In this topic
- About the tool
- About the abstract class Tool
- deactivate() method
- init() method
- isChecked() method
- isEnabled() method
- keyPressed() method
- keyReleased() method
- mouseMoved() method
- mousePressed() method
- mouseReleased() method
- onContextMenu() method
- onDoubleClick() method
- refresh() method
- Understanding the config.xml
- Creating different types of customizations
About the tool
A tool is to a button; however, a tool requires user interaction with the desktop application's display first, then based on that interaction, executes some business logic. ArcMap's Zoom In tool is a good example that requires an end user to click or drag a rectangle over a map before the display is redrawn to show the map contents in greater detail for the specified area.
This topic guides you through the process of creating a tool using the Eclipse IDE. Before beginning this workflow, make sure that you have created an ArcMap add-in project using Eclipse. For more information, see How to create an add-in project in Eclipse.
Since there is no difference between creating a tool for any ArcGIS for Desktop application, this workflow shows you how to create a tool for ArcMap. The workflow for creating a tool in Eclipse consists of the following (done in the order as shown):
- Creating a tool—Examines the process of creating a tool in Eclipse.
- Setting properties—Examines the properties that can be set for the tool with descriptions for each property.
- Creating a Java class and defining your business logic—Examines the creation of a Java class that is used to define the business logic for the tool.
Creating a tool
The following shows how to create a tool for an existing Eclipse add-in project. Ensure that you have the Add-In view enabled on the Add-In Editor for the config.xml file and that you have completed the required Add-In Overview properties. See the following screen shot:

-
Under All Add-ins on the Add-In Editor, click the Add button. The Create New Add-In dialog box appears. See the following screen shot:
-
Select the Tool option on the preceding screen shot (there are eight different options), then click OK.
A new section of the Add-In Editor appears with various properties for you to set for your new tool. By default, the id*, class*, caption*, and category*are completed with default values to help expedite the development process. Also, observe the warning symbol under the Tool Details and next to the class* property. This indicates that the Java class for the new tool has not been created. In a later step in this workflow, you will learn how to create your Java class and where you write your business logic. For now, the following screen shot shows you the new Tool Details section that is added to the Add-In Editor with default values:
Setting properties
A tool has a number of properties for you to set. The following is a list of all of the properties with an explanation for each:
- id*—Represents the unique name that is used to identify your tool. You can create more than one tool for a given project and this id is used to distinguish between the different tools. Notice that the image shows a default value for this property. Ideally, you should replace this id with a more meaningful name. Use the Java package naming convention when constructing your id property value. For example, com.esri.arcgis.arcmap.addin.arcmaptool could be used to represent the tool being created in this topic (required).
- class*—Used to identify the Java class for your tool. The Java class is where you will write your business logic. This class is important because it is called when the tool is invoked in a desktop application. To create a class, you can click the class property. Notice that the class property has a link (blue and underlined) to indicate this. Use the Java package naming convention when constructing your class. For example, com.esri.arcgis.addin.ArcMapTool could be used to represent the tool class being created (required).
-
caption*—Used to name the tool and is used in two different locations after a project is deployed (required).
The first location is in the Add-In Manager, where the caption is used as metadata to help a user identify the different types of customizations available. The following screen shot shows a value of ArcMap Tool for the caption property and is exposed in the Add-In Manager as follows (notice the type of add-in is identified in parentheses):
The second place that the caption is used is when an end user is adding the tool to the user interface (UI) of a desktop application. For this workflow, see How to deploy your add-in. For reference, the Commands tab on the Customize dialog box is used (uses the caption defined under the Commands text area). See the following screen shot:
-
category*—Used to give a name that can identify your add-ins as a group. A given category can have more than one by supplying the same name for the category property in each defined add-in. For example, you could have a category that can contain all your add-ins for ArcMap, such as a button, tool, toolbar, and so on. To achieve this, give the same category value for each type of add-in defined in your project (required).
Identical to the caption property, the category property is visible in two locations; the first is in the Add-In Manager (notice that the category explicitly identifies this property). See the following screen shot:
The second place the category is used is when a user is locating the add-in project on the Customize dialog box in a desktop application. The Customize dialog box Commands tab lists a number of categories (for example, Animation, ArcScan, and so on) and the category property value you set, is used in this list to identify your add-in type or types. See the following screen shot:
-
cursor —16 by 16 pixel image that can be used to represent your cursor. The cursor image is used when a user clicks the tool in a desktop application. The cursor image must have a .cur extension. The Browse button allows you to add a reference to the cursor image (optional).
-
image—16 by 16 pixel image that can be used to represent your tool. This image is used on the Customize dialog box Commands tab to symbolize your tool and also used by default when your tool is added to the UI of a desktop application. The image can be placed in your project and the Browse button allows you to add a reference to this image (optional).
-
tooltip—Brief description of your tool that can be used to intuitively help the user apply the tool or remind them of the tool's purpose. The ToolTip appears when the mouse pointer pauses over the tool in a desktop application. A message dialog box shows with the supplied message for this property (optional).
-
message—Detailed description of the tool that describes what the tool does. Identical to the ToolTip, the message appears when the mouse pointer pauses over the tool in a desktop application in conjunction with the ToolTip. This message appears with the value supplied with this property on the status bar of a desktop application, which is located in the lower left corner (optional).
The final section of the Tool Details is Help Content. This section is for the help content you can supply a user with about the tool. These properties allow you to supply information that will be used when a user invokes context-sensitive Help. These are pop-up topics that remain on-screen until a user clicks somewhere else.
The following properties comprise this context-sensitive Help section:
-
Heading—Header to indicate what the text is about (optional).
-
Content—Where you place the content for the tool (optional).
Creating a Java class and defining your business logic
At this stage, you have finished adding values for all of the necessary properties to define the tool. The final step is to create a Java class that contains your business logic. Do the following steps to create the Java class:
- Click the class* property found under Button Details. A new Java class dialog box appears with a few pre-populated form boxes. The one of interest is the superclass property. This property is automatically unavailable because a tool class that you create must inherit the abstract class Tool from the com.esri.arcgis.addins.desktop package. This abstract class is what is used to hide the implementation details for making your tool work with the desktop applications.
- Add a package for your new class, add a name for the Java class file, then click Finish.
A class, in this instance, called ArcMapTool, is similar to the one generated next. See the following code example:
[Java]
public class ArcMapTool extends Tool{
@Override public void activate()throws IOException, AutomationException{}
@Override public void mousePressed(MouseEvent arg0){}
}
The generated class file has two significant pieces. The first is the use of the extends keyword to inherit from the abstract class Tool (a required piece that contains the necessary plumbing code to get your tool to work with desktop applications). The second is the auto-generated activate() method that is stubbed out in the source code. The activate() method is important because this is where you write logic to define the action performed by your tool when it is activated in a desktop application (called once the user clicks the tool).
Typically, you will not just work with the activate() method alone, but in conjunction with another method, such as mousePressed(), to build your tools. The mousePressed() method is an action that you can write business logic for and will be called when the mouse is clicked by a user in the desktop application display area. This differs from a button, because a tool requires end user action before the business logic is executed (a subtle, but important difference). Depending on what you are attempting to achieve with your tool, your business logic will be placed in the method accordingly.
The following code example prints a message dialog box with the "Hello, World!" message when the tool is activated, and a user clicks the data or layout views in ArcMap. For instructions on how to deploy the tool, see the previously referenced topic, How to deploy your add-in.
[Java]
public class ArcMapTool extends Tool{
@Override public void activate()throws IOException, AutomationException{}
@Override public void mousePressed(MouseEvent arg0){
JOptionPane.showMessageDialog(null, "Hello, World!");
}
}
About the abstract class Tool
The abstract class Tool provides you with a set of additional methods that can be overridden, but are not required like the activate() method, but are necessary to make your tool useful. The following sections describe these methods.
deactivate() method
The deactivate() method is called by the ArcGIS for Desktop framework when the tool is active, and the user selects another tool instead of using the active selected tool to deactivate it. If appropriate, this method can be overridden with a Boolean test to determine if this tool should be deactivated.
init() method
When working with one of the ArcGIS for Desktop applications, it is possible that you might need to access various items within the applications. For example, in ArcMap, you might want to access the map (that is, data frame) or layers contained within a map.
Your Java application cannot just instantiate an instance of the ArcMap application; instead, you are passed a reference to an object that gives you the application that the tool is contained within. The init() method serves this purpose, as well as defining any logic that is necessary to initialize your tool (for example, instantiate objects that are needed in your mousePressed() method).
To get an object that points to the desktop application your tool is hosted in, write code to hold onto the IApplication object that is passed into the init() method when it is invoked. This can be achieved with the following initialization code example:
[Java]
private IApplication app;
@Override public void init(IApplication app){
this.app = app;
}
Once you have a reference to the application object, you can use it to get a reference to the desktop application in which the tool is being used.
- Obtain an object reference to ArcMap. See the following code example:
IMxDocument mxDocument = (IMxDocument)app.getDocument();
- Obtain an object reference to ArcCatalog. See the following code example:
IGxDocument gxDocument = (IGxDocument)app.getDocument();
- Obtain an object reference to ArcGlobe. See the following code example:
IGMxDocument gMxDocument = (IGMxDocument)app.getDocument();
- Get an object reference to ArcScene. See the following code example:
ISxDocument sxDocument = (ISxDocument)app.getDocument();
isChecked() method
In some special circumstances it might be important for you to determine if your tool has been executed or not. The isChecked() method reports this state of a tool, where by default, it is set to false. When this method returns true, the tool appears as though it is pressed in the desktop application as the following screen shot shows:

A system event is periodically called to set the status of tools on toolbars, thus determining if a tool is checked or not. If a condition is used to determine this status, the logic should not be complicated or lengthy for this reason.
isEnabled() method
This method allows you to add some logic to specify in what state the desktop application should be in for the tool to be enabled and thus, allow someone to click it. For example, you might have a tool that requires a data layer to be loaded in ArcMap before execution is possible. The isEnabled() method allows you to write logic to test if a layer is present or not.
If a layer has been added to ArcMap, the tool is enabled; otherwise, it remains disabled until that action is performed. Similar to the isChecked() method, a system event is called to check if a tool is enabled or not.
keyPressed() method
The keyPressed() method is invoked when a keyboard key is pressed, while the tool is active. Business logic can be written to respond to any key or a specific key on the keyboard. The KeyEvent that is generated and passed into the keyPressed() method comes from the java.awt.event package.
keyReleased() method
The keyReleased() method is invoked when a keyboard key is released, while the tool is active. Business logic can be written to respond to any key or a specific key on the keyboard. The KeyEvent that is generated and passed into the keyReleased() method comes from the java.awt.event package.
mouseMoved() method
The mouseMoved() method is invoked when the mouse is moved, while the tool is active. Business logic can be written to respond to mouse movement on the display of desktop applications. The MouseEvent that is generated and passed into the mouseMoved() method comes from the java.awt.event package.
mousePressed() method
The mousePressed() method is invoked when the mouse button is pressed, while the tool is active. Business logic can be written to respond to a mouse click on the display of desktop applications. The MouseEvent that is generated and passed into the mousePressed() method, comes from the java.awt.event package.
mouseReleased() method
The mouseReleased() method is invoked when the mouse button is released, while the tool is active. Business logic can be written to respond to a mouse click's release on the display of desktop applications. The MouseEvent that is generated and passed into the mouseReleased() method comes from the java.awt.event package.
onContextMenu() method
The onContextMenu() method is invoked when a context menu event occurs at a given x,y location. A context menu is initiated when a right-click is done and a pop-up menu appears. Business logic can be written to respond to a context menu and the x,y coordinates of the clicked location are passed to this method. It is possible to expose different business logic depending on where the user invoked the context menu.
onDoubleClick() method
The onDoubleClick() method is invoked when a double-click is performed by the user and the tool is active. Business logic can be written to respond to this double-click action.
refresh() method
The refresh() method is invoked when the screen display on the desktop application is refreshed. Business logic can be written while the refresh() method is being invoked.
Instead of writing code to stub out all of these methods, Eclipse provides a mechanism to include any or all of the preceding methods into your source code, and it also helps to avoid typographical errors.
To insert any or all of the methods into your Java class source code, right-click on Eclipse's source code editor and select Source, then Override/Implement methods. The following Override/Implement Methods dialog box appears, which allows you to add any or all of the defined methods:
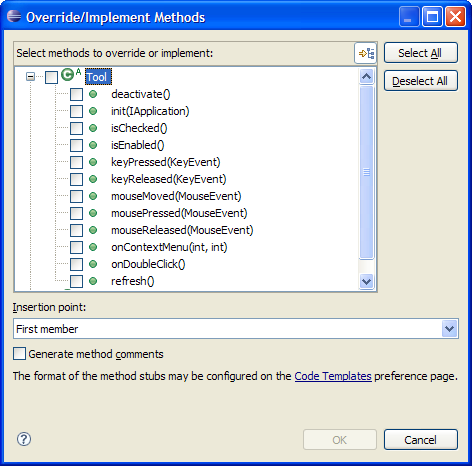
Understanding the XML
When setting all of the properties, the raw XML for the config.xml file was being generated behind the scenes. To understand more about how this config.xml file is defined, see Understanding the config.xml.
Creating different types of customizations
This topic showed how to create a tool; however, there are additional types of add-ins that can be created and defined. See the following topics that discuss each type of add-in.
- How to create an add-in button
- How to create an add-in combo box
- How to create an add-in toolbar
- How to create an add-in tool palette
- How to create an add-in menu
- How to create an add-in dockable window
- How to create an add-in application extension
See Also:
Understanding the config.xmlDevelopment licensing | Deployment licensing |
---|---|
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |