand
- ControlsSchematicPropagateLayerPropertiesCommand
- ControlsSchematicRestoreDefaultLayerPropertiesCommand
- ControlsSchematicSaveEditsCommand
- ControlsSchematicStartEditCommand
- ControlsSchematicStopEditCommand
systemUI
The IToolPalette interface has been added to the com.esri.arcgis.systemUI package to define the attributes of a custom tool palette.
Deprecated or removed
The following sections describe items that have been deprecated or removed.
Eclipse documentation plug-in
The Eclipse documentation plug-in, deprecated at 9.3.1, has been removed at this release. It is still possible to get the documentation within Eclipse through an alternative workflow. For more information, see How to install ArcGIS plug-ins.
arcgisant
Ant is a powerful build tool for Java projects. In previous releases of ArcGIS, the use of a custom tool, arcgisant, was supported when working with the Java SDK samples. At ArcGIS 10, this approach has been deprecated. Beyond ArcGIS 10, ESRI will not ship the arcgisant product or the associated build files for samples. Samples will continue to be supported through the Eclipse IDE and executable Java Archive files (JARs).
RegTool
In the previous release, RegTool was an alternative approach to Java extension registration that provided a Java API, and command line tool that could be used to explicitly register and unregister Java extensions on a machine. This API and commend line tool has been removed from ArcGIS 10. Copy extension JARs to the well-know run time locations as the only workflow. See the following:
- To deploy extension JARs for ArcGIS Desktop, use <AGSDESKTOPJAVA>/java/lib/ext as the well-known location.
- To deploy ArcGIS Engine runtime, use <AGSENGINEJAVA>/java/lib/ext as the well-known location.
- To deploy ArcGIS Server, use <AGSSERVERJAVA>/java/lib/ext as the well-known location (<AGSDESKTOPJAVA>, <AGSENGINEJAVA>, and <AGSSERVERJAVA> are new environment variables introduced at ArcGIS 10 to point to the install location of each run time, respectively).
arcweb package
ESRI no longer offers the ArcWeb Services product line, and the ArcWeb package and its classes, interfaces, and methods have been removed. Any application that references the ArcWeb package cannot be migrated to 10 until and unless the references are removed. The alternative for ArcWeb services is ArcGIS Online Services.
Survey Analyst extension
The Survey Analyst extension, deprecated at 9.3.1, has been removed at this release and the subsequent SurveyDataEx, SurveyExt, and SurveyPkgs packages have been removed. The functionality of the Survey Analyst extension is now available through the Parcel Editor and Parcel Fabric features.
Development licensing | Deployment licensing |
---|---|
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |
Engine Developer Kit | Engine |