- ArcGIS for Desktop Basic
- ArcGIS for Desktop Standard
- ArcGIS for Desktop Advanced
Additional library information: Contents, Object Model Diagram
The Catalog library contains objects and interfaces that support data catalogs. The catalog is a representation of persistent data. The data can be local and remote. Using the objects in the Catalog library, you can browse data holdings and, if required, obtain connections to the data. Many of the objects defined in the Catalog library are referred to as GxObjects, as they inherit from the GxObject abstract class. All GxObjects implement the IGxObject interface. Objects that implement this interface can be manipulated in a catalog. GxFilter objects allow you to browse for certain types of data and are also defined in this library.
Developers commonly extend this library to add catalog support for a data type not already supported by the ArcGIS system.
See the following sections for more information about this namespace:
GxCatalog
The GxCatalog object represents the actual data tree, as shown in the tree view (the top-level object in the tree view). From the GxCatalog object, you can navigate to any of its descendants to access and manipulate them. The GxCatalog object is a type of GxObject and a type of GxObjectContainer because it is an item in the tree view and it contains additional GxObjects. The GxCatalog object is shown in the following diagram:
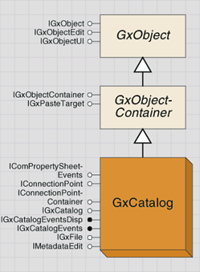
The GxCatalog object is also an event source that monitors the addition, deletion, and change of GxObjects in the catalog through the IGxCatalogEvents interface.
The GxCatalog object implements the IGxCatalog interface. It lets you connect and disconnect folder objects. It also maintains the file filter associated with ArcCatalog.
GetObjectFromFullName returns a variant because it is possible to get more than one GxObject back from this method. For example, if you use this method on a computer-aided design (CAD) file, it returns two objects: one for the CAD file and one for the CAD dataset.
The SelectedObject property returns the first selected object in the tabbed view of ArcCatalog.
The following code uses the Location property to change the selected folder in the tree view to d:\tools:
[VB.NET] Dim gxApp As IGxApplication
Dim gxCat As IGxCatalog
gxApp=TryCast(Application, IGxApplication)
gxCat=gxApp.Catalog
gxCat.Location="d:\tools"
[C#] IGxApplication gxApp=null;
IGxCatalog gxCat=null;
gxApp=Application as IGxApplication;
gxCat=gxApp.Catalog;
gxCat.Location="d:\\tools";
GxObject
GxObject is the most important abstract class in the Catalog library. Every item in the catalog is a type of GxObject. There are several dozen types of GxObjects. Different types of GxObjects are used for different types of data. For example, the GxLayer object represents layer files, whereas the GxMap object encapsulates map documents.
GxObject is extensible, meaning you can implement your own GxObjects so that they display in ArcCatalog. To create a custom GxObject, first implement IGxObjectFactory, which returns GxObjects. Then, implement the IGxObject and IGxObjectUI interfaces to show the GxObject in ArcCatalog using your custom icons. You can implement various methods under the IGxObject interface to provide specific operations on this object. For example, implementing the Category property displays the object's category in the Type column of the Details view in ArcCatalog. The IGxObjectUI interface allows you to specify a bitmap for your custom GxObject that is viewable in the tree view. It includes methods to use small or large icons according to the type of view being displayed - details, list, or icons.
To be a GxObject, an object only needs to support IGxObject, although it usually implements a number of other interfaces as well. It must support IGxObject since ArcCatalog uses this interface to set up and tear down the object, as well as to retrieve certain critical information from it during its lifetime. The IGxObject interface provides read-only access to the descriptors of the object, such as name, parent, and category.
Using the IGxObject interface, ArcCatalog calls Attach to initialize the object, passing in references to its parent and the GxCatalog object. The object holds on to these references, releasing them when ArcCatalog calls the Detach method. This behavior guarantees that no circular dependencies develop between the object and its parent or the GxCatalog coclass.
ArcCatalog relies on the following separate properties to retrieve information about the textual name of the object:
- Name - Indicates the short name of the object including its extension if any.
- BaseName - Returns the name without the filename extension, if it has one.
- FullName - Returns a string identifying the fully qualified pathname of the object starting at the root level. This is not necessarily a path to a file on disk, since the object could exist within a database hierarchy. It is a fully qualified path in the context of ArcCatalog. It is comprised of the names of all its GxObject parents, each separated with the backslash ("\") character.
The easiest way for an object to assemble and return this path is to call the ConstructFullName method on the GxCatalog object, passing in itself as a parameter.
InternalObjectName is used for data transfer operations. If you want your object to participate in drag-and-drop or copy-and-paste operations, you need to return something for this property. This property represents the data object managed by GxObject. For example, database objects, such as GxDatabase and GxDataset, wrap underlying geodatabase entities, such as workspaces and datasets. It is these underlying objects that InternalObjectName references, not the GxObject itself. Moreover, this property indirectly references these underlying objects via a name object (also called a moniker).
ArcCatalog calls Refresh on an object to ensure the state is up to date. In most cases, this happens as a direct result of forcing a refresh as part of the GxCatalog tree. The object has the responsibility to release and recreate its internal state, then propagate the Refresh call on to any children it has. IsValid is called periodically by ArcCatalog to verify that the object is in a legitimate state. Typically, it does so prior to performing critical operations involving the object, such as data transfer.
The following code demonstrates how to loop through the selected objects in the tabbed view and print their categories:
[VB.NET] Dim gxApp As IGxApplication=TryCast(Application, IGxApplication)
Dim gxSel As IGxSelection
Dim enumGxObj As IEnumGxObject
Dim gxObj As IGxObject
gxSel=gxApp.Selection
enumGxObj=gxSel.SelectedObjects
gxObj=enumGxObj.Next
Do While Not gxObj Is Nothing
Debug.Print(gxObj.Category)
gxObj=enumGxObj.Next
Loop
[C#] IGxApplication gxApp=Application as IGxApplication;
IGxSelection gxSel=null;
IEnumGxObject enumGxObj=null;
IGxObject gxObj=null;
gxSel=gxApp.Selection;
enumGxObj=gxSel.SelectedObjects;
gxObj=enumGxObj.Next();
while (gxObj != null)
{
Debug.Print(gxObj.Category);
gxObj=enumGxObj.Next();
}
During an object's lifetime, ArcCatalog uses the SmallImage, SmallSelectedImage, LargeImage, and LargeSelectedImage properties of the optional IGxObjectUI interface to determine the images to use when displaying the object in the tree and contents views. The object should return HBITMAPs for these properties. Since these properties are requested frequently, load the images once and cache them for later retrieval, rather than loading them each time they are requested. If you don't implement IGxObjectUI, ArcCatalog can still display and work with the object, but it will use a generic icon in the various views.
ContextMenu and NewMenu return globally unique identifiers (GUIDs) that indicate the menus that display when you attempt to manipulate the object through the ArcCatalog user interface (UI).
An object implements the IGxObjectEdit interface if its properties can be edited within the context of ArcCatalog. This interface consists of the following methods:
- Rename assigns a new short name to the object (if true is returned for the CanRename method).
- If true is returned for the CanDelete method, Delete physically deletes the object and its associated underlying data; ArcCatalog handles deleting the GxObject, but it is the object's responsibility to delete all underlying and associated data that the object represents or wraps.
- CanCopy indicates if the object is a valid source for a copy operation; a return value of true enables the Copy command or menu item in ArcCatalog. However, to fully enable an object to participate in data transfer operations, you also need to implement the IGxObject.InternalObjectName property as described in the earlier discussion on data transfer.
- The EditProperties method opens a dialog box appropriate to the object that allows you to manipulate its internal properties and state. It's up to you as to what can and cannot be manipulated through this dialog box, but a good rule of thumb is the object's properties, not the data contained by the object, should appear here. For example, if the object is a table, this dialog box might show a list of the present columns and their data types, and permit editing of this information. However, the rows of data in the table would not be presented in this dialog box.
The IGxPasteTarget interface is implemented by those GxObjects that can have other objects pasted into them. For example, GxDataset implements IGxPasteTarget because it's possible to paste feature classes into an object of this type through the ArcCatalog UI. The interface provides methods for testing whether or not a set of Name objects can be pasted and methods to perform the paste. Use CanPaste to determine if at least one object in the current set can be pasted before executing the Paste method.
IGxObjectInternalName is an optional interface for the various types of GxObjects. This interface provides access to the internal name of the object that implements it through the InternalObjectName property.
GxObjectContainer
GxObjectContainer is an abstract class. This container class is for GxObjects that contain other GxObjects. For example, the GxDatabase object can contain GxDataset objects, so the GxDatabase object is also a type of GxObjectContainer object. If an object can contain other objects as children, it must implement the IGxObjectContainer interface. This interface exposes methods and properties that access and manipulate the children of the object.
The HasChildren property indicates if the object presently has any children. Children returns an enumeration of the current set of children. AreChildrenViewable indicates if the children are shown in the tree view in ArcCatalog; typically this makes sense, but in certain cases, you may not want them viewable.
The AddChild and DeleteChild methods do not have to be implemented; they are only used when a container is running in ArcCatalog and you want to either create new items in that container or remove items from it. They are not required, since performing a Refresh on the container (or one of its ancestors) will refresh its children as well.
The following code demonstrates how to loop through the children of a GxObjectContainer object:
[VB.NET] Dim gxApp As IGxApplication=TryCast(Application, IGxApplication)
Dim gxSel As IGxSelection
Dim gxObj As IGxObject
gxSel=gxApp.Selection
gxObj=gxSel.Location
If TypeOf gxObj Is IGxObjectContainer Then
Dim gxObjCont As IGxObjectContainer
Dim gxEnum As IEnumGxObject
Dim gxObject As IGxObject
gxObjCont=TryCast(gxObj, IGxObjectContainer)
gxEnum=gxObjCont.Children
gxObject=gxEnum.Next
Do While Not gxObject Is Nothing
Debug.Print(gxObject.Category)
gxObject=gxEnum.Next
Loop
End If
[C#] IGxApplication gxApp=Application as IGxApplication;
IGxSelection gxSel=null;
IGxObject gxObj=null;
gxSel=gxApp.Selection;
gxObj=gxSel.Location;
if (gxObj is IGxObjectContainer)
{
IGxObjectContainer gxObjCont=null;
IEnumGxObject gxEnum=null;
IGxObject gxObject=null;
gxObjCont=gxObj as IGxObjectContainer;
gxEnum=gxObjCont.Children;
gxObject=gxEnum.Next();
while (gxObject != null)
{
Debug.Print(gxObject.Category);
gxObject=gxEnum.Next();
}
}
GxObjectContainer objects that are based on remote connections implement the IGxRemoteContainer interface. The GxRemoteDatabaseFolder object is an example of a container object for remote database connections. The IGxRemoteContainer interface has no properties or methods, but it does identify the implementing object as a remote container object.
GxObjectFilter
There are more than thirty types of GxObjectFilter objects you can use in the code. You can also create your own code depending on how you want your users to apply the GxDialog object. Through the use of objects of this type, you can determine the types of objects your users can select for open and save operations when browsing data.
The GxObjectFilter object is shown in the following diagram:
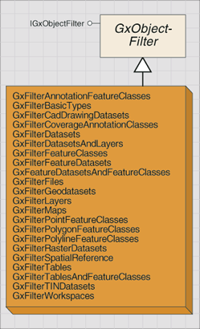
All GxObjectFilter objects implement the IGxFileFilter interface. This interface enables the specification of certain file types during open and save operations when using a GxDialog browser object. You typically only access this interface when implementing it as part of a custom GxObjectFilter.
The following code demonstrates how to create a custom filter in a class:
[VB.NET] Dim basicFilter As IGxObjectFilter
Private Sub New()
basicFilter=New GxFilterBasicTypes
End Sub
Private Function IGxObjectFilter_CanChooseObject(ByVal gxObject As IGxObject, ByVal result As _
esriDoubleClickResult) As Boolean
Dim canChoose As Boolean
canChoose=False
If TypeOf gxObject Is IGxFile Then
Dim ext As String
ext=GetExtension(gxObject.Name)
If LCase(ext)=".shd" Or LCase(ext)=".pal" Then canChoose=True
End If
IGxObjectFilter_CanChooseObject=canChoose
End Function
Private Function IGxObjectFilter_CanSaveObject(ByVal Location As _
IGxObject, ByVal newObjectName As String, _
ByVal objectAlreadyExists As Boolean) As Boolean
End Function
Private ReadOnly Property IGxObjectFilter_Name() As String
Get
IGxObjectFilter_Name="Custom filter"
End Get
End Property
Private ReadOnly Property IGxObjectFilter_Description() As String
Get
IGxObjectFilter_Description="Browses for .shd and .pal files."
End Get
End Property
Private Function IGxObjectFilter_CanDisplayObject(ByVal gxObject As IGxObject) As Boolean
Dim canDisplay As Boolean
canDisplay=False
If basicFilter.CanDisplayObject(gxObject) Then
canDisplay=True
ElseIf TypeOf gxObject Is IGxFile Then
Dim ext As String
ext=GetExtension(gxObject.Name)
If LCase(ext)=".shd" Or LCase(ext)=".pal" Then canDisplay=True
End If
IGxObjectFilter_CanDisplayObject=canDisplay
End Function
Private Function GetExtension(ByVal fileName As String) As String
Dim extPos As Long
extPos=InStrRev(fileName, ".")
If extPos > 0 Then
GetExtension=Mid(fileName, extPos)
Else
GetExtension=""
End If
End Function
[C#] private IGxObjectFilter basicFilter;
private void NewFilter()
{
basicFilter=new GxFilterBasicTypes();
}
private bool IGxObjectFilter_CanChooseObject(IGxObject gxObject,
esriDoubleClickResult result)
{
bool canChoose=false;
canChoose=false;
if (gxObject is IGxFile)
{
string ext=null;
ext=GetExtension(gxObject.Name);
if (ext.ToLower() == ".shd" | ext.ToLower() == ".pal")
{
canChoose=true;
}
}
return canChoose;
}
private bool IGxObjectFilter_CanSaveObject(IGxObject Location, string newObjectName,
bool objectAlreadyExists)
{
return false;
}
private string IGxObjectFilter_Name
{
get
{
return "Custom filter";
}
}
private string IGxObjectFilter_Description
{
get
{
return "Browses for .shd and .pal files.";
}
}
private bool IGxObjectFilter_CanDisplayObject(IGxObject gxObject)
{
bool canDisplay=false;
canDisplay=false;
if (basicFilter.CanDisplayObject(gxObject))
{
canDisplay=true;
}
else if (gxObject is IGxFile)
{
string ext=null;
ext=GetExtension(gxObject.Name);
if (ext.ToLower() == ".shd" | ext.ToLower() == ".pal")
{
canDisplay=true;
}
}
return canDisplay;
}
private string GetExtension(string fileName)
{
string tempGetExtension=null;
long extPos=0;
extPos=(fileName.LastIndexOf(".") + 1);
if (extPos > 0)
{
tempGetExtension=fileName.Substring(extPos - 1);
}
else
{
tempGetExtension="";
}
return tempGetExtension;
}
Metadata import and export
Starting with ArcGIS 10, importing and exporting metadata is done using the Metadata toolset. For a complete overview of the toolset, see An overview of the Metadata toolset.
The Import Metadata command opens the Import Metadata geoprocessing tool. The term "Importing metadata" is used in reference to importing metadata from a standard metadata Extensible Markup Language (XML) format to an ArcGIS item or to copying ArcGIS metadata from one item to another. Metadata can be imported from the International Organization for Standardization (ISO) 19139 XML metadata format, the Federal Geographic Data Committee (FGDC) XML metadata format, and the ESRI-ISO XML metadata format. ArcGIS metadata can also be metadata that's copied from one item to another. Importing metadata from other formats is not supported. The target item's thumbnail, geoprocessing history, and various ESRI properties of the item are not overwritten when metadata is imported with this tool. The item's metadata is synchronized after the information is imported.
The Export Metadata command opens the Export Metadata geoprocessing tool. The term "Exporting metadata" is used exclusively when referring to exporting ArcGIS metadata content to a standard metadata XML format for use outside of ArcGIS such as the ISO 19139 XML format. ArcGIS metadata is exported to the XML format associated with the current ArcGIS metadata style. To export ArcGIS metadata in Hypertext Markup Language (HTML) format, use the XSLT Transformation tool. If your current metadata style supports exporting metadata to the FGDC metadata XML format, the resulting file can be used with the U.S. Geological Survey (USGS) MP Metadata Translator geoprocessing tool to generate the text, HTML, and Standard Generalized Markup Language (SGML) files that are commonly associated with FGDC metadata.
For more information, as well as instructions for converting FGDC metadata in text or SGML format to XML format using Python, see Importing metadata and Exporting metadata.
In ArcGIS Desktop 9.3.1 and earlier releases, the Import Metadata command on the Metadata toolbar in ArcCatalog used objects that implemented the IMetadataImport interface to import metadata from a specific file format to the selected item. Similarly, the Export Metadata command used objects that implemented the IMetadataExport interface to export the selected item's data to a specific file format. The objects previously provided with ArcGIS Desktop that implemented these interfaces are no longer provided with ArcGIS. The capabilities they provided are now available using the metadata geoprocessing tools.
The IMetadataExport and IMetadataImport interfaces continue to be supported and are available programmatically. Custom objects that implement these interfaces will continue to work if you author code to programmatically create the objects and call the Export or Import method. Custom metadata exporter and importer objects do not have to be registered in the Metadata Exporters or Metadata Importers component categories - these categories are no longer used.