In this topic
- Creating the class library
- Creating the custom component
- Transferring .NET code
- Transferring XML
- Building, testing, debugging, and deploying the custom component
Creating the class library
You can use the Visual Studio Class Library template to create the class library that will contain your custom component. For more information, see Using project templates to extend ArcObjects. The following screen shot shows the Visual Studio New Project dialog box:
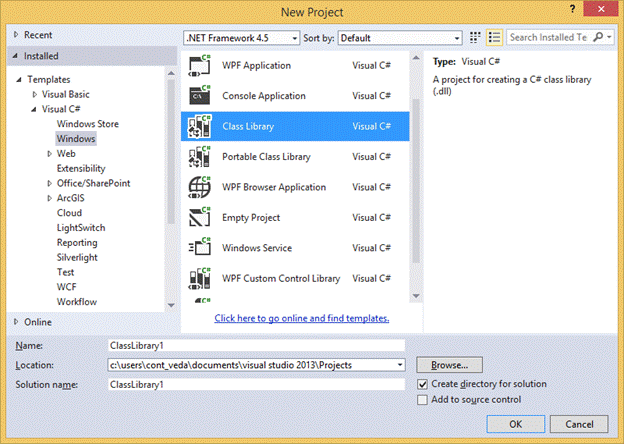
Your custom component must be registered for Component Object Model (COM) interoperability. If you create your class library using the Class Library project template, your library will be automatically registered for COM interoperability. To register your class library for COM interoperability when using something other than a project template, see How to register COM components.
Creating the custom component
Determine the type of add-in from which you're converting by examining the Config.esriaddinx file from your add-in project. The Extensible Markup Language (XML) code will be similar to the following (which indicates toolbar and button components are needed):
[XML] <ArcMap>
<Commands>
<Button
id="ArcMapAddin3_ArcGISAddin1"
class="ArcGISAddin1"
message="Add-in command generated by Visual Studio project wizard."
caption="My Button"
tip="Add-in command tooltip."
category="Add-In Controls"
image="Images\ArcGISAddin1.png"/>
</Commands>
<Toolbars>
<Toolbar id="ArcMapAddin3_My_Toolbar" caption="My Toolbar" showInitially="false">
<Items>
<Button refID="ArcMapAddin3_ArcGISAddin1" separator="false"/>
</Items>
</Toolbar>
</Toolbars>
</ArcMap>
The following XML code is another example. (In this case, a custom Editor extension is needed.)
[XML] <ArcMap>
<Editor>
<Extensions>
<Extension
id="ESRI_AddInEditorExtension_ValidateFeaturesExtension"
class="ValidateFeaturesExtension"/>
</Extensions>
</Editor>
</ArcMap>
Using an item template
The quickest way to create the custom component equivalent of an add-in is to use an item template. In Visual Studio, click Project, and click Add New Item. The Add New Item dialog box opens as shown in the following screen shot:
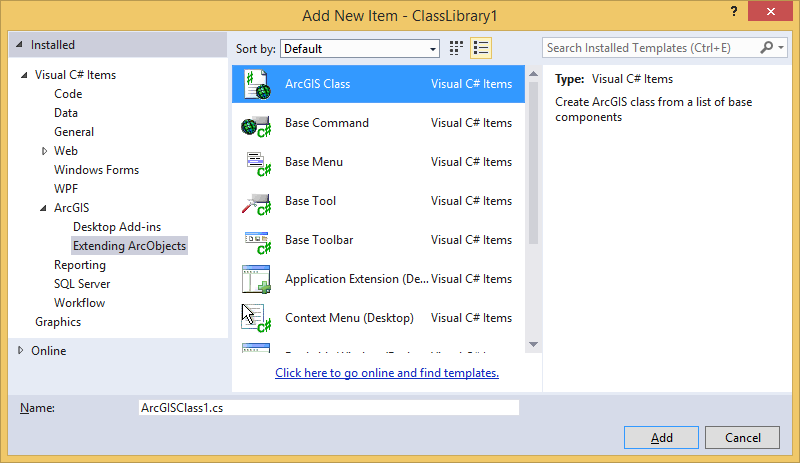
For more information, see Using item templates to extend ArcObjects.
The following table shows the ArcGIS item templates that are equivalent to certain add-in types:
Add-in type |
Item template |
ArcGIS Engine notes |
Button |
Base Command |
|
Tool |
Base Tool |
|
Menu |
Base Menu |
|
Context menu |
Context Menu (Desktop) or Context Menu (Engine) |
|
Application extension |
Just-In-Time Extension (Desktop) or Application Extension (Desktop) |
|
Dockable window |
Dockable Window (Desktop) |
There is no equivalent to a dockable window. |
MultiItem |
ArcGIS Class |
For a MultiItem, choose the ArcGIS Class item template, followed by the Application Framework option. The ArcGIS Add Class Wizard dialog box opens. See the following screen shot:
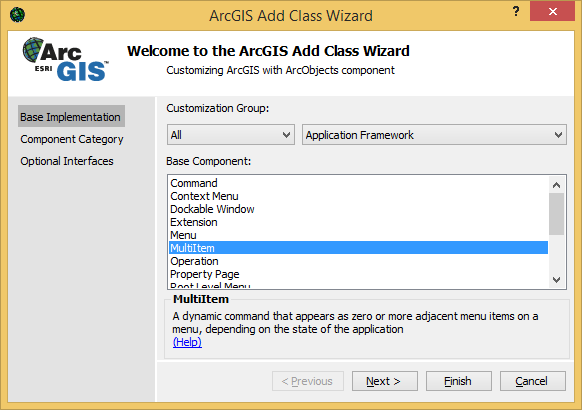
For more information, see Using the ArcGIS Add Class Wizard to extend ArcObjects.
Using any other method
The following table lists add-in types and related SDK documentation to help you create equivalent custom components if you're not using item templates:
Add-in type |
SDK documentation |
Button |
To implement a button, see Creating commands and tools. For example, you can create a class that implements the ICommand interface or inherits from BaseCommand. |
Tool |
|
Combo box |
You can extend ArcObjects by implementing custom combo boxes that inherit from BaseCommand and implement IComboBox. For more information, see Creating combo boxes. |
MultiItem |
How to create dynamic menu commands using a MultiItem shows how to implement IMultiItem. |
Menu |
|
Context menu |
For ArcGIS for Desktop only, the topic How to implement custom toolbars and menus shows how to implement the IMenuDef and IShortcutMenu interfaces. |
Toolbar |
Consider using the ToolbarControl, which provides a toolbar interface as well as a framework to enable the user to customize the application by allowing them to reposition, add, and remove most other user interface components (such as toolbars, commands, and menus). For more information, see Using the ToolbarControl.
To implement a custom toolbar, see How to implement custom toolbars and menus, which shows how to implement the IToolbarDef interface. |
ToolPalette |
You can extend ArcObjects by inheriting from BaseCommand and implementing IToolPalette. For more information, see Creating tool palettes. |
Extension |
Application extensions and Editor extensions can be created by following the same workflow as described in How to create an application extension (that is, by implementing the IExtension interface).
For Editor extensions, the Editor object is passed to your extension via the InitializationData variant in the extension startup method, and you can set a module level variable for the editor in this routine. The extension is then registered to the ESRI Editor Extensions component category (editorextensions).
Application extensions can be registered to other categories, such as the ArcMap application extension (MxExtension). |
DockableWindow |
You can extend ArcObjects by implementing IDockableWindowDef. For more information, see Creating dockable windows. |
AddInComponent |
There is no equivalent to AddInComponent. This add-in type provides a way for add-ins to communicate with other external add-ins or COM components. Your custom component will be able to communicate directly with other COM components. |
AddInDispatchHelper |
There is no equivalent to AddInDispatchHelper. This add-in type provides a way for add-ins to communicate with other external add-ins or COM components. Your custom component will be able to communicate directly with other COM components. |
Your custom component must be registered in a component category. If you created your custom component using an ArcGIS item template, it will be automatically registered in a COM component category. To register your custom component in a COM component category when using something other than an item template, see Registering classes in COM component categories.
Transferring .NET code
After you have created you custom component, transfer any member data and methods from the add-in to the component. Most methods from the add-in's .NET code can be transferred directly to the component. The OnUpdate method is specific to add-ins and replaces the ICommand.OnCreate method and ICommand.Enabled property of a component.
Add-ins include a Config.Designer.cs file, which predefines several static members - for example, add-ins can use ArcMap.Document when IMxDocument is needed. In your component, replace those static members with the actual types as shown in the following table:
Static members |
Type |
ArcMap.Application |
IApplication |
ArcMap.Document |
IMxDocument |
ArcMap.ThisApplication |
IMxApplication |
ArcMap.DockableWindowManager |
IDockableWindowManager |
ArcMap.Events |
IDocumentEvents_Event |
The add-in from which you are converting may contain code to work with a MapControl or a ToolbarControl or to access the map via a hook helper. For custom components, see How to create a command or tool to work with the controls.
Transferring XML
An add-in's Config.esriaddinx XML file looks similar to the following:
[XML] <Name>ArcMapAddin1</Name>
<AddInID>{C042EA1A-C5E0-410c-8F2F-04422EBD78C1}</AddInID>
<Description>Type a description for the add-in.</Description>
<Version>1.0</Version>
<Author>Xiaoling</Author>
<Company>ESRI</Company>
<Date>7.28.2009 2:42:42 PM</Date>
In this XML file, only the image can be coded in your .NET component. You can include the image directly in the project as an embedded resource and set the ICommand.Bitmap property in the component constructor.
Do not transfer the AddInID tag from the XML to your .NET component. A globally unique identifier (GUID) was assigned to your custom component class when it was created with an item template or through registering the class in the applicable COM component category.
Building, testing, debugging, and deploying the custom component
See Create a command by inheriting from BaseCommand to complete your custom component.
See Also:
Using item templates to extend ArcObjectsRegistering classes in COM component categories
Creating toolbars and menus
Creating an application extension
Development licensing | Deployment licensing |
---|---|
Engine Developer Kit | Engine |
ArcGIS for Desktop Basic | ArcGIS for Desktop Basic |
ArcGIS for Desktop Standard | ArcGIS for Desktop Standard |
ArcGIS for Desktop Advanced | ArcGIS for Desktop Advanced |