In this topic
- About the button
- About the abstract class Button
- Understanding the config.xml file
- Creating different types of customizations
About the button
A button in the add-in framework is the simplest form of functionality that can be used to execute some business logic when the button is clicked. A button assumes that a series of actions will be executed.
The following guides you through the process of creating a button using the Eclipse IDE. Before beginning this workflow, make sure that you have created an ArcMap add-in project using Eclipse. For more information, see How to create an add-in project in Eclipse. Since there is no difference between creating a button for any ArcGIS for Desktop application, this workflow shows you how to create a button for ArcMap. This topic examines the process of creating a simple "Hello, world!" button. The abstract class button is then examined in greater detail to explore the additional methods that can be overridden to add additional functionality to your button. The final piece examines the Extensible Markup Language (XML) that is generated by the workflow presented in this topic.
The workflow for creating a button in Eclipse consists of the following (done in the order as shown):
- Creating a button—Examines the process of creating a button in Eclipse.
- Setting properties—Examines all of the properties that can be set for the button with detailed descriptions for each property.
- Creating a Java class and defining your business logic—Examines creating a Java class that is used to define all the business logic for the add-in button.
Creating a button
The following shows how to create a button for an ArcMap add-in project. Ensure the Add-In view is enabled on the Add-In Editor for the config.xml file and that you have completed the required Add-In Overview properties. See the following screen shot:

- Under all Add-Ins on the Add-In Editor, click the Add button. The Create New Add-In dialog box appears. See the following screen shot:
-
Select the Button option on the preceding screen shot (there are eight different options), then click OK.
A new section of the add-in editor appears with various properties for you to set for your new button. By default, the id*, class*, caption*, and category* are completed with default values to help expedite the development process. Also, observe the warning symbol under Button Details and next to the class* property. This indicates that the Java class for your new button has not been created. In a later step in this workflow, you will learn how to create your Java class and where you write your business logic. For now, the following screen shot shows you the new Button Details section that is added to the add-in editor with default values:
Setting properties
A button has a number of properties for you to set. The following is a list of all of the properties with an explanation for each:
- id*—Represents the unique name that is used to identify your button. It is possible for you to create more than one button for a given project and this id property is used to distinguish between the different buttons. Notice that the preceding screen shot shows a default value for this property. Ideally, you should replace this id with a more meaningful value. Use the Java package naming convention when constructing your id property value. For example, com.esri.arcgis.arcmap.addin.arcmapbutton could be used to represent the button being created for this topic (required).
- class*—Used to identify the Java class for your button. The Java class is where you write your business logic for the button. This class is important because it is executed when the button is clicked in a desktop application. To create a class, you can click the class property. Notice that the class property has a link (blue and underlined) to indicate this (required). Use the Java package naming convention when constructing your class. For example, com.esri.arcgis.addin.ArcMapButton could be used to represent the add-in button class being created (required).
-
caption*—Used to name the button and is used in two different locations after a project is deployed (required).
The first location is the Add-In Manager, where the caption is used as metadata to help an end user identify the different types of customizations available. The following screen shot shows a value of ArcMap Button for the caption property and is exposed in the Add-In Manager as follows (notice the type of add-in is identified in parentheses):
The second place that the caption property is used is when an end user is adding the button to the user interface (UI) of a desktop application. The workflow for doing so is discussed in How to deploy your add-in. For reference, the Customize dialog box Commands tab is used and will use the previous caption under the Commands text box as shown in the following screen shot: -
category*—Used to give a name that can identify your add-ins as a group. A given category can have more than one customization by supplying the same name for the Category property in each add-in that is defined. For example, you could have an ArcMap Add-Ins category that can contain all your customizations for ArcMap, for example, button, tool, toolbar, and so on. To achieve this, give the same category value for each type of add-in defined in your project (required).
Identical to the caption property, the category property is visible in two locations, first is in the Add-In Manager (notice that the category explicitly identifies this property). See the following screen shot:
The second place that the category is used is when a user is locating the customization on the Customize dialog box in a desktop application. The Customize dialog box Commands tab lists a number of categories (for example, Animation, ArcScan, and so on), and the category property value you set is used in this list to identify your add-ins. See the following screen shot: -
image—16 by 16 pixel image that can be used to represent your button. The image format should be one of the commonly used formats (that is, .bmp, .jpg, and so on). This image is used on the Customize dialog box Commands tab to symbolize your button and also used by default, when your button is added to the UI of a desktop application. Place the image within an image folder in your Eclipse add-in project and the Browse button allows you to add a reference to this image (optional).
-
tooltip—Brief description of the button that can be used to intuitively help your end user use your button. The ToolTip appears when the mouse pointer pauses over the button in a desktop application. A message dialog box then appears with the message supplied for this property (optional).
-
message—More detailed description of the button that describes what the button does. Identical to the ToolTip, the message appears when the mouse pointer pauses over the button in a desktop application in conjunction with the ToolTip. This message appears with the value supplied to this property in the status bar of a desktop application, which is located in the lower left corner (optional).
The final section of the button is Help Content. This section is for the help content you can supply a user with about the button. These properties allow you to supply information that will be used when a user invokes context-sensitive Help. These are pop-up topics that remain on-screen until a user clicks somewhere else.
The following properties comprise this context-sensitive Help section:
-
Heading—Indicates what the text is about (optional).
-
Content—Where you place the content for the button (optional).
Creating a Java class and defining your business logic
At this stage, you have finished adding values for all of the properties needed to define the add-in button. The final step in this workflow is to create a Java class that contains your business logic. Do the following steps to create the Java class:
- Click the class* property found under the Button Details section of the add-in editor. A new Java class dialog box appears with a few pre-populated form boxes. The one of interest is the superclass property. This property is unavailable automatically because a button class that you create must inherit the abstract class Button from the com.esri.arcgis.addins.desktop package. This abstract class is used to hide the implementation details for making the button work with the desktop applications.
- Add a package for your new class, add a name for the Java class file, then click Finish.
A class, in this instance, called ArcMapButton, is similar to the one generated by Eclipse. See the following code example:
[Java]
public class ArcMapButton extends Button{
@Override public void onClick()throws IOException, AutomationException{}
}
The generated class file has two significant pieces. The first is the use of the extends keyword to inherit from the abstract class Button. The second is the auto-generated onClick() method that is stubbed out in the previous code example. The onClick() method is important because this is where you write your business logic code for the button. If your button is clicked in a desktop application, this method is invoked to execute your macro or domain specific functionality.
- Add your business logic to the auto-generated onClick() method. See the following code example:
public class ArcMapButton extends Button{
@Override public void onClick()throws IOException, AutomationException{
JOptionPane.showMessageDialog(null, "Hello, World!");
}
}
The previous code example prints a message dialog box with the "Hello, World!" message when the button is clicked in a desktop application. This method provides the location that your macro or domain specific functionality is defined. For more information on how to deploy the button, see the previously mentioned topic, How to deploy your add-in.
About the abstract class Button
The abstract class Button provides you with additional methods that can be overridden, but are not required like the onClick() method described previously. Three such methods exist and are described here in detail.
init() method
The first, and most significant, is the init() method. When working with one of the ArcGIS for Desktop applications, it is possible that you need to access various items within the given application. For example, in ArcMap you might want to access the maps (that is, data frames) or layers contained within a map. Your Java application cannot instantiate an instance of the ArcMap application; instead, you are passed a reference to an object that gives you the application that the button is contained within.
The init() method serves this purpose as well as defining any logic that is necessary to initialize your button (for example, instantiate objects that are needed in your onClick() method). How then are you able to get an object that points to the desktop application your button is hosted in? First, you need to write some code to hold onto the IApplication object that is passed into the init() method when it is invoked. This can be achieved with the following initialization code example:
[Java]
private IApplication app;
@Override public void init(IApplication app){
this.app = app;
}
Once you have a reference to the IApplication object, you can use it to get a reference to the specific desktop application the button is being used in with the following code example:
- Obtain an object reference to ArcMap.
IMxDocument mxDocument = (IMxDocument)app.getDocument();
- Obtain an object reference to ArcCatalog. See the following code example:
IGxDocument gxDocument = (IGxDocument)app.getDocument();
- Obtain an object reference to ArcGlobe. See the following code example:
IGMxDocument gMxDocument = (IGMxDocument)app.getDocument();
- Get an object reference to ArcScene. See the following code example:
ISxDocument sxDocument = (ISxDocument)app.getDocument();
isChecked() method
In some special circumstances, it might be important for you to determine if the button has been executed or not. The isChecked() method reports this state of a button, where by default, it is set to false. When this method returns true, the button appears as though it is pressed in the desktop application as the following screen shot shows:
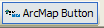
A system event is periodically called to set the statement of buttons on toolbars, thus determining if a button is selected or not selected. If a condition is used to determine this status, the logic should not be complicated or lengthy for this reason.
isEnabled() method
The following method allows you to add some logic to specify the state the desktop application should be in for the button to be enabled and clicked. For example, you might have a button that requires a data layer to be loaded in ArcMap before execution is possible. The isEnabled() method allows you to write logic to test if a layer is present or not. If a layer has been added to ArcMap, the button is enabled; otherwise, it remains disabled until that action is performed. Similar to the isChecked() method, a system event is called to check if a button is enabled or not, and thus, the logic should not be complicated or lengthy for this reason.
Instead of writing code to stub out all of these methods, Eclipse provides you with a mechanism for including any one or all of the methods into your source code and it also helps you avoid typos. To insert any one or all of the methods into your Java class source code, right-click anywhere in the Eclipse's source code editor and select Source, then Override/Implement Methods. The following dialog box gives you the ability to add any one or all of the methods defined:
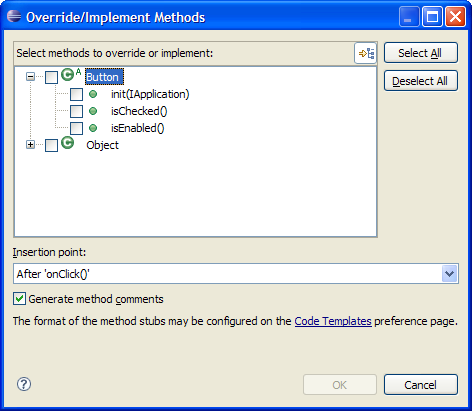
Understanding the config.xml file
When setting all of the properties, the raw XML for the config.xml file was being generated behind the scenes. To understand more about how this config.xml file is defined, see Understanding the config.xml.
Creating different types of customizations
This topic showed how to create a button. However, there are additional types of add-ins that can be created and defined. See the following topics that discuss each type of add-in.
- How to create an add-in tool
- How to create an add-in combo box
- How to create an add-in toolbar
- How to create an add-in tool palette
- How to create an add-in menu
- How to create an add-in dockable window
- How to create an add-in application extension
See Also:
Understanding the config.xmlDevelopment licensing | Deployment licensing |
---|---|
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |